Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial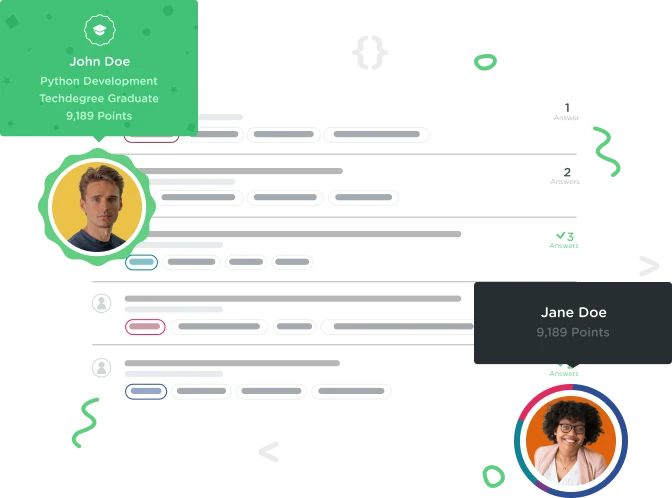

Craig Steward
9,100 PointsI need help with the word_count challenge in python collections
Hi,
I'm receiving the following error for my code - 'Bummer! Hmm, didn't get the expected output. Be sure you're not splitting only on spaces!
My understanding is that as I'm not specifying a separator, the default is to split by spaces. Where I'm lost is what I should actually be entering as the separator in split() ?
If someone could hint or point me in the right direction that'd be great!
Thanks
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
string_1 = "I do not like it Sam I Am"
def word_count(string_1):
list_1 = string_1.split()
dict_1 = {}
count_1 = 0
for key in list_1:
if key in dict_1:
count_1 += 1
dict_1.update({key: count_1})
else:
count_1 = 1
dict_1.update({key: count_1})
return dict_1
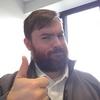
stephenmelendy
3,578 PointsJohn, make sure you are doing string = string.lower()
If you simply put string.lower(), it doesn't carry to future lines. I was getting the "splitting on spaces" error until I properly applied the .lower() method.
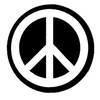
john larson
16,594 PointsHi Steven, yes. Here is how I did .lower()
string = string.lower().split()
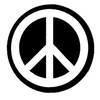
john larson
16,594 PointsSteven, since your code passed...do you feel like sharing it? My code works, I think there's just some quirk I'm missing
def word_count(string):
dict_count = {}
count = 0
string = string.lower().split()
for word in string:
if word in dict_count:
count +=1
else:
count =1
dict_count.update({word: count})
return dict_count
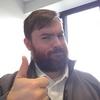
stephenmelendy
3,578 PointsSee my new answer below. This question seems a little funky, hopefully they don't mind me posting my code.
3 Answers
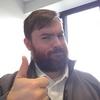
stephenmelendy
3,578 PointsSince this question has weird behavior, here is my code (which worked). Although I don't remember Mr.Love covering the .count() method.
def word_count(string):
string = string.lower()
list_1 = string.split()
dict_1 = dict()
for item in list_1:
dict_1.update({item:list_1.count(item)})
return dict_1
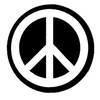
john larson
16,594 PointsThanks Steven, string.count(word) did the trick, and made it a whole lot simpler. I'd still like to know what is wrong with mine the way I did it first. I passed in a different string and the counts were messed up.
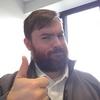
stephenmelendy
3,578 PointsTry making the string lowercase, my answer didn't take until I did:
string_1 = string_1.lower()
edit: simply putting string.lower() will only apply the method for that single line. You need the equals sign for it to apply to the string variable and carry forward.

Craig Steward
9,100 PointsTried that, still returning the same error. I knew I still had to lowercase everything but figured the error returning is specific to spaces so wouldn't be the problem.

Chris Walker
5,004 PointsI really hate these challenges. They aren't challenges to get the same output. They're challenges to do it exactly how they want. I write variation after variation that returns the same output (verified in repl.it) and still get errors.
john larson
16,594 Pointsjohn larson
16,594 PointsI'm getting that error also, local seems to be returning the proper output. Anyone resolve this? I am doing .lower() on the string.