Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial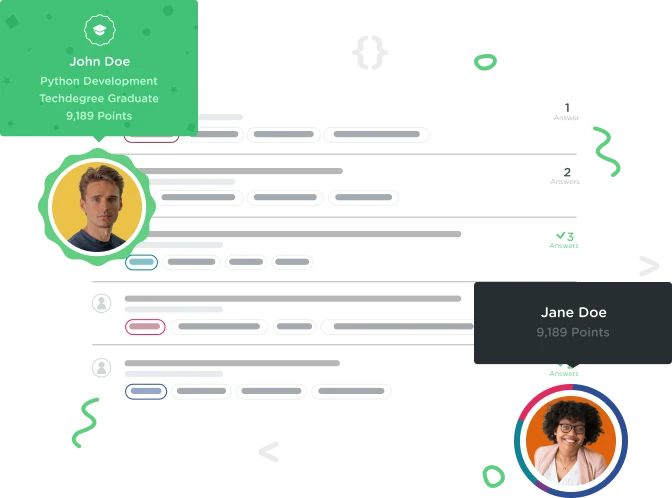

Ismail Shabbir
359 PointsI was wondering why it wont change like this when the variables are already defined?
<?php
//add 5 to integer one and substract 1 from integer 2
$integerOne = 1;
$integerTwo = 2;
$integerOne + 5;
$integerTwo - 1;
?>
```index.php
<?php
//Place your code below this comment
$integerOne = 1;
$integerTwo = 2;
$integerOne + 5;
$integerTwo - 1;
?>
1 Answer
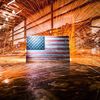
Andrew Shook
31,709 PointsThe problem is you are not actually changing the variables. When you use the variable you are using the value stored in the variable and not changing the value that the variable holds. If you want to change a variable then you need to reset it's value using the equal sign.
<?php
$integerOne = 1;
$integerTwo = 2;
// These two statements are equivalent and the simple add 1 plus 2.
// However, they don't store the value of 1 plus 2 anywhere so after
// the statement execute the value 3 is just dumped from memory.
$integerOne + $integerTwo;
1 + 2;
// In order to save the value of the statements you need to put them in
// a variable.
$newVariable = $integerOne + $integerTwo;
// now $newVariable is equal to 3.
// In order to change the value of an existing variable you need to use the equal sign
$integerOne = $integerOne + $integerTwo;
// now the value of $integerOne is 3, because 2 plus 1 is three.
// Also, you can rewrite the above statement like so.
$integerOne += $integerTwo;
//this is just a shorthand way of writing $integerOne = $integerOne + $integerTwo;