Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial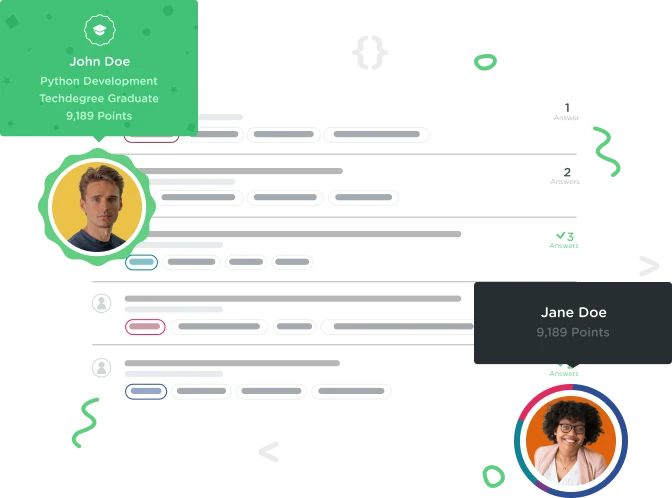

Gabriel Rogow-Patt
5,329 PointsI'm getting the error "IndexError: list assignment index out of range" on the "disemvowel" code challenge. Why is this?
Is there a quicker or more concise way of doing this? I feel like I'm doing extra steps, but I can't think of another way to do it...
def disemvowel(word):
list_word = list(word)
index = 0
for letter in list_word.copy():
if 'a' or 'e' or 'i' or 'o' or 'u' in letter.lower:
del list_word[index]
index += 1
word = ''.join(list_word)
return word
1 Answer

Wade Williams
24,476 PointsYou have a good code smell that you're doing extra work and when you feel that way, it's good to look at the entire code base. Here are some thoughts:
- Python will naturally loop over each character when given a string in a for loop, so no need to turn your word into a list first.
- We don't want to check if a vowel is in a letter, but instead check if a letter is in vowels. Let's create a vowels variable that holds all vowels.
- We're returning a joined list, so lets create a variable that's an empty list and add all non-vowels to it, then returned the joined list.
def disemvowel(word):
vowels = "aeiouAEIOU"
result = []
for letter in word:
if letter not in vowels:
result.append(letter)
return "".join(result)
Gabriel Rogow-Patt
5,329 PointsGabriel Rogow-Patt
5,329 PointsThanks! This really helps! Do you know what was actually making the error occur in my version? I’m just wondering because I want to understand why it didn’t work so I can avoid doing it in the future. I was making it a list so that I could use the del function, not for the loop, but I see that your way works better. Thanks again!
Wade Williams
24,476 PointsWade Williams
24,476 PointsThe reason you were getting a index error is because you were deleting letters from the list_word as you were looping and list_word was actually getting shorter as you went through each iteration and eventually you try to access an index value that exceeds the length of list_word.
Lets try disemvowel("Gabriel") for an example :