Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial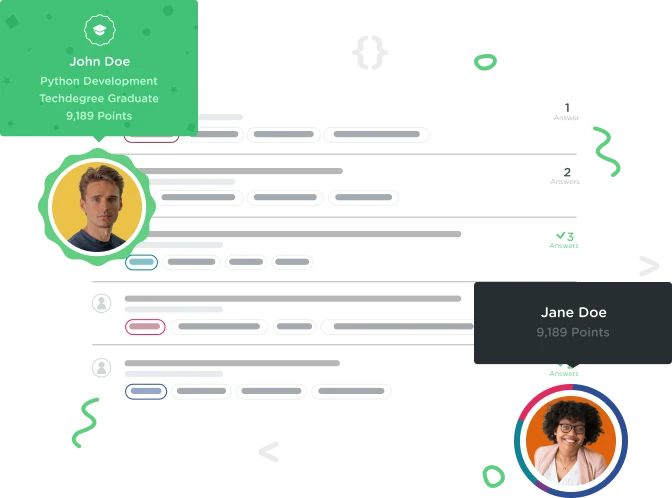

Dewi Tjin
2,275 Pointsif else statement is wrong...
I don't know why this won't pass..
def check_speed(car_speed)
# write your code here
if (car_speed == 40) && (car_speed == 50) && (car_speed < 50)
puts "safe"
end
3 Answers

giovannialvarez
6,487 PointsOK now I understand. The problem you're having is the grouping of these conditionals. There is an order of operations that takes place that I won't get into but basically you can treat conditionals like an algebra equation.
So
def check_speed(car_speed)
# write your code here
if (car_speed > 40) && ( car_speed < 50) || (car_speed == 40) || (car_speed == 50)
puts "safe"
end
Becomes
def check_speed(car_speed)
# write your code here
if ((car_speed > 40) && (car_speed < 50)) || ((car_speed == 40) || (car_speed == 50))
puts "safe"
end

giovannialvarez
6,487 PointsBecause car_speed can't be equal to 40 AND 50 at the same time, also car_speed can't be 50 AND less than 50 at the same time

Dewi Tjin
2,275 PointsSorry, made a mistake.. okay I changed the code but this still doesn't work..
def check_speed(car_speed)
# write your code here
if (car_speed > 40) && ( car_speed < 50) || (car_speed == 40) || (car_speed == 50)
puts "safe"
end
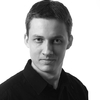
Maciej Czuchnowski
36,441 PointsYou need the following things:
1) add another end
after the if
` statement
2) remove puts
- they want you to return the string, not print it.
3) try using <=
and >=
operators to make the statement more readable and shorter
giovannialvarez
6,487 Pointsgiovannialvarez
6,487 PointsLike @Maciej says you could also use <= >= for readability
Dewi Tjin
2,275 PointsDewi Tjin
2,275 PointsAlmost worked.. missing another end.. thanks!!!
and this would work too: