Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial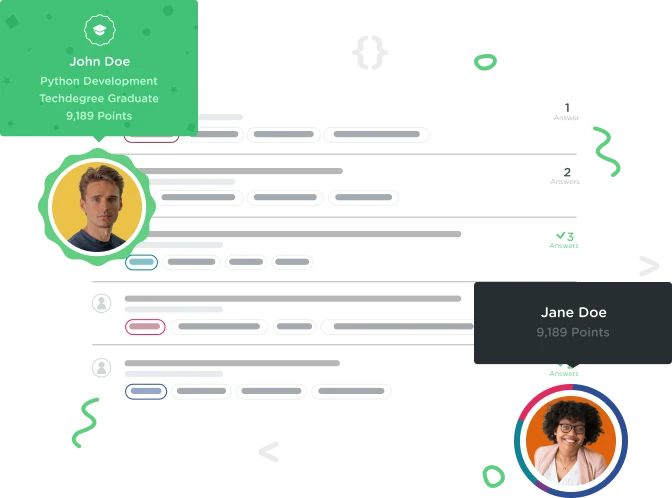

Dylan Merritt
15,682 PointsI'm trying to add input to devowel.py so that a user can enter the words they want devowled. Can anyone help me?
Here is the code I'm trying:
input = []
vowels = list('aeiou')
output = []
print('Enter the words that you would like devoweled. Type DONE when finished.')
while True:
word = input("> ")
if word == "DONE":
print('No more vowels!')
while True:
for vowel in vowels:
try:
word.remove(vowel)
except:
break
output.append(word)
else:
input.append(word)
print(output)
and here is the error I'm getting:
C:\Users\Dylan\Documents>python devowel.py
Enter the words that you would like devoweled. Type DONE when finished.
Traceback (most recent call last):
File "devowel.py", line 8, in <module>
word = input("> ")
TypeError: 'list' object is not callable
C:\Users\Dylan\Documents>
I've been using shopping_list.py and devoel.py as guides in building this and I can't figure out why it isn't working. Can anyone help?
3 Answers

Dan Johnson
40,533 PointsThe interpreter thinks you're trying to call your global variable "input" as a function, rather than the built-in function. Rename it and that should fix that.
Don't forget you'll need to iterate over each word the user has input, convert it to a list, and then attempt to remove the vowel (unless you want to use a different method, such as replace).

Dylan Merritt
15,682 PointsOkay, I changed 'input' to 'words' and that fixed the compiler error. I am now getting stuck here:
C:\Users\Dylan\Documents>python devowel.py
Enter the words that you would like devoweled. Type DONE when finished.
> Dylan
> Riley
> Lauren
> DONE
No more vowels!
I think it has to do with the second piece of advice you gave me. Could you elaborate on the iteration and list conversion?

Dan Johnson
40,533 PointsYou'll want to replace your inner "while True" loop with a loop that goes through each word in your list of user input. Currently you're just using the last word input, which is "DONE".

Dylan Merritt
15,682 PointsSo this is what I have so far. I feel like I'm getting close, but I'm having trouble implementing the user input part.
words = []
vowels = list('aeiou')
output = []
print('Enter the words that you would like devoweled. Type DONE when finished.')
for word_items in words:
word = list(word_items.lower())
for vowel in vowels:
while True:
try:
word.remove(vowel)
except:
break
output.append(''.join(word))
print(output)

Dan Johnson
40,533 PointsThe outer "while True" loop you had was fine for catching input. The inner one wasn't needed.
Here's an alternative solution I made for testing that may help you with the structure (You can still use the list methods with the try except blocks if you prefer):
user_input = []
output = []
vowels = list('aeiou')
print('Enter the words that you would like devoweled. Type DONE when finished.')
while True:
word = input("> ")
if word == "DONE":
print('No more vowels!')
for given in user_input: #Get each word from the list of input
given = given.lower() #Set input to lowercase to catch capitalized input.
for vowel in vowels:
given = given.replace(vowel, "") #str doesn't have the remove method, so we can use replace instead.
output.append(given)
break #The user has entered "DONE" and we've removed the vowels, we can exit the while loop.
else:
user_input.append(word)
print(output)
This method doesn't retain the original casing, though I don't think the one in the video does either.

Gordon Reeder
2,521 PointsYour line: word.remove(vowel) is working on the wrong data. I think you ment: words.remove(vowel)
In your else code block you can put a print(user_input) statement to check to see if the user_input list is being built correctly. This would be for debugging only. You would remove the print statement when you get your script working
Dylan Merritt
15,682 PointsDylan Merritt
15,682 PointsThank you so much! I think I really understand it all. I'm going to study this carefully.