Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial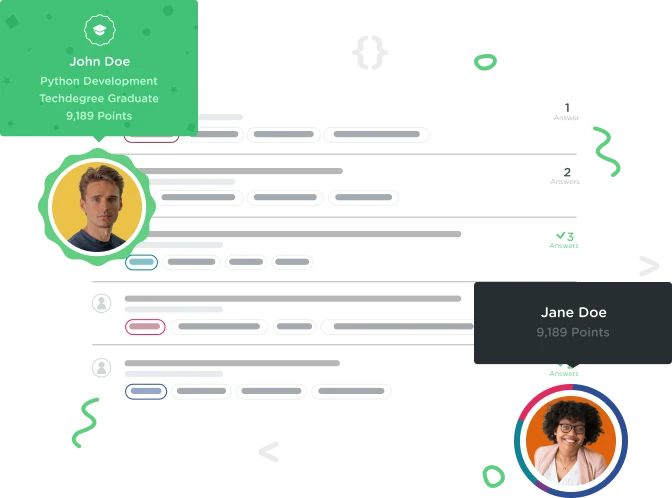
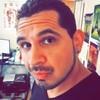
Daniel LittleThunder
6,975 PointsIf the filename contains the string "fun", display the name of the file surrounded by H3 tags.
Challenge Task 2 of 3
If the filename contains the string "fun", display the name of the file surrounded by H3 tags.
HINT: strpos will return 0 if the result is found at the beginning of the file. Returns FALSE if the needle was not found.
Allow me to preface this by stating that I am quite the noob when it comes to programming, so my question comes from lack of experience with the logic of PHP. My question is not asked because I'm stuck, but to better understand WHY the language works in the fashion it does. Below is the code:
<?php
//add code here
$files = scandir('example');
foreach ($files as $file) {
if (strpos($file, 'fun') !== FALSE) {
echo '<h3>' . $file . '</h3>';
}
}
My question is, why inside of the if control structure, when looking as the string function strpos, does the argument work as !== FALSE, or IS NOT FALSE, but does not work as == TRUE? The code below does not work:
<?php
//add code here
$files = scandir('example');
foreach ($files as $file) {
if (strpos($file, 'fun') == TRUE) {
echo '<h3>' . $file . '</h3>';
}
}
Logically, it would seem to me, that the comparison operator/boolean combination !== FALSE is the same as == TRUE, because 'is not false' means the same is 'is true'. I would think either could be used to return the same value when used inside of the function argument. Obviously this is not the case. I'm just wondering why.
I'm sure it has something to do with the way that strpos searches for the string and returns the Boolean, am I correct? From what I read in the PHP Manual, it would appear that strpos only returns a FALSE value, so it doesn't know what to do with a TRUE value, or evaluates everything matching the argument as FALSE, maybe?
Any explanation would be helpful. Thank you.
1 Answer

andren
28,558 PointsThere are two issues:
First the !== and == are actually not the same operators reversed. The inverse of !== is the === (Identical comparison) operator. The == operator is the value comparison operator, whose opposite would be !=.
Second If we were purely talking about Booleans then yes, not false and is true would be pretty much the same statement, but we aren't purely talking about Booleans. The strpos
function can return a Boolean (False
) or a number (the position the letter(s) where found). Lets say it returns the number 0.
With your first example the comparison would become 0 !== False
. That would result in True
(0 is not False
). With your second example the comparison would become 0 === True
which would result in False
(0 is not True
).
Both of the comparisons will find that the thing being compared is not equal to the thing it is compared to, but only the first comparison will return True
(since you use the not equal to operator) and since the if
statement only runs when it receives True
that is the code that makes the if
statement run.
Daniel LittleThunder
6,975 PointsDaniel LittleThunder
6,975 PointsOkay. That makes sense. I really appreciate the clear and concise explanation. Thinking in this type of logic is sometimes difficult for me, but I'm sure it gets easier with practice. Thank you for answering.