Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial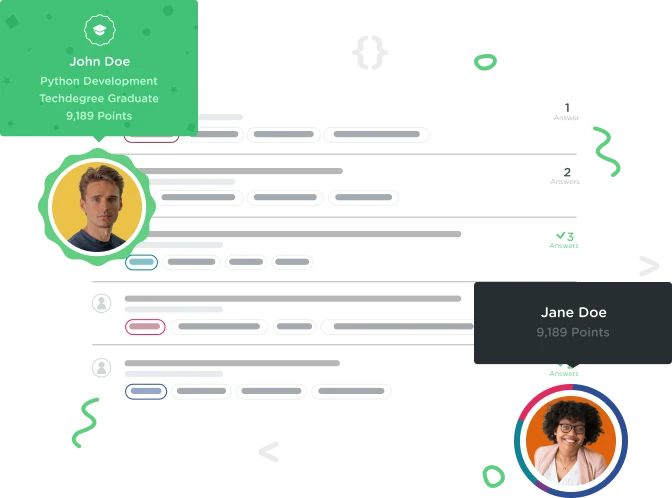
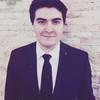
MIK 7
Courses Plus Student 881 Pointsidea's , how i can refactor it ? from shopping list, with a function named : main ,
i need to refactor this code from line 22 , idea's ?
shopping_list = []
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
show_help()
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
show_list(shopping_list)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
2 Answers
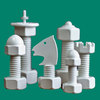
Steven Parker
230,995 PointsMoving code into a function is basically a 2-step process.
But both steps are pretty easy:
First, you'd put a def statement where the function begins, naming the function and it's arguments.
Then, you just indent everything that should be part of the function one more stop than it already is.
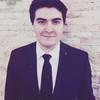
MIK 7
Courses Plus Student 881 Pointsgot it guys , thanks
Jeff Muday
Treehouse Moderator 28,720 PointsJeff Muday
Treehouse Moderator 28,720 PointsYou probably knew this already-- Python requires consistent indentation to run properly. In case that was caused by a cut-and-paste error, ignore my comment below:
I notice there are some indentation issues and some recursive calls happening which are definitely yielding syntax errors.
Issue 1: Recursion (e.g. the function calling itself) is present at the end of show_help() function and show_list() function. You probably don't want that, so we delete those lines.
Issue 2: we need to put the main loop inside a main() function. The loop and its body needs to be indented four spaces under the def main(). We also want to add the shopping list into the scope of the main() function. It could be declared at the very top as a global, but this is a choice a programmer can make. We also want to show_help() when the main function starts so the user knows what to do.
Note: if you are using Python 2.7, the input() would be changed to raw_input()
Lastly-- There is a fancy part at the end where Python asks if the name is 'main'. If it is, then it will execute the main() function. That is kind of a cool trick you will understand later.