Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial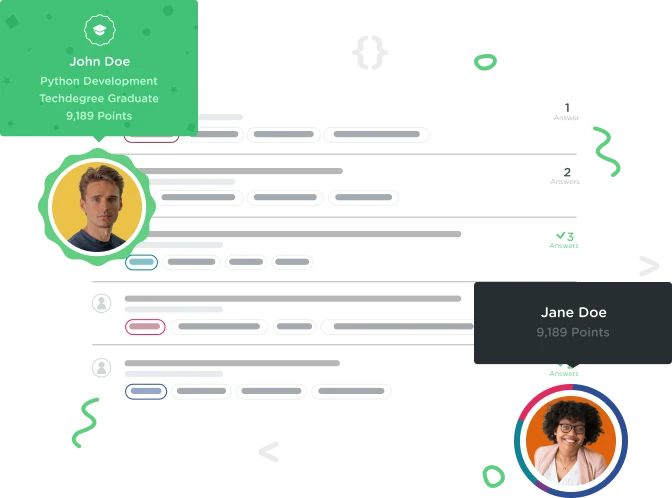

Amanuel Tadesse
6,862 PointsI need help with this Challenge Task. I have checked my answer in the python IDLE and it seems to be correct there.
Alright, this one might be a bit challenging but you've been doing great so far, so I'm sure you can manage it. I need you to make a function named word_count. It should accept a single argument which will be a string. The function needs to return a dictionary. The keys in the dictionary will be each of the words in the string, lowercased. The values will be how many times that particular word appears in the string. Check the comments below for an example.
E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
{'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
Lowercase the string to make it easier.
def word_count(string):
string = string.lower()
word_dictionary = dict()
list_of_words = string.split(" ")
for word in list_of_words:
word_dictionary[word] = list_of_words.count(word)
return word_dictionary
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
string = string.lower()
word_dictionary = dict()
list_of_words = string.split(" ")
for word in list_of_words:
word_dictionary[word] = list_of_words.count(word)
return word_dictionary
2 Answers

andren
28,558 PointsThe challenge wants you to split on all whitespace. Your code splits on spaces, which is a type of whitespace, but there are lots of other characters considered whitespace. Like line breaks, tabs, and other things like that.
The code works on the example input since that only uses spaces, but the example input the challenges shows you is often different from the value they actually pass to the function. They often differ in order to make it harder to cheat the challenge checker by hardcoding your return to the right values.
Conveniently enough the split
method actually splits on all whitespace by default if you don't pass it any arguments, so if you remove your argument to split
like this:
def word_count(string):
string = string.lower()
word_dictionary = dict()
list_of_words = string.split()
for word in list_of_words:
word_dictionary[word] = list_of_words.count(word)
return word_dictionary
Then your code will pass.
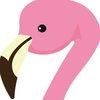
Dave StSomeWhere
19,870 PointsInteresting, your codes does appear to work in the console for the sample of sam I am, but when entered into the challenge it bummers out with something like make sure you lower case everything and split on all white space,
So, try a passing this string and see your results in IDLE - "I not it I Am here is some extra space not am i" and the error should reveal itself.

Amanuel Tadesse
6,862 PointsThanks for the feedback! This was my first question on Treehouse. Unfortunately, I tried that as well but the output seems to be correct again. Still haven't found the bug. Thanks for your suggestion though.
Amanuel Tadesse
6,862 PointsAmanuel Tadesse
6,862 PointsThank you so much! Your answer was clear and informative.
Andrew Kircher
16,612 PointsAndrew Kircher
16,612 PointsThank you! this fixed my code