Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial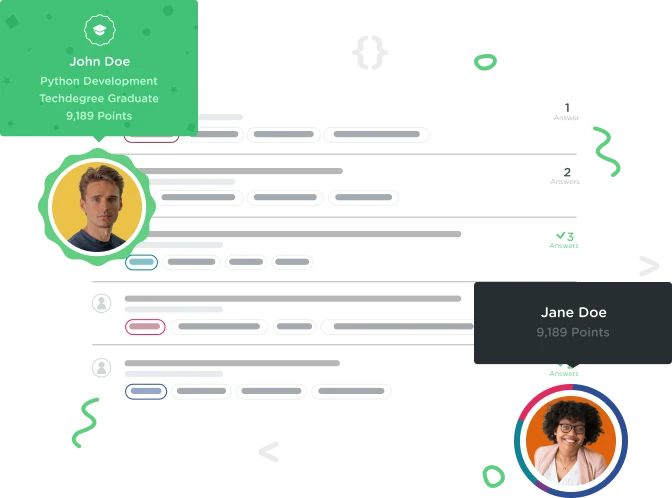

romankozak2
5,782 PointsIf global variable should be avoided in most cases, why does Dave continue to define global variables rather than local?
If global variable should be avoided in most cases, why does Dave continue to define global variables rather than local within particular loops or functions?
3 Answers

LaVaughn Haynes
12,397 PointsChyno is correct. If specifically you mean when he does something like this?
var student;
function getStudentReport( student ){
var report = '<h2>Student: ' + student.name + '</h2>';
report += '... more student message stuff...';
return report;
}
If so, the student parameter used in the function is not the same as the global variable student. With parameters, the function takes whatever is passed to it, like this
function print( message ){
console.log(message);
}
print('hello world');
and quietly in the background inside the function treats it like a local variable like this
{
//inside the function called via print('hello world');
var message = 'hello world';
console.log(message);
}
If you are referring to the use of the students variable inside the while loop: while loops don't have their own scope. Only the functions have scope. Any variables declared inside the loop would still be global. Plus it would be less efficient as the variable would keep being declared in every loop iteration. Thats why search and message are declared at the top and not in the while and for loops.
For now, I think that he is stressing the idea of "not using global variables within functions" more than "not using global variables at all". There will be more advanced techniques to avoid polluting the global scope down the line like immediately invoked function expressions (IFFE) but that might be unnecessarily confusing in a beginner level course.
For example:
;(function(){
/*
now student is not a global variable, but dave has
to go deeper into scope and other things that are not
necessary at this point */
var student;
function getStudentReport( student ){
var report = '<h2>Student: ' + student.name + '</h2>';
report += '... more student message stuff...';
return report;
}
})();
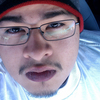
Chyno Deluxe
16,936 PointsWhile it is true that you should avoid global variables, In some cases, you'll need to have access to specific variables within different areas in your scripts.
If a variable is only used in one function and does not need to be access elsewhere. then the best thing would be to make it a local variable to the specific function.

romankozak2
5,782 PointsThank you! I definitely missed this: "while loops don't have their own scope. Only the functions have scope."
So loops do not have scope? That's extremely helpful. I just assumed that because it fell within the {}, local scope would apply.

LaVaughn Haynes
12,397 PointsCorrect. That threw me off initially as well. The curly braces {} of a function have scope, but the curly braces of loops and if statements DO NOT have scope. For example:
function someFunction(){
var foo = "I'm NOT global";
}
if(1 + 1 === 2){
var bar = "I am global";
}
for(var i = 0; i < 1; i++){
var baz = "I am global also";
}
bar and baz are global variables but you still want to decalre them at the top of the page.
- It's easier to read
//this is what you should do
var bar;
var baz;
if(1 + 1 === 2){
bar = "I am global";
}
for(var i = 0; i < 1; i++){
baz = "I am global also";
}
- If you don't, javaScript will automatically declare your variables at the top which could cause confusion. It's called hoisting. If you don't understand it now then don't worry. You will see it in the future.
//this is what javaScript did
//var bar;
//undefined
console.log(bar);
//Uncaught ReferenceError: whatever is not defined
console.log(whatever);
/*
You would think bar doesn't exist yet but
even though YOU have not declared bar yet...
(If it weren't for the error caused by trying to
log whatever), this function would run instead of
also throwing an error because bar was delared
up top by javaScript.*/
if(bar !== null && bar !== ""){
var bar = "I am global";
}