Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial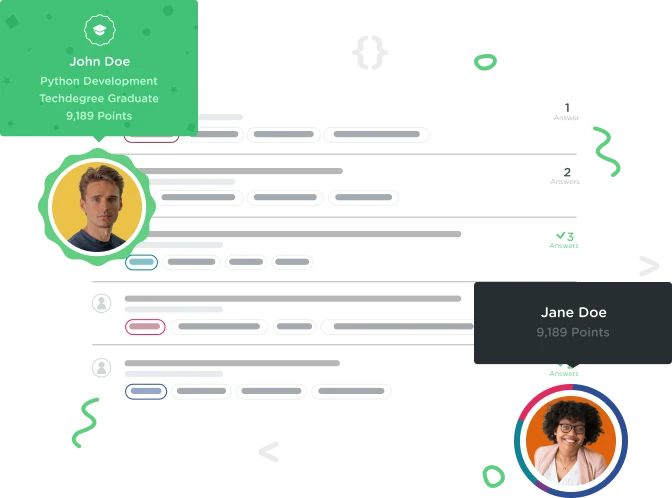
jackmartin3
5,559 PointsI used a while loop for this, Dave used a for loop, am i wrong even though it worked fine?
As the title says, my first thoughts when doing this challenge was a while loop. It worked perfectly fine but Dave used a for loop. Now i'm sitting wondering if i'm thinking about the problem wrong or if i just do things differently? Ultimately, does it matter what type of loop is used? If it gets the job done, its fine right?
My solution :
var html = '';
var red;
var green;
var blue;
var rgbColor;
var colorCounter = 0;
while (colorCounter < 10) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
colorCounter += 1;
}
document.write(html);
Daves solution:
var html = '';
var red;
var green;
var blue;
var rgbColor;
for ( var i = 1; i <= 10; i += 1 ) {
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(html);
2 Answers
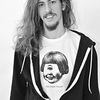
eck
43,038 PointsIt is a matter of preference whether you use a for loop or while loop in this situation. While learning, it is probably a good idea to use both to be familiar with the syntax.
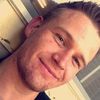
Stephen Bolton
Front End Web Development Techdegree Student 7,933 PointsIn programming you do a lot of loops. I have always found that a for loop and while loop are the same. For loops are a little quicker and lazier than while. If you feel comfortable using while loops then use them. Eventually you might see that for loops are very convenient!
ztwbzekpvk
12,893 Pointsztwbzekpvk
12,893 PointsThey both seem to do the same job, but then again, you wouldn't use a mallet to hammer a screw or a wrench to hammer a nail.