Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial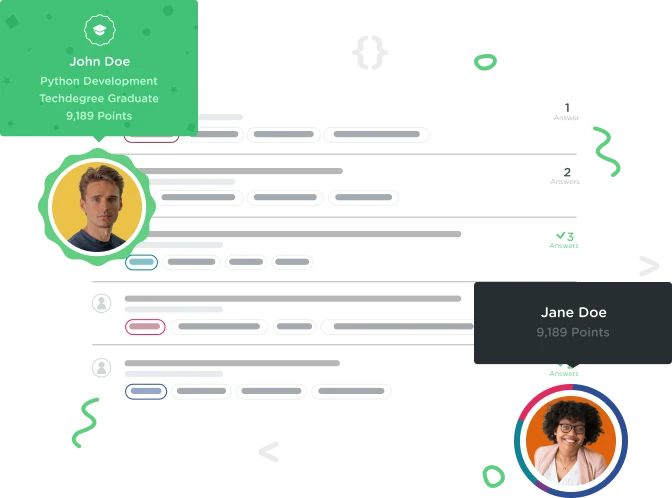
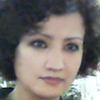
Durdona Abdusamikovna
1,553 PointsI need better explanation on SCOPE
I am slightly lost and don't feel that I fully comprehended it ... Here is a line of codes below
function greetings(){ // function scope
var person = "Lilah";
alert (person);
}
var person ="George"; // this is a scope outside of the function and is called a global scope
greetings();
alert(person);
greetings();
alert(person);
// in these case "Lilah", "George", "Lilah", "George" WHY? - Because all functions can access the
//GLOBAL SCOPE and FUNCTION can change the value of a variable from the GLOBAL SCOPE.
//This is how I understood a script above based on a given lecture
Here is another one. Why this code shows 'Trish' first ?
var name = "Trish";
function setName() {
var name = "Sarah";
}
setName();
alert(name);
Thank you beforehand
14 Answers
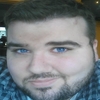
Marcus Parsons
15,719 PointsHey, Durdona aka Dash Dash! :P
Nice to see you again. I'm seeing a lot of confusion here, so I thought I'd try my hand at clearing the air here. You already know how to initialize variables in the global and local scope, so I won't go over that. But, I will over hoisting so that you can get a better feel for how JavaScript treats variables.
Hoisting
Variable Declaration
This is a variable declaration (notice that there is no var keyword):
y = 10;
In a variable declaration, there is no need to use the "var" keyword to initialize the variable, and the variable is always created in the global scope, regardless of where it is declared from. This means that you can use the variable even if you haven't properly initialized it and it is declared within a function. This is what it means to hoist a variable, because even though it's a part of the local scope, it is still hoisted into the global scope:
function myfunc() {
y = 10;
}
myfunc();
alert(y);
Variable Initialization
This is variable initialization (notice now that the var keyword is used both times):
var y = 10;
alert(y);
function myfunc() {
var y = 20;
alert(y);
}
myfunc();
alert(y);
The first alert will show 10, because y is initialized to 10 in the global scope. The second alert, within myfunc(), will show 20 because JavaScript always uses local variables before global ones within functions. Then, the 3rd alert displays 10 again, because after the function executes, the y that was initialized within the function is destroyed, and the only y remaining is the one initialized to begin with var y = 10;
JavaScript always prefers local variables over global ones, even if the initial variable was declared and not initialized.
But let's go back to the declaration and try the above code again, changing what's inside the myfunc() function around a little bit:
var y = 10;
alert(y);
function myfunc() {
y = 20;
alert(y);
}
myfunc();
alert(y);
Notice now that there is no var keyword within myfunc() for the y value. What this means is that we aren't initializing a local variable named y, we are setting the global variable named y to be 20. This means that instead of 10, 20, and 10 showing for alerts, it will now be 10, 20, 20.
Read over this a few times if necessary, and let me know if that helped you some! :)
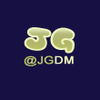
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi there Durdona,
Function scope is a term used to describe the availability of a variable for use in another part of the program. If for instance you declared a variable inside of the function this variable would be local to that function. It has local scope and can only be used inside that function.
If however you had a variable declared outside of any function that variable would be global. Any function can then access and use it!
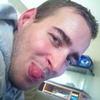
Raymond Osier
16,581 Pointsthe reason that the var name = "trish" shows first because it ia set as a global scope variable witch is avail to all methods in the program .. the var name = "sarah" is a local scope variable witch is only avail to the function setName . the only way to return that variable is to call the function setName() . I hope I helped with that without confusing you more think of scope as global or avail to the entire program and local as avail to a peice of the program

Brian Martin
3,773 PointsMy only question is, in the example it calls setName( ) at the end... why wouldn't it show Trish and then show Sarah as well, since it is being called as setName ( ); right after the function?
var name = "Trish"; function setName() { var name = "Sarah"; } setName(); alert(name);
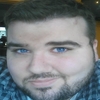
Marcus Parsons
15,719 PointsBrian,
Whenever you set a variable in a function using the var
keyword, that variable is created as a local variable to that function and is destroyed when the function ends. So, the name variable that exists outside of the function is different from the name variable within the function. If you leave off the var keyword, however, when setting the name inside the function, it will change the global variable.
Read my answer for a full explanation :)
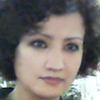
Durdona Abdusamikovna
1,553 PointsI apologize it is still not clear to me ... My confusion probably starts with knowing Java programming language... Here is what I found http://stackoverflow.com/questions/500431/what-is-the-scope-of-variables-in-javascript
I started running given codes. The first example runs empty alert box ... What is it ? Why can't I recognize scope ?
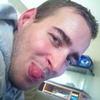
Raymond Osier
16,581 Pointsif you know java the concepts behind variable scope are very similar ...when you create a variable or an object inside a method in one of your classes, it is useable only inside that method . if you go outside of that part of the program you can no longer use the variable. The { and } brackets in a program define the boundaries for a variables scope . any variable created inside thease brackets can not be used outside of them . This same concept applies to JavaScript if you create a variable outside of the { and } brackets it can be used and where in the program if you create a variable inside { and } brackets it can only be used with in that method.
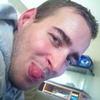
Raymond Osier
16,581 Pointsvar scope ="global";
// this var can be used in any part of the program
function local (){
var scope = "local";
// this var can only be used inside this function
};
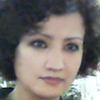
Durdona Abdusamikovna
1,553 PointsRaymond thank you but you keep explaining to me what I already know ... can you pay attention to previous my answer where I put a link from stackoverflow ? Ifound out it is not only about scope but also about hoisting
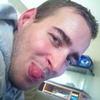
Raymond Osier
16,581 PointsI am sorry I guess I am confused on what portion of Scope you are having trouble with I looked over the link you posted and to me it added more variables to your difficulty insted of narrowing down the root cause of your confuision
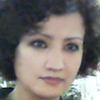
Durdona Abdusamikovna
1,553 PointsI was told Visual Studio has JavaScript Debugger tool maybe after trying it I will comprehend it better. I am trying to install it now. Thank you again
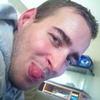
Raymond Osier
16,581 PointsYour welcome Im sorry i couldnt have been more help.

Leyna Cameron
3,792 PointsBasically when you declare a variable within a function, between the two curly braces, it only exists within that function. This is called a local variable. You can only use this variable by calling the function. However, if you declare a variable outside of a function it's considered a global variable so it can be used inside of a function as well as outside of it. If you're using a local variable and a global variable with the same name, you'll have to declare the variable within the function so that it knows that it's local. Otherwise the global function could be pulled into the function and changed.
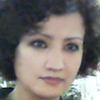
Durdona Abdusamikovna
1,553 PointsWould you mind to run a scrip below and tell me what it is ? Why it is not displaying number 1 in an alert box ?
var a = 1;
// global scope
function one() {
alert(a);
}
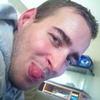
Raymond Osier
16,581 Pointsyou have to call the function like this
one();
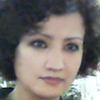
Durdona Abdusamikovna
1,553 Pointsand I have the second alert box with 'undefined' in it ... which refers to a variable in the function (local variable) am I right ?
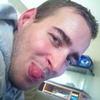
Raymond Osier
16,581 Pointsno im not sure why you have a second alert if your just running the code above the only alert you should have pop up would display the number 1
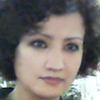
Durdona Abdusamikovna
1,553 Pointsseems like because I call the function with another alert ...
var a = 1;
// global scope
function one() {
alert(a);
}
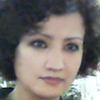
Durdona Abdusamikovna
1,553 PointsRaymond I asked this question on stackoverflow and here is what they say http://stackoverflow.com/questions/29612538/alert-function-in-javascript/29612590#29612590
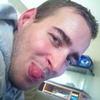
Raymond Osier
16,581 Pointsi see what you did to get the second alert now .. was it your intention to have two alerts with two different values
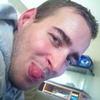
Raymond Osier
16,581 Pointsthis is how i called this code
var a = 1;
function one(){
alert (a);
};
one();
// by not wrapping this function call in an alert() method it calls function one that is already programmed to alert the val of the global variable a i would not wrap this function call in an alert method by doing so is what gave you the second alert that said undefined.
// now if i had wanted to change a global var value to a local var value i would do some thing like this
function two(){
var a = 2;
alert(a);
}
two();
// preview this in the browser and it will display two alert boxes both showing different val for the same variable.
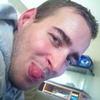
Raymond Osier
16,581 Pointssorry formatting the last post went wrong the back ticks were on the wrong line
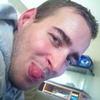
Raymond Osier
16,581 Pointsthis is how i called this code
var a = 1;
function one(){
alert (a);
};
one();
// by not wrapping this function call in an alert() method it calls function one that is already programmed to alert the val of the global variable a i would not wrap this function call in an alert method by doing so is what gave you the second alert that said undefined.
// now if i had wanted to change a global var value to a local var value i would do some thing like this
function two(){
var a = 2;
alert(a);
}
two();
// preview this in the browser and it will display two alert boxes both showing different val for the same variable.
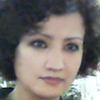
Durdona Abdusamikovna
1,553 Pointswould you like to start the back ticks on a new line it would color your code I use lowercase ```javascript :)
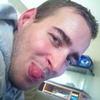
Raymond Osier
16,581 Points var a = 1;
function one(){
alert (a);
};
one();
// by not wrapping this function call in an alert() method it calls function one that is already programmed to alert the val of the global variable a i would not wrap this function call in an alert method by doing so is what gave you the second alert that said undefined.
// now if i had wanted to change a global var value to a local var value i would do some thing like this
function two(){
var a = 2;
alert(a);
}
two();
// preview this in the browser and it will display two alert boxes both showing different val for the same variable.
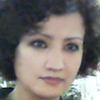
Durdona Abdusamikovna
1,553 PointsI'm using Chrome Debugger Visual Studio needed a lot of RAM space 6GB ... what I see is in you first script: there is no local variable we have only global one which overwrites a variable inside the function the reason of that is function didn't define any variable if it does it should include 'var' like 'var a' otherwise global changes its value.
the second script has a local variable inside the function and shows that value in the alert box
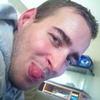
Raymond Osier
16,581 Pointsyes exactly
the first script only defined the alert method and we used the global var a as its argument .
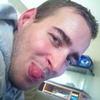
Raymond Osier
16,581 Pointsnow with this same code example i can go a step further and show you a 3rd alert with the original val of var a
var a = 1;
function one(){
alert (a);
};
one();
function two(){
var a = 2;
alert(a);
}
two();
// by using the alert method and passing it the var a as its argument it will display 1 in 3rd alert pop up
alert(a);
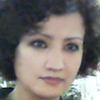
Durdona Abdusamikovna
1,553 PointsAs I see in chrome debugger a program runs meaning starts with function like one(); in this case ...
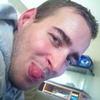
Raymond Osier
16,581 Pointsyes ;)
Durdona Abdusamikovna
1,553 PointsDurdona Abdusamikovna
1,553 PointsIt is nice to see you again ... actually I was hoping to get your response so I was not wrong :) You gave well detailed explanation including hoisting. Now I comprehend it better.
Truly appreciate your taking the time to give well detailed answer :)
By the way now I have full name :) See you around !
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsThank you very much, Durdona, and you're very welcome! :) Anytime you need me you can tag me on here! Do you know how to tag yet on the forums?
Durdona Abdusamikovna
1,553 PointsDurdona Abdusamikovna
1,553 Pointsno never done this before
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsIt's very easy! It's the same as tagging on Twitter/Instagram/Some other social media site I've forgotten to mention! All you have to do is type the @ symbol and then start typing my name. You'll see this ugly mug of mine pop up, and then you just click that name!
Durdona Abdusamikovna :D
Durdona Abdusamikovna
1,553 PointsDurdona Abdusamikovna
1,553 PointsCool :) I will do that . Thank you again
Danielle Bartholomew
1,549 PointsDanielle Bartholomew
1,549 PointsMarcus Parsons, thank you SO MUCH for this clear, detailed explanation over what for me was a foggy issue!