Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial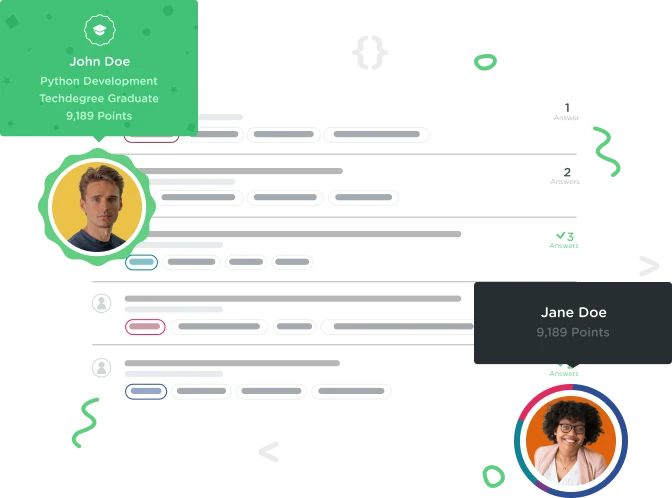

Daniel Abbott
16,780 PointsI seriously don't understand 2 things. 1) what is the point of the IF statement and 2) what am I doing wrong?
def word_count(string):
words = string.lower()
word_dict = {}
for word in words.split():
if word in word_dict[word]:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict[word]
there's my code
2 Answers

Chris Shaw
26,676 PointsHi Daniel,
The
IF
statement is required so we can determine whether the keyword
exists in the dictionary already, if it doesn't we refer to theelse
statement instead of set the key with a value of 1 by default, if that key does exist then we simply increment the value by 1 using the+=
operator which is a shortcut in Python and many other languages.Your
return
statement is incorrect, you want to be returning justword_dict
but instead you haveword_dict[word]
which will just return a single value and not the entire dictionary.
def word_count(string):
words = string.lower()
word_dict = {}
for word in words.split():
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict

Jason Anello
Courses Plus Student 94,610 PointsHi Daniel,
The purpose of the if
condition is to find out if that particular word has already been added as a key in the dictionary.
As mentioned in the comments, it should be if word in dict
Your condition should be if word in word_dict
If the key already exists in the dictionary then you want to increment the value at that key, otherwise you would add that key into the dictionary and set the count at 1. That part you're doing correctly.
Lastly, you want to return the dictionary. You're trying to return the count at a particular key.