Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial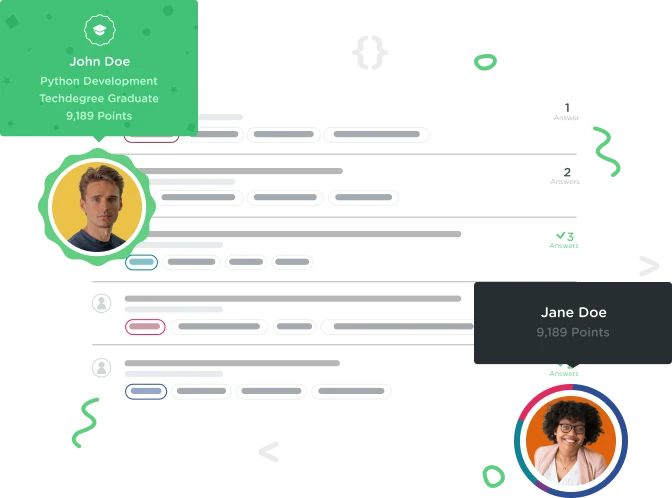
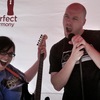
Martin Cross
4,593 PointsIs less code and/or variables always more efficient?
Below is my solution where I actively tried to reduce the number of variables and lines of code that I use. Is this even worth the effort? Is this actually making my code more efficient?
var input = prompt('Give me a low number'); var low_int = parseInt(input);
input = prompt('Give me a high number'); var high_int = parseInt(input);
document.write('The number between ' + low_int + ' and ' + high_int + ' that I was thinking of was ' + (Math.random() * (high_int - low_int) + low_int));
1 Answer
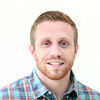
Daniel Botta
17,956 PointsYou generally would not want to write like that for the reason of keeping organized code and readability. When scanning through that, it is very easy to miss the variables on the right. It won't affect the the file size and speed of the page much so I'd say it's not worth it.
Also, I'd say it's good to use many variables. Let's say you have a variable called "name" that stores the value of Brian. Let's say you have that variables used 20 times throughout the script. If you had typed out "Brian" everytime you used it you'd have to go back and individually find all of the places that you find the name "Brian" written out. But with a variable, you only have to change it in one place and it will update all of the other places that use that variable.
What I recommend is write out your code first so that it is very readable. Then when you are ready to use it/apply it to a site, minify it which wil remove all of the white space. But make sure to keep the original file in case you need to go back and make some edits.
Martin Cross
4,593 PointsMartin Cross
4,593 PointsApologies. I just learned how to post code properly. My code was originally formatted like the below, but was re-formatted by the forum's post method (hope I'm using that reference correctly).
Also, regarding the number of variables, would my 3 variable solution be considered a more efficient usage of memory (in theory and in larger scale practice) than, say, a 4 variable solution similar to the below? Or is there something about the JavaScript interpreter that makes this a non-issue?