Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial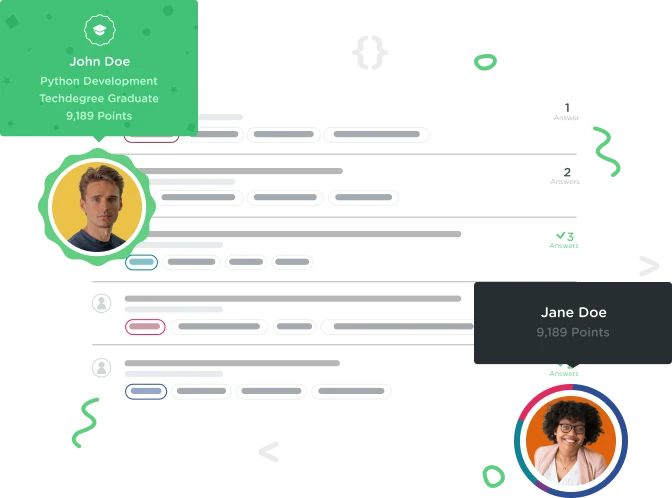

Meet Jethwa
Courses Plus Student 234 Pointsi want a code for when you tap the screen the background changes please help if not the code just tell me give me
touchtocchange
2 Answers

Meet Jethwa
Courses Plus Student 234 Pointsthank you so much Peter . but i dont want a button just tap on the screen and the color should change
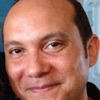
Peter Corletto
2,682 PointsI have never done it, but I checked in stackOverFlow.com and they recommend doing something like this:
yourRelativeLayout.setOnTouchListener(new View.OnTouchListener() {
@Override
public boolean onTouch(View arg0, MotionEvent arg1) {
// Place the change color code inside here.....
return true;//always return true to consume event
}
});
This should make the whole relativeLayout responsive to touch, without using a button.
Also, you have to set your RelativeLayout view this attribute to true: android:clickable="true" (In the XML file).
Peter Corletto
2,682 PointsPeter Corletto
2,682 PointsHello Meet Jethwa,
First, create a new Blank Activity, like "ColorChange" activity. Then, define your xml layout file for your MainActivity, or activity_main.xml, as follows:
RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="wrap_content" android:layout_height="wrap_content" tools:context=".MainActivity" android:id="@+id/relativeLayout">
</RelativeLayout
Then, in MainActivity.java file, put the following code. The user will need to enter the color name, using an EditText, and then tap on the Button. It will then change the background color to that color. Please let me know if this helped. Thank you. Peter. Note: In the above activity_main.xml, do not forget to close the RelativeLayout tag at the end. I do not know why my posted code was cut off here.
package com.example.android.colorchange;
import android.content.Context; import android.graphics.Color; import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.text.TextUtils; import android.view.MotionEvent; import android.view.View; import android.view.inputmethod.InputMethodManager; import android.widget.Button; import android.widget.EditText; import android.widget.RelativeLayout;
public class MainActivity extends ActionBarActivity {
}