Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial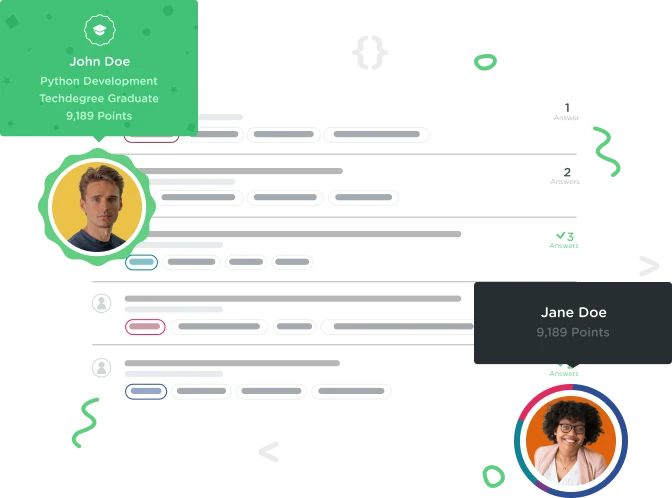
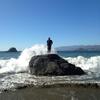
Joe Williams
4,014 PointsI realize there's multiple ways to program the same thing, but would this be considered acceptable? If not, why?
In this assignment, we were supposed to create a 5 question multiple choice quiz that at the end informs the user if they won a gold crown (5 answers correct), silver crown (3-4 answers correct), or bronze crown (1-2 answers correct).
The way Dave did it in the video is not at all how I ended up doing mine. I didn't think to set var correctAnswer = 0, and as the person answer, increase it by +1 if the answers was indeed correct.
I was simply proud that the code I wrote even worked, even after testing it for correct answers 1-5. Is what I created an acceptable program, or did I miss the boat?
If it isn't acceptable, why? What should I keep in mind for the future?
((( I purposely used alert() instead of document.write(). )))
console.log("begin")
alert ("QUIZ TIME! How good are you at simple elementary math? Time to find out!")
guessOne = prompt("Question 1: What is 2+2?");
if (parseInt(guessOne) === 4) {
var answerOne = 1;
} else {
var answerOne = 0;
};
guessTwo = prompt("Question 2: What is 3 x 2?");
if (parseInt(guessTwo) === 6) {
var answerTwo = 1;
} else {
var answerTwo = 0;
};
guessThree = prompt("Question 3: What is 4 + 4?");
if (parseInt(guessThree) === 8) {
var answerThree = 1;
} else {
var answerThree = 0;
};
guessFour = prompt("Question 4: What is 5 + 5?");
if (parseInt(guessFour) === 10) {
var answerFour = 1;
} else {
var answerFour = 0;
};
guessFive = prompt("Question 5: What is 1 + 1?");
if (parseInt(guessFive) === 2) {
var answerFive = 1;
} else {
var answerFive = 0;
};
alert("Time to tally the results and see if you're a winner!");
var finalAnswer = parseInt(answerOne) + parseInt(answerTwo) + parseInt(answerThree) +
parseInt(answerFour) + parseInt(answerFive);
if (parseInt(finalAnswer) === 5) {
alert("Congratulations! You got all 5 correct! You are awarded the gold crown")
} else if (parseInt(finalAnswer) === 3 || parseInt(finalAnswer) === 4 ) {
alert("Congratulations! You got " + finalAnswer + " correct. You are awarded the silver crown")
} else if (parseInt(finalAnswer) === 1 || parseInt(finalAnswer) === 2 ) {
alert("Congratulations! You got " + finalAnswer + " correct. You are awarded the bronze crown")
} else {alert("Unfortauntely you got " + finalAnswer + " correct. You receive no crown and are terrible at math.")}
console.log("end");
1 Answer
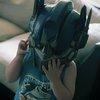
Colby Work
20,422 PointsI would say that your code is fine, but it could be refined and made more efficient. It's good that you are asking this, because the more you look at your code, the more you will find ways to simplify it. And you are right, there are lots of ways to write the same program, so you are definitely on the right track.
the reason for the counter variable at the beginning is for the sake of efficiency. By keeping track of the score as you go along, you don't need any calculations at the end, and also simplify the if/else, into a single if statement. (If the answer is right, add 1 to the score)
also, you have a lot of unnecessary parseInts...
when you are adding up the variables for finalAnswer, you do not need to use parseInt because they are already integers. You only need to use parseInt on the prompt, since they are coming in as strings. Your if/else statements at the end don't need parseInt either, because it's already an integer.
overall, I'd say it's a great start, and if it works that's great! Just keep learning the ins and outs, best practices, etc...and you'll do fine. I'm still learning myself! Hope that helps :)
haunguyen
14,985 Pointshaunguyen
14,985 PointsI have not watch the video or I forgot about it. But I don't think this code is helpful to your personal development.
If you have learned about objects, my approach would be to create an array that contains objects (say 5 objects). Each object has key:value pairs {"question":"what is this?", "answer":"good question"}.
Use a "for" loop or "while/do while" loop or practice with all of them, and loop through the array. Use the variable "total" and increase it by 1 if the prompt input matches the "answer's" value.
In your if statements, use the ( n greater than min && n less than max ) to range the conditions. For practice, you can also use "switch" to replace the if / else if block.