Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial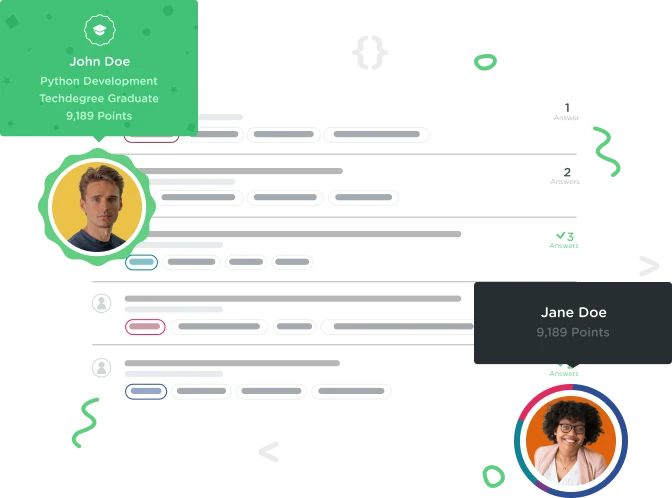

Khurram Shahzad
3,695 PointsI want to send an array of single object by clicking on the item of list from one fragment to another fragment but how ?
write now I am toasting it.. but i want to send the data from one fragment to another fragment and then update the UI there.. how can I do that?
ArrayAdapter<String> adapter=new ArrayAdapter<String>(getActivity(),android.R.layout.simple_list_item_1, dp.getDaysOfMonth()); mListView.setAdapter(adapter);
mListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
String thisDate=mDailyPrayer[position].getDate();
String SunRise=mDailyPrayer[position].getSunRise();
String Fajr=mDailyPrayer[position].getFajrBegins();
String message=String.format("%s ------- %s ------ %s", thisDate,SunRise,Fajr);
Toast.makeText(getActivity(),message,Toast.LENGTH_LONG).show();
}
});
2 Answers

Elikem Kuivi
12,024 PointsThanks for the feedback :). The reason why it is returning null when you rotate is because of the releaseRefList() method call in the onStop() method. When you rotate the screen, the activity/fragment gets destroyed and then recreated. So the onStop() method is called as a result, and in there the releaseRefList() method is called also to remove the object from the ReferenceMap. The ReferenceMap is supposed to work like how you pass Strings/primitive objects between activities; so if you want to keep the object even after it is rotated, the best solution will be to save the variables you need in your onSaveInstance() method. This guide does a good job of explaining how to do this (http://code.hootsuite.com/orientation-changes-on-android/). However, if that won't work well for you, then the best thing to do is to make the object Parcelable instead. Hope this helps :)

Elikem Kuivi
12,024 PointsThere's two ways you could do this, depending how you want to use the items in the mDailyPrayer object in the next fragment:
The recommended approach is to make the associated activity handle communication between both Fragments. So you will need to create an interface in your first fragment (Fragment A). Then in your Activity you will have to implement that interface to pass the data in mDailyPrayer to your second fragment. Please take a look at this tutorial link to find out how to do that [https://developer.android.com/training/basics/fragments/communicating.html]. Also, this link on codepath will be helpful as well [https://guides.codepath.com/android/Creating-and-Using-Fragments#communicating-with-fragments]
I created a small utility class that provides a simple alternative to the first option [https://gist.github.com/kkuivi/161f254374a4007ae6014f67ae45789e]. It acts pretty much the same way as passing data between Intents. All you will need to do is give a key to the data you wish to send, and provide the name of the Fragment you want to send it to.
In Fragment A (Send Object to Fragment B):
ReferenceMap.getInstance().putObjectReference(FragmentB.class, "myKey", mDailyPrayer[position])
In Fragment B (Receive Object to Fragment A):
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
...
ReferenceMap.ReferenceList refList = ReferenceMap.getInstance().getReferenceList(this);
if(refList != null) {
DailyPrayer prayer = (DailyPrayer) refList.get("myKey");
}
....
}
@Override
public void onStop(){
ReferenceMap.getInstance().releaseRefList(this);
super.onStop();
}

Khurram Shahzad
3,695 PointsThanks Sir, I used ReferenceMap to resolve my problem and it worked perfectly.. but I am having one issue, that is.. On rotating screen of Fragment B, it says DailyPrayer object is null.