Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial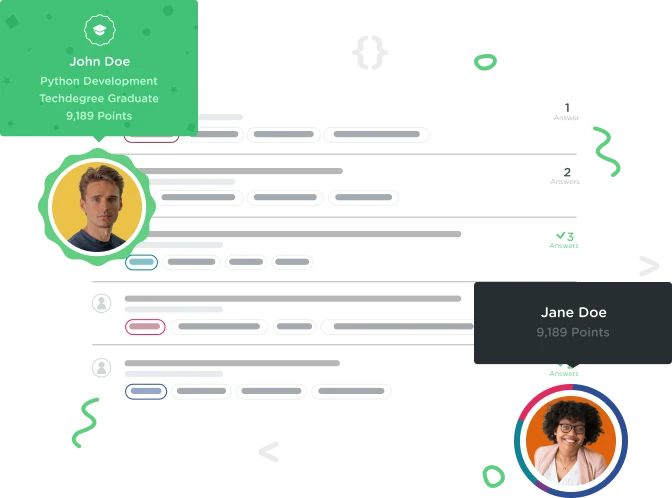
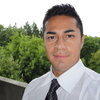
Walter Cortez
4,071 PointsI need help with my code... Thank you.
<?php $studentOneName = 'Dave'; $studentOneGPA = 3.8;
$studentTwoName = 'Treasure'; $studentTwoGPA = 4.0;
//Place your code below this comment if ($studentOneGPA == 4.0) { echo "$studentOneName made the Honor Roll"; } else { echo "$studentOneName has a GPA of GPA"; }
if ($studentTwoGPA == 4.0) { echo "$studentTwoName made the Honor Roll"; } else { echo "$studentTwoName has a GPA of GPA"; }
?>
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA == 4.0) {
echo "$studentOneName made the Honor Roll";
} else {
echo "$studentOneName has a GPA of GPA";
}
if ($studentTwoGPA == 4.0) {
echo "$studentTwoName made the Honor Roll";
} else {
echo "$studentTwoName has a GPA of GPA";
}
?>

Kim Gee
3,841 Pointswrite echo statements like this. Place the variable without "". Then add the + sign to add the 2 together echo $studentOneName + "made the Honor Roll"; echo $studentOneName + "has a GPA of $studentOneGPA";
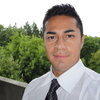
Walter Cortez
4,071 PointsThank you Amr!
1 Answer
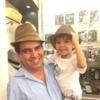
Humberto Sifuentes
7,694 PointsAs I remember, the "." is used as concatenation symbol in PHP:
echo $variable1 . "String" . $variable2 ;
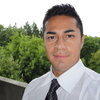
Walter Cortez
4,071 PointsGracias Humberto!
Amr Aly
5,201 PointsAmr Aly
5,201 PointsYou're almost done with this task . All you have to do is : in the echo statement replace the the word GPA with variable $studentOneGPA in the first if statement and the second $studentTwoGPA so you can know what grade he or she has gained if their grade is not equal to 4.0. And it will look like this in the index.php <?php $studentOneName = 'Dave'; $studentOneGPA = 3.8;
$studentTwoName = 'Treasure'; $studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA == 4.0) { echo "$studentOneName made the Honor Roll"; } else { echo "$studentOneName has a GPA of $studentOneGPA"; }
?>