Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial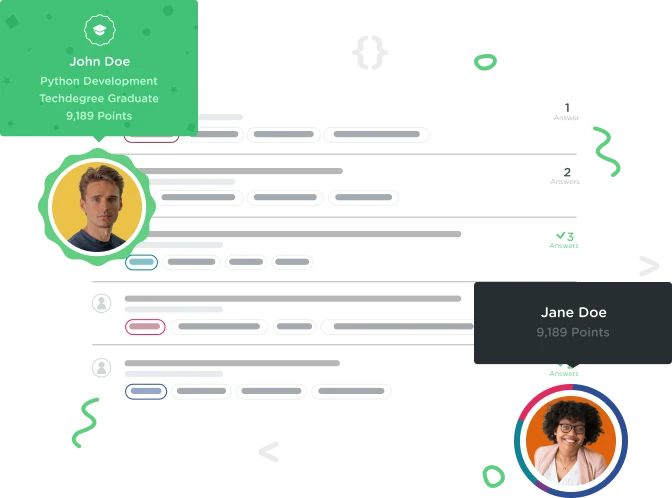
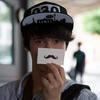
charlesmonaghan
742 PointsI simply don't understand unpacking and packing **kwargs.
I've been trying to solve this for over 2 hours. I've just given up on this question for the day. It'd be awesome to have a clear explanation on what I'm doing wrong.
At the point where it asks "Write a function named string_factory that accepts a list of dictionaries as an argument", what list of dictionaries does it want me to enter?
Thanks!
# Example:
# values = [{"name": "Michelangelo", "food": "PIZZA"}, {"name": "Garfield", "food": "lasagna"}]
# string_factory(values)
# ["Hi, I'm Michelangelo and I love to eat PIZZA!", "Hi, I'm Garfield and I love to eat lasagna!"]
template = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(name=None, food=None):
for name and food:
return("Hi, I'm {name} and I love to eat {food}!".format(name, food))
string_factory(**{"name": "Charles", "food": "Sushi"})
2 Answers

Juan Hurtado
2,243 PointsYou're doing a few things wrong
- The two arguments should be one. Also, NOT in key, value format like how you have it. The instruction says "A list of dictionaries". Meaning one singular list as your argument
- For loop should iterate through the singular list of dictionaries. Meaning ONE list with many dictionaries.
- For each dictionary in dictionaries, unpack the singular dictionary inside of the .format using the ** operator
- Do not call your function at the end. Simply define it. Teamtreehouse is going to call your function to test it.
I suggest you give it a try to solve it using the tips I gave above. If you're still stuck, look at my code below. I suggest reading this which a great article that illustrates in simple terms how to use *args **kwargs.
My code:
def string_factory(dictionaries):
new_list = []
template = "Hi, I'm {name} and I love to eat {food}!"
for dictionary in dictionaries:
new_list.append(template.format(**dictionary))
return new_list
One liner:
def string_factory(dictionaries):
template = "Hi, I'm {name} and I love to eat {food}!"
return [template.format(**dict) for dict in dictionaries]
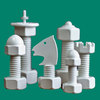
Steven Parker
241,970 PointsWhen the challenge asks you to create a function "that accepts a list of dictionaries as an argument", it's not expecting you to create the list or call the function yourself. You only need to write the function that will work with such a list, and when you submit it, the challenge will call your function and provide the list for it to work on.
The function shown here takes two arguments named "name" and "food", but it should take a list of dictionaries instead (which will be a single argument). The actual name of the list can be anything you like.
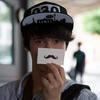
charlesmonaghan
742 PointsCould you please give me a step by step example? I'm still struggling to understand. :)
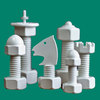
Steven Parker
241,970 PointsLooks like Juan beat me to it. I generally don't leave code spoilers, but I would have given you the same hints.

Juan Hurtado
2,243 PointsI only left the code because after a certain amount of time, sometimes it's just best to see what exactly is it that one is doing wrong. I always encourage solving the answer by yourself, which is why I told him to try to solve it, with the tips I gave him.
But if you really don't get the answer and you have been at it for hours, there is no shame looking for the answer and then the explanation to understand why the answer worked and what you did wrong.