Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial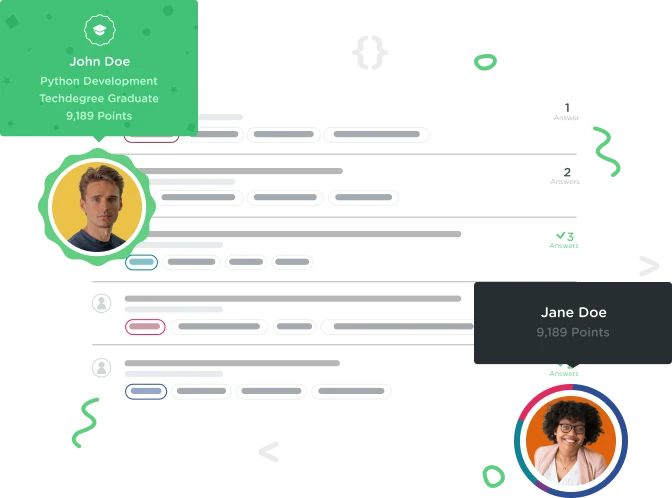

Jason Chiu
4,373 PointsI think my solution is actually better
Here is my solution:
var quizSet = [
['What is your most favorite color?', 'black'],
['What is your American dream?', 'billionaire'],
['What will be your next goal?', 'find a job']
];
function print(message) {
document.write(message);
}
var correctGuess = 0;
function getQuiz (quiz) {
for (var i = 0; i < quiz.length; i++) {
var color = prompt(quiz[i][0]);
if (color === quiz[i][1]) {
correctGuess++;
}
}
}
getQuiz(quizSet);
document.write('<h1>You got ' + correctGuess + ' questions right</h1>');
The program runs properly. Isn't my solution actually more simple than the one that Dave provided? I saw in his solution that he declare many global variables and did not have a function for the loop, so the codes become more complex and redundant.
Any thoughts or suggestions?
3 Answers

Ryan Duchene
Courses Plus Student 46,022 PointsIn the long-term, your code probably is a bit better, as it's better scoped (as you said). Dave's is a intentionally more beginner-friendly, however; it cuts to the chase and has less syntactical sugar.

Drew Teter
Courses Plus Student 1,004 PointsOne thing I'd recommend is making a question/answer pair into an object. So your quizSet would look like this:
var quizSet = [
{'question': 'What is your most favorite color?', 'answer': 'black'},
{'question': 'What is your American dream?', 'answer': 'billionaire'},
{'question': 'What will be your next goal?', 'answer': 'find a job'}
];
This aids readability which is worth the extra resources taken up by the object.
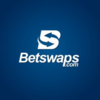
Michael Timbs
15,761 PointsAre you declaring a variable inside a loop here? I thought that was bad programming practice as it is inefficient to keep re-declaring variables inside a loop.
Perhaps try:
function getQuiz (quiz) {
var color;
for (var i = 0; i < quiz.length; i++) {
color = prompt(quiz[i][0]);
if (color === quiz[i][1]) {
correctGuess++;
}
}
}
Also i like to name my variables in way that makes them easy to understand. I recently did a large collaboration project with overseas programmers and it is much easier to read the code to figure out what is going on than to bridge language barriers a lot of the time. here it is obvious what 'color' does but if you are reading thousands of lines of code with hundreds of variables then it helps to name them something as precise as possible.

Drew Teter
Courses Plus Student 1,004 PointsJavascript pulls out the variable declaration from the loop itself. But yes, it is best practice to do it that way.

Ryan Duchene
Courses Plus Student 46,022 PointsFYI, I tweaked your code to add syntax highlighting and added closing curly braces. :)
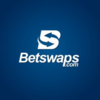
Michael Timbs
15,761 PointsAh I did not know that Drew. I've only been learning JavaScript for a few days. That's handy to know.
Cheers Ryan. I should give the style guide a read for posting comments :)
Dave McFarland
Treehouse TeacherDave McFarland
Treehouse TeacherYeah, what Ryan Duchene says! :)