Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial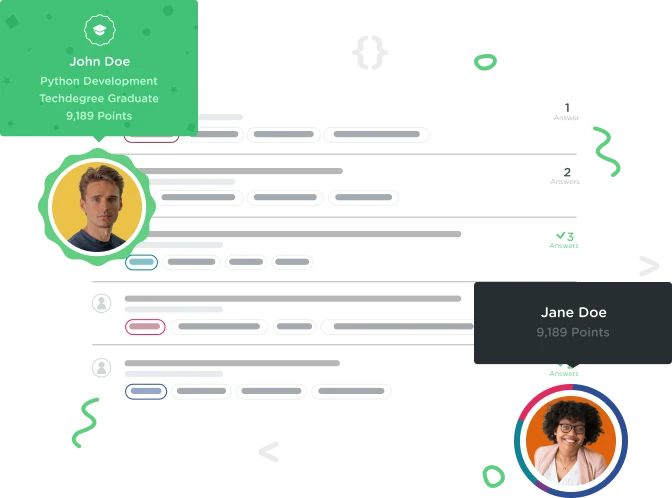
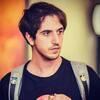
Jonathan Leon
18,813 PointsI succeeded but I think my code is long could anyone take a look and give me tips how to shorten it using booleans?
//settings
var score = 0;
var correct_answer1 = ("KUKI");
var correct_answer2 = ("ANNA");
var correct_answer3 = ("JONATHAN");
var correct_answer4 = ("23");
var correct_answer5 = ("LEON");
//questions
var answer1 = (prompt ("What is the name of my dog?")).toUpperCase();
if (answer1 === correct_answer1) {
(score += 1) + alert ("Correct! you answered " + score + " questions correctly!");
} else {
alert ("Thats wrong! you answered " + score + " questions correctly.");
}
var answer2 = (prompt ("What is the name of my girlfriend?")).toUpperCase();
if (answer2 === correct_answer2) {
(score += 1) + alert ("Correct! you answered " + score + " questions correctly!");
} else {
alert ("Thats wrong! you answered " + score + " questions correctly.");
}
var answer3 = (prompt ("What is my name?")).toUpperCase();
if (answer3 === correct_answer3) {
(score += 1) + alert ("Correct! you answered " + score + " questions correctly!");
} else {
alert ("Thats wrong! you answered " + score + " questions correctly.");
}
var answer4 = (prompt ("What is my age?")).toUpperCase();
if (answer4 === correct_answer4) {
(score += 1) + alert ("Correct! you answered " + score + " questions correctly!");
} else {
alert ("Thats wrong! you answered " + score + " questions correctly.");
}
var answer5 = (prompt ("What is my family name?")).toUpperCase();
if (answer5 === correct_answer5) {
(score += 1) + alert ("Correct! you answered " + score + " questions correctly!");
} else {
alert ("Thats wrong! you answered " + score + " questions correctly.");
}
if (score === 5) {
alert ("Congratulations, you earned a Golden Crown!!!");
} else if (score === 4 || score === 3) {
alert ("Congratulations, you earned a Silver Crown!!!");
} else if (score === 2 || score === 1) {
alert ("Congratulations, you earned a Bronze Crown!!!");
}
else {
alert ("Sadly, you don't know me enough, leave my script at once!!!!");
}
//CONSOLE TEST
console.log (answer1);
console.log (answer2);
console.log (answer3);
console.log (answer4);
console.log (answer5);
console.log (correct_answer1);
//QUIZ
console.log (score);
2 Answers

Chris Bennett
7,499 PointsProgrammers are lazy. We have a saying "Don't Rpeat Yourself" aka DRY.
If you find yourself repeating code try to think about how you can simplify it by making a function or object.
Basically, I put your prompts and alerts into a function call and your questions/answers into an array.
Another thing in JavaScript is that you don't want to put variables into the global namespace, so try to contain your code in a function call if possible.
Hopefully the code with the comments makes sense if not let me know and I'll try to help ya out.
//create a main app function to hide variables from the global namespace.
var runApp = function(){
//set score in the main functions namespace so all the other functions have access to it.
var score = 0;
//Create a list of questions and answers as key/value pairs that we can iterate (loop) through
var questions_and_answers = [
{question: "What is the name of my dog?", answer: "KUKI"},
{question: "What is the name of my girlfriend?",answer: "ANNA"},
{question: "What is my name?", answer: "JONATHAN"},
{question: "What is my age?", answer: "23"},
{question: "What is my family name?", answer: "LEON"}
];
//Function that prompts a qustion checks the answer against the correct answer.
var questionPrompt = function(prompt_text,correct_answer){
answer = prompt(prompt_text).toUpperCase()
if (answer === correct_answer) {
score += 1;
alert("Correct! you answered " + score + " questions correctly!");
} else {
alert("Thats wrong! you answered " + score + " questions correctly.");
}
}
//Checks the value of score to arrive at an alert.
var checkScore = function(){
if (score === 5) {
alert("Congratulations, you earned a Golden Crown!!!");
} else if (score === 4 || score === 3) {
alert("Congratulations, you earned a Silver Crown!!!");
} else if (score === 2 || score === 1) {
alert("Congratulations, you earned a Bronze Crown!!!");
} else {
alert("Sadly, you don't know me enough, leave my script at once!!!!");
}
}
//loops through the list of questions and answers.
//calls the qustionPrompt function for each item in the list of questions_and_answers
for (var i =0; i<questions_and_answers.length; i++){
questionPrompt(questions_and_answers[i]["question"],questions_and_answers[i]["answer"]);
}
//call check score after we have looped through the list to get an alert.
checkScore();
}
//call the app to run
runApp();
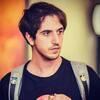
Jonathan Leon
18,813 PointsThanks alot! as I'm learning more now I know how to call functions and I'll look back into this code and shorten it with functions and compare it to yours, much obliged!