Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial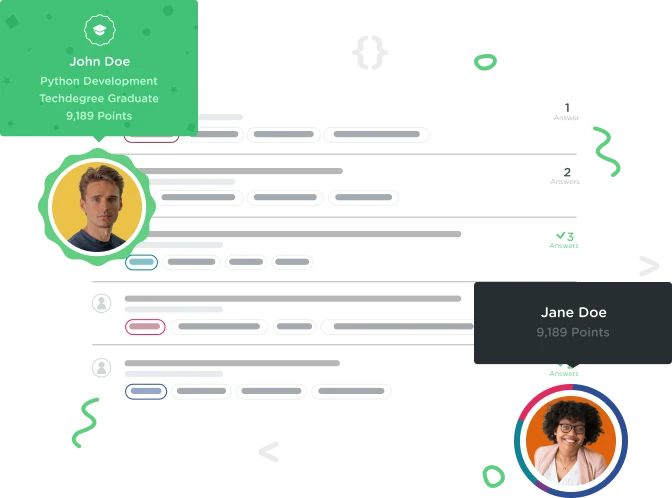

Emil Hejlesen
3,014 Pointsi'm stuck
someone please help
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
dict = {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def num_teachers(dict):
num_teachers = 0
for x in dict.keys():
num_teachers += 1
return num_teachers
def num_courses(dict):
courses = 0
for x in dict.values():
for y in x:
courses += 1
return courses
def courses(dict):
list = []
for x in dict.values():
for y in x:
list.append(y)
return list
def most_courses(dict):
high = 0
for teacher in dict:
if len(dict[teacher]) > high:
boss = []
boss.append(teacher)
return "".join(boss)
def stats(dict)
total = []
for x in dict:
total.insert(list(x))
2 Answers
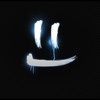
Grigorij Schleifer
10,365 PointsHe Emil,
you are on the right track. You can use a for loop and the items() method. You just need to append a list to total where the key will be the first element of the list and the length of the value will be the second element.
Try it out and report back if you need assistance.
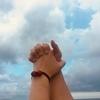
Yang Hu
7,782 Pointshi, my method is kinda overly complicated because I haven't got to tuple yet... but it worked:
def stats(dict):
master_list = []
personal_dict = {}
placeholder = 0
for i in dict:
personal_dict.update({placeholder: [i, len(dict[i])]})
placeholder += 1
for i in personal_dict.values():
master_list.append(i)
return master_list
But my goodness, Grigorij's code with the use of tuple is much easier and cleaner.
Emil Hejlesen
3,014 PointsEmil Hejlesen
3,014 Pointshey i tried again and i still couldnt get it working:
this is my code: def stats(dict): total = [] for x in dict.items(): total.append(list(x))
return total
the only problem is that i dont return the length of the number of courses please help :)
Emil Hejlesen
3,014 PointsEmil Hejlesen
3,014 Pointsi just dont know how to get the length of the list added to the end
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHi, you will get there Emil
See my code:
Makes sense?