Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial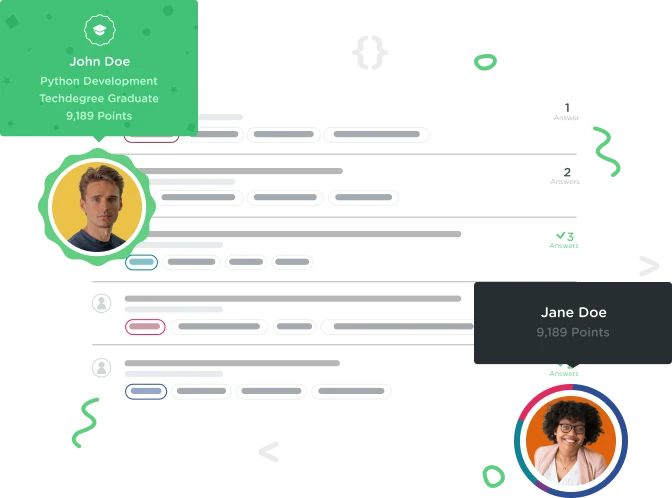
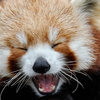
Randi Wilson
1,265 PointsInexplicable error caused by name not included in dictionary
I'm receiving errors like "Bummer! 'most_classes' returned Andrew Chalkley, expected 'Jason Seifer'. Instead of Andrew Chalkley, I've also seen 'Pasan Premaratne' and 'Dave Macfarland', in addition to 'Kenneth Love' (an expected incorrect answer here). Sometimes it will rotate through correctly and give me a correct answer, allowing me to proceed to the part 2. Immediately after, I'm receiving the exact same errors on part 2 and am forced to return to part 1.
I was able to fix the error by changing max_count = len(teacher) to max_count = len(teacher), but I’m still not sure why these errors were being returned in the first place.
Has anyone else seen errors like these, or might be able to explain where I'm getting these from?
It looks to be a bug to me, but Treehouse requested I put this into the forums just in case anyone else might be experiencing similar issues.
Thanks!
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(teachers):
max_count = 0
busy_teacher = ''
for teacher in teachers:
if len(teachers[teacher]) > max_count:
max_count = len(teacher)
busy_teacher = teacher
return busy_teacher
def num_teachers(teachers):
total_teachers = 0
for teacher in teachers:
total_teachers += 1
return total_teachers
7 Answers
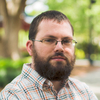
Kenneth Love
Treehouse Guest Teachermax_count
should be len(teachers[teacher])
since it should be the count of that teacher's classes, not the count of their name. You're comparing who has the most classes.
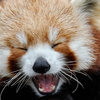
Randi Wilson
1,265 PointsGreat, thanks! I did fix that as well. Any ideas on where the other names might be coming from?
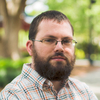
Kenneth Love
Treehouse Guest TeacherWhat I show in the comment there is only an example. It's not the actual dictionary that's fed to your function when it's validated.
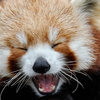
Randi Wilson
1,265 PointsAhhhh I just saw your last comment over here and finally put the pieces together. We shouldn't be making the dictionary, because the dictionary is provided and unknown. The teachers I was seeing in the errors were there all along, I just didn't have the visual on the dictionary. This has confused me for a few challenges now, so I'm glad to finally have figured out where I was going wrong!
Thanks for all your help!
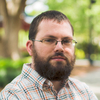
Kenneth Love
Treehouse Guest TeacherIt actually doesn't matter if you make the dictionary (outside of your function) or not, because the function will still use the one I give it when I test.
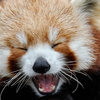
Randi Wilson
1,265 PointsGood to know, thanks!
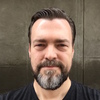
Jeffrey Hacker
8,379 PointsI get the same problem. I don't change anything and it cycles through names and then I pass the challenge eventually by continuing to submit my answer.
It then tells me part 1 has failed on the next challenge and sends me back to challenge 1 to start the whole process again.
Also, it refuses to acknowledge my function, but when I pass nothing in to the function, it suddenly recognizes that my function has no argument... I put the argument 'teachers' back in and now it doesn't recognize my function exists any longer.
extremely frustrating.
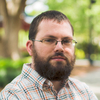
Kenneth Love
Treehouse Guest TeacherShare your code?
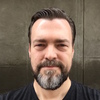
Jeffrey Hacker
8,379 Pointsthanks Kenneth sorry, I don't understand how to make the formatting look correct. The markdown example uses html, but I'm not sure if I'm actually supposed to use the <p> tags
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'], 'Kenneth Love': ['Python Basics', 'Python Collections']}
def most_classes(teachers):
teacher_most = ""
class_count = 0
for teacher in teachers:
if len(teachers[teacher]) > class_count:
teacher_most = teacher
return teacher_most
def num_teachers(teachers):
total_number = 0
for _ in teachers:
total_number += 1
return total_number
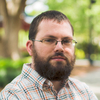
Kenneth Love
Treehouse Guest TeacherSo let's just focus on your for
loop in most_classes
. You compare the length of the current teacher's value against class_count
. Good job, that's what you should do. But, inside of there, you only update teacher_most
. Well, ever teacher is going to have more than 0 courses so every teacher is going to pass that test!
You need to update the class_count
, too.
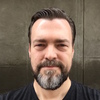
Jeffrey Hacker
8,379 PointsI must have deleted that line during my attempts to format my text, but I do increment class_count in my actual code.
I am currently getting the error "Bummer! Where's 'most_classes()'? If I remove the argument 'teachers' the challenge notices the problem, but when I put it back in, I get the 'bummer' error again like it can't see it.
Am I still making a mistake here?
teachers = {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
teacher_most = ''
class_count = 0
def most_classes(teachers):
for teacher in teachers:
if len(teacher[key]) > class_count:
class_count = len(teacher[key])
teacher_most = teacher
return teacher_most
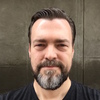
Jeffrey Hacker
8,379 PointsNever mind, I've copied back and forth so many times i don't think I even have the same code now as I've been fighting this for a couple hourse. thanks anyway
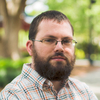
Kenneth Love
Treehouse Guest TeacherI've updated the CC a bit to provide a little more feedback, especially on the error that you were triggering.
Keep teacher_most
and class_count
inside of most_classes
. Also, in your last example, where did key
come from?
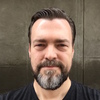
Jeffrey Hacker
8,379 Points'key'. that was in an older version of my code as I worked through this. I had copied code off to another location because I kept losing it when the browser would refresh... but it turns out that this was not my latest. I've lost all of it now.
I feel like I've derailed this thread even though originally I was having some odd issues that matched it.
I have now started over with a fresh browser too, just in case that was causing the odd browser errors.
Randi Wilson
1,265 PointsRandi Wilson
1,265 PointsA full screenshot can be found posted here.