Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial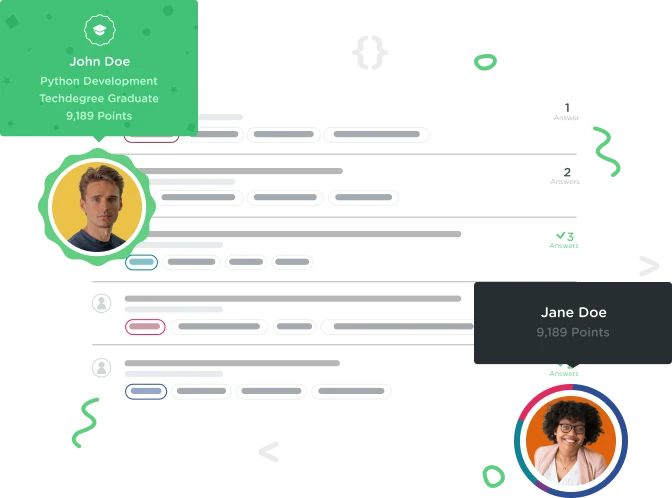

David Rosenman
875 PointsI'm pretty sure my code is correct... not sure why it is saying it isn't working. The output seems correct.
import random def even_odd(num): # If % 2 is 0, the number is even. # Since 0 is falsey, we have to invert it with not. return not num % 2 start = 5 while start: test_number = random.randint(1,99) if even_odd(test_number): print("{} is even".format(test_number)) else: print("{} is odd".format(test_number)) start = start - 1
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start:
test_number = random.randint(1,99)
if even_odd(test_number):
print("{} is even".format(test_number))
else:
print("{} is odd".format(test_number))
start = start - 1
2 Answers

andren
28,558 PointsYour code is indeed completely correct. After a bit of experimenting I have discovered that the cause of the issue seems to be the variable name you choose for the random number to be stored in. I do not know why (and this is definitively a bug on Treehouse's side) but the challenge will not accept the solution with the variable named test_number
but will accept it if you name the variable literally anything else.
This will work for example:
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start:
randNum = random.randint(1,99)
if even_odd(randNum):
print("{} is even".format(randNum))
else:
print("{} is odd".format(randNum))
start = start - 1
But even a less descriptive name like the letter a
is accepted, the only name not accepted is the one you happened to choose test_number
.
The code checker for these challenges will sometimes create some variables for your code automatically as part of its verification process, it's possible that you accidentally ended up naming your variable something that the code checker was using itself and therefore messing up its code. I do not know for sure but it's clearly a bug either way.
Maybe a mod like Jennifer Nordell can take a look at this and see if she can get it fixed. But until then you can bypass the bug by simply changing the name of your variable.
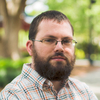
Kenneth Love
Treehouse Guest TeacherYep, you found a fun little bug!
I've logged it with the developers so it should be fixed soon.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHi there! I can confirm what @andren has experienced. If I simply rename your
test_number
totes_number
it passes. I'm inclined to agree with him on the theory that this is possibly a variable already use by the code used to run your code.Nice catch, andren! And thank you for your help in the Community!
Unfortunately, I'm not Treehouse staff and do not have any magical abilities like they do. However, I think in this case we might tag Kenneth Love.
But, in short, your code was just fine!