Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial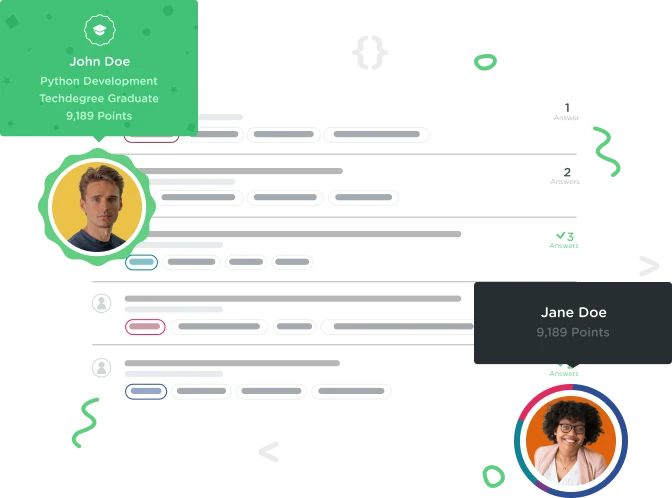

Emiliyan Tsanov
Courses Plus Student 562 PointsI receive the following error: "Bummer! You need to check that $studentOneGPA is equal to 4.0". My code seems okay.
I wrote two separate codes - one using foreach (just for the fun), and another doing exactly what you wanted. "Check work" does not approve my code. What is the reason?
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if($studentOneGPA == '4.0')
echo $studentOneName.' '.'made the Honor Roll';
else
echo $studentOneName.' has a GPA of '.$studentOneGPA;
if($studentTwoGPA == '4.0')
echo $studentTwoName.' '.'made the Honor Roll';
else
echo $studentTwoName.' has a GPA of '.$studentTwoGPA;
//$students = array(
//array(
//'name' => $studentOneName,
//'gpa' => $studentOneGPA),
//array(
// 'name' => $studentTwoName,
// 'gpa' => $studentTwoGPA));
//foreach($students as $student) {
// if($student['gpa'] == '4.0')
// echo $student['name'].' '.'made the Honor Roll'."<br>";
// else
// echo $student['name'].' '.'has a GPA of'.$student['gpa']."<br>";
//}
?>
3 Answers

Bogdan Cabaj
16,349 PointsYou are missing brackets and you had '4.0' instead of 4.0.
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if($studentOneGPA == 4.0) {
echo $studentOneName.' '.'made the Honor Roll';
}else{
echo $studentOneName.' has a GPA of '.$studentOneGPA;
}
if($studentTwoGPA == 4.0) {
echo $studentTwoName.' '.'made the Honor Roll';
}else{
echo $studentTwoName.' has a GPA of '.$studentTwoGPA;
}
?>
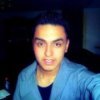
Abdul Zainos
4,938 PointsIt may want you to call out the GPA as an integer instead of a string. I remember having issues with this as well. When in doubt check out the teacher's notes and refer to the PHP.NET site they provide.
<?PHP
if ($studentOneGPA == 4.0) {
echo "$studentOneName made the Honor Roll";
} else {
echo "$studentOneName has a GPA of $studentOneGPA";
}
if ($studentTwoGPA == 4.0) {
echo "$studentTwoName made the Honor Roll";
} else {
echo "$studentTwoName has a GPA of $studentTwoGPA";
}
?>

Emiliyan Tsanov
Courses Plus Student 562 PointsThat's not it, still getting a Bummer...

Christof Beck
5,185 PointsI had the same problem. I think the solution is to add the { } brackets. It isn't actually needed here since you only have 1 statement, the echo statement. I think it's a bug in the challenge and I reported it.