Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial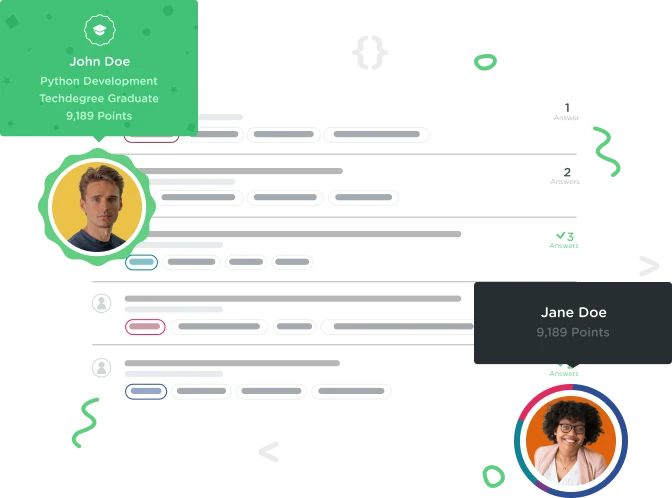

Milind Wankhede
3,571 PointsI'm trying to submit wordcount challenge. However, the console is erroring out. I tried my code locally and it worked.
First, it was complaining about the method get_key. I see that we can use that method with dict. Now, it just gives error 'Try again!' no details so, don't know how to make it run successfully. Any pointers would be appreciated! :)
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(str):
dataList = str.lower().split(' ')
wordCount = {}
for item in dataList:
if wordCount.has_key(item):
wordCount[item] += 1
else:
wordCount[item] = 1
return wordCount
1 Answer

JD Vila
8,095 PointsThe line if wordCount.has_key(item)
won't work, since dictionaries in python don't have that function. You could refactor that into something like:
def word_count(str):
dataList = str.lower().split(' ')
wordCount = {}
for item in dataList:
if item in wordCount.keys():
wordCount[item] += 1
else:
wordCount[item] = 1
return wordCount
I faced the same problem earlier, and a moderator pointed out that I was passing a space, or " " as a parameter in the split function, like split(" ")
, when it should have been split()
without any arguments, that way I could catch all forms of whitespace!
Also, your parameter str
is a class type, like int
or list
, so you might reconsider changing that to something like "string", or something else that's less confusing. Hope that helps!
Milind Wankhede
3,571 PointsMilind Wankhede
3,571 PointsThanks JD!