Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial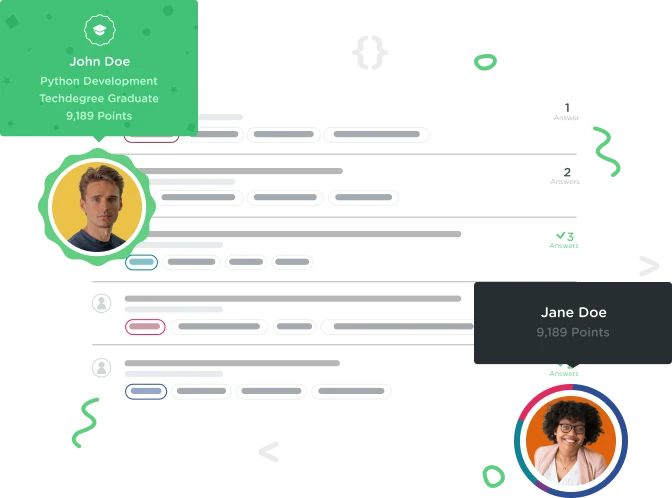

kali kali
Courses Plus Student 632 PointsImprove this code?
Hello,
How could I improve this code? Mine is pretty long...
Thank you for your answers :)
def disemvowel(word):
l = list(word)
test = True
while (test == True):
try:
l.remove('a')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('e')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('i')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('o')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('u')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('A')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('E')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('I')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('O')
except ValueError:
test = False
test = True
while (test == True):
try:
l.remove('U')
except ValueError:
test = False
word = ""
for i in range (len(l)):
word += l[i]
return word
3 Answers
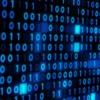
Alexander Davison
65,469 PointsThe shortest possible way I can think of is a whopping two lines of code. I used Python's List Comprehensions to accomplish this:
def disemvowel(word):
return ''.join([letter for letter in word if letter.lower() not in 'aeiou'])
If I was coding though, this is a little confusing and I'd avoid trying to use such a long list comprehension.
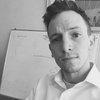
Matthew Connolly
iOS Development Techdegree Student 11,595 PointsHey Kali,
Here's an example of what I put together for disemvowel. The key to shortening up you code is to use an in
statement. Instead of removing the letter, I just checked it wasn't a vowel and added it to a new variable.
def disemvowel(word):
new_word = ''
for letter in word:
# edited this line of code from "if letter in ['a','e','i','o','u']"
if letter in 'aeiouAEIOU':
pass
else:
new_word += letter
return new_word
Edited: per Alexander's suggestions, compared letter against a string instead of a list and included checks for uppercase letters.
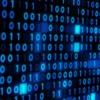
Alexander Davison
65,469 PointsYour code doesn't also check the uppercase "AEIOU" and also you don't need to make a list. This is also valid Python:
I'm in the Python shell
>>> 'a' in 'aeiouAEIOU'
True
>>> 'f' in 'aeiouAEIOU'
False
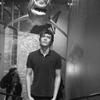
olegovich7
6,605 Pointsyou could use something like:
word = list(word)
for vowel in word:
if word.lower() in "aeiou":
word.remove(vowel)

kali kali
Courses Plus Student 632 PointsIt doesn't compile, word.lower() can't exist since word is a list
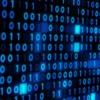
Alexander Davison
65,469 PointsYou should always test your code before posting. In this situation your code causes an error because you can't call "lower" on a list as Kali has said.
Matthew Connolly
iOS Development Techdegree Student 11,595 PointsMatthew Connolly
iOS Development Techdegree Student 11,595 PointsAlexander, you're right! Don't need to make a list to compare against - thanks for pointing that out. Going to update my file now.
Love the simplicity of your TWO LINE code implementation.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsREPEAT: If I was coding, I wouldn't use this method because it can be very confusing. Python was meant to be easily readable. :)
If I were coding on something, I would use something like your method.