Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial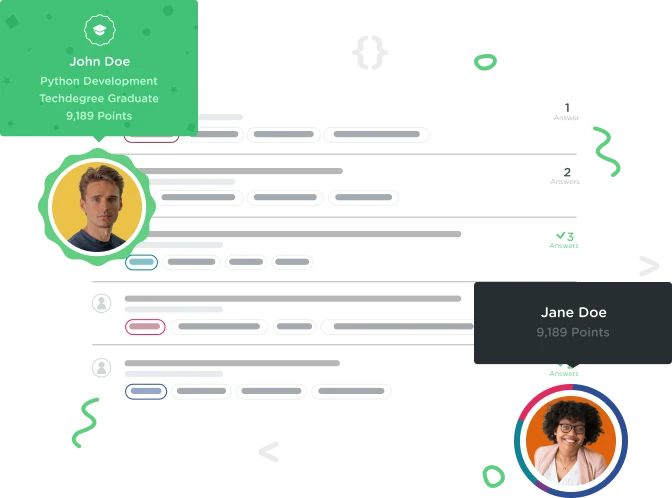

Derek Wong
Python Web Development Techdegree Student 888 PointsIncomplete disemvowel
For this challenge I was using an IELT to write down my code and used a trial word to test.
My word used was "Treehouse" and the output of my code was "Trhuse". I am struggling with this problem and would appreciate any help possible.
My code is as follows:
vowels = ["a", "e", "i", "o", "u"]
def disemvowel(word):
wordlist = list(word)
for x in wordlist:
try:
if x in vowels or x.lower in vowels:
wordlist.remove(x)
except ValueError:
pass
word = "".join(wordlist)
print word
word = "Treehouse"
disemvowel(word)
2 Answers
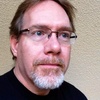
Chris Freeman
Treehouse Moderator 68,426 PointsYou very close to correct. Please correct these three errors:
You shouldn't alter the iterable used in a
for
loop. It causes items to be skipped. Instead, use a copy:
for x in wordlist.copy(): ## copy
The method
x.lower()
needs parens or else you would be comparing the existence of the lower
method, not its result.
The function needs to
return
a value and not just print
it.
Post back if you need more help. Good luck!!

ds1
7,627 PointsHi, Derek- this is not an easy challenge at this point in the Learn Python track, so don't feel bad. I found it quite difficult when I was first working through it! In your code above, you can use "for x in word" instead of "for x in wordlist". I think the reason your code is not taking out all of the vowels is because it's looping through a container ("wordlist") whose length is being changed (i.e. it's getting shorter) each time a letter is removed in "wordlist.remove(x)"... so I think it never gets through the entire original 'Treehouse' value, which is why it works for the first part of 'Treehouse' but not nearer to the end of 'Treehouse'. Try picking another word and seeing if the code does the same thing (i.e. removes the vowels like it should in the first part of a word but not near the end). Also, for this exercise, you'll want to use parentheses for your "print" function since we're working with Python 3 vs. 2. Also, I believe you can remove the "x in vowels" part of your "if" statement since your "x.lower() in vowels" (note the parentheses, like Chris mentions below) should take care of it.
-----More Stuff: There's some other ways to solve this as well. For example, here's a way that doesn't involve removing/deleting any letters:
def disemvowel(word):
vowel_list = list('aeiou')
result_list = []
for letter in word:
if letter.lower() not in vowel_list:
result_list.append(letter)
word = ''.join(result_list)
return word
There's (at least) one other way to do this too by using a list comprehension. Not that you need to be concerned with this right now, but you may want to make a mental note to check out Kenneth's workshop on comprehensions when you get further along... using a comprehension can be a nice shortcut.