Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial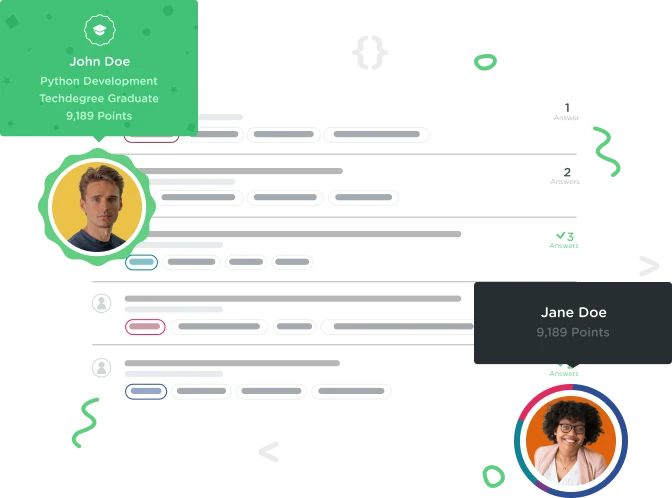
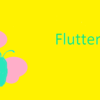
Catherine Kim
Courses Plus Student 7,498 PointsI ran this code in my browser and it works. Why doesn't it answer the challenge question
var secret = prompt("What is the secret password?");
do{
secret = prompt("What is the secret password?");
}
while ( secret !== "sesame" ) {
}
document.write("You know the secret password. Welcome.");
var secret = prompt("What is the secret password?");
do{
}
while ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
}
document.write("You know the secret password. Welcome.");
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
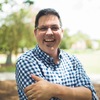
Dave McFarland
Treehouse TeacherYou don't need the second set of braces -- { } -- following the line with the while
in it. The basic structure of a do...while loop is this:
do {
// loop
} while ( condition === true )
Of course, you replace the condition === true
in my example above with the real condition you are testing.
1 Answer
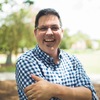
Dave McFarland
Treehouse TeacherHi, Catherine Kim
The basic structure of the do...while loop looks like this:
do {
// the loop code goes in here
} while ( )
Because a do...while loop always runs at least once, you only need the prompt()
method a single time: inside the loop. The prompt will appear once -- if the user provides the secret password then the while condition will be false and the loop exits. But if the answer is wrong, then the loop will run a second time, and the prompt will appear a second time. The prompt will appear again and again until the user types sesame
.
I haven't given you the exact answer just a few hints -- try to see if you can solve this code challenge with just those hints.
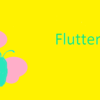
Catherine Kim
Courses Plus Student 7,498 PointsThanks Dave!
Justin Swatloski
5,769 PointsJustin Swatloski
5,769 PointsThe issue here is that you are prompting the user for an input, and once they put it in, the do loop does nothing, and will then continue to do nothing while the variable secret doesn't equal "sesame". Once it DOES equal sesame, you will be prompted for the secret again.
What your code should look like:
You first define the variable outside of the loop so that the do loop can use it for storage, then you execute the Do loop, which in this case will prompt the user for an input, and so long as that input does not equal "sesame", the do loop will keep repeating. Once it does equal sesame, it will go to the next line and write it.