Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial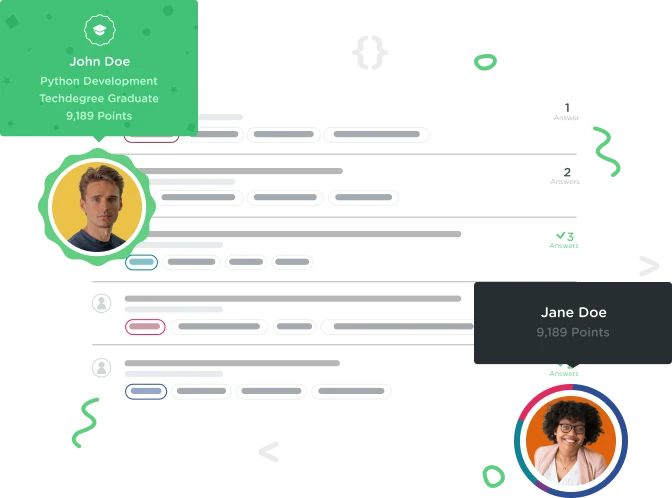

lachlanratjens2
6,759 PointsI seem to have an intermittent error in part one and when part three does run it doesnt pass the test but cant see why.
Any help would be much appricated.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(dict_1):
loop_count, max_count, teacher_max = 0, 0, ''
for teacher in dict_1:
loop_count = 0
for classes in dict_1[teacher]:
loop_count = loop_count + 1
max_count,teacher_max = loop_count, teacher if max_count < loop_count else teacher_max
return teacher_max
def num_teachers(dict_1):
teachers_count = 0
for teacher in dict_1:
teachers_count = teachers_count + 1
return teachers_count
def stats(dict_1):
list_1 = list()
counter = 0
for teacher in dict_1:
counter = 0
for classes in dict_1[teacher]:
counter = counter + 1
list_1.append((teacher,counter))
return list_1
1 Answer

Unsubscribed User
3,273 PointsIt took me a second but I found the problem with your section 3 code. Here is your code:
def stats(dict_1):
list_1 = list()
counter = 0
for teacher in dict_1:
counter = 0
for classes in dict_1[teacher]:
counter = counter + 1
list_1.append((teacher,counter))
return list_1
Here are the changes I made to get it working: 1) removed the counter variable that is outside of the for loop (it doesn't do anything and is just taking up space).
2) moved the list_1.append((teacher, counter)) so that it is outside of second for loop but inside the first one. This was causing your problem to fail since it was appending the teacher and counter to the list each time you itterated through it meaning a teacher with multiple classes would have multiple entries in the list. By moving it so that it is only in the first for loop we only append each teacher to the list once with their total class count.
3) changed counter = counter +1 to counter += 1. These perform the same function but the later is shorter and easier to look at. Remember that programmers are lazy and will do almost anything thing to save a few keystrokes : )
Here is what that final count looks like:
def stats(dict_1):
list_1 = list()
for teacher in dict_1:
counter = 0
for classes in dict_1[teacher]:
counter += 1
list_1.append((teacher, counter))
return list_1

Unsubscribed User
3,273 PointsIf you're looking to make your code a bit cleaner I've gone though the first three steps of the challenge and written a slightly more concise version. Here it is if you are interested:
def most_classes(teachers):
max_count = 0
best_teacher = ""
for teacher in teachers:
if len(teachers[teacher]) > max_count:
max_count = len(teachers[teacher])
best_teacher = teacher
return best_teacher
def num_teachers(teachers):
return len(teachers)
def stats(teachers):
teacher_list = []
for teacher in teachers.keys():
teacher_list.append([teacher, len(teachers[teacher])])
return teacher_list

lachlanratjens2
6,759 PointsThank you so much for your help. I see where you are coming from about the more concise version. I like how you got rid of my nested for loops. More concise and probably runs a whole lot faster.
Christian Andersson
8,712 PointsChristian Andersson
8,712 PointsSorry for posting this off-topic, but I'm helping Treehouse investigate a potential issue with their webserver. Please follow this link and leave a comment saying if you have experienced this webserver issue or not.
https://teamtreehouse.com/forum/attention-treehouse-webserver-issues-please-read
Thank you.