Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial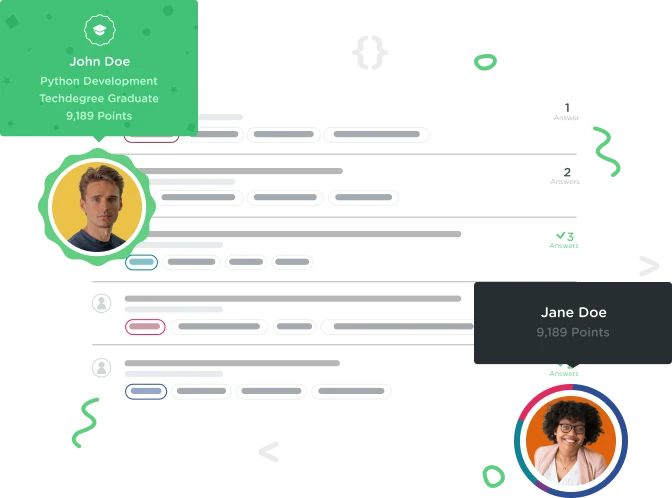

Jay Wilson
1,494 PointsIs there a better way to code the solution than what I have done?
Here is the code that I wrote to solve the challenge:
var answeredCorrectly = 0;
var question1 = prompt('What is my name?');
if (question1 === 'Jay' || question1 === 'jay') {
answeredCorrectly ++;
}
var question2 = prompt('What is my middle name?');
if (question2 === 'edward' || question2 === 'Edward') {
answeredCorrectly ++;
}
var question3 = prompt('What is my last name?');
if (question3 === 'wilson' || question3 === 'Wilson') {
answeredCorrectly ++;
}
var question4 = prompt('What is my mom\'s name?');
if (question4 === 'ruby' || question4 === 'Ruby') {
answeredCorrectly ++;
}
var question5 = prompt('What is my nickname?');
if (question5 === 'Big Sexy' || question5 === 'big sexy') {
answeredCorrectly ++;
}
var prize = ''
if (answeredCorrectly === 5) {
prize = 'a Gold Crown';
} else if (answeredCorrectly === 3 || answeredCorrectly === 4) {
prize = 'a Silver Crown';
} else if (answeredCorrectly === 2 || answeredCorrectly === 1) {
prize = 'a Bronze Crown';
} else {
prize = 'absolutely nothing. Try again';
}
var message = '<p>You answered ' + answeredCorrectly + ' questions correctly.</p><p>You win ' + prize + '</p>'
document.write(message);
3 Answers
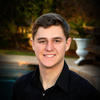
Colton Ehrman
Courses Plus Student 5,859 PointsFor all your if statements, you could do
if (question1.toLowerCase() === 'answer')
Instead of having to check for different cap inputs, this will work for all no matter what the user inputs, just make sure that you use an all lowercase 'answer' when checking.
Other than that looks pretty good :)
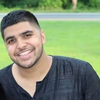
Aman Mundra
2,755 PointsI think you did a good job with your code.
I might make a syntactical change:
answeredCorrectly++
(Normally I see textbooks not including a space between the ++ and the variable name).
That's about it.

eric Weintraub
1,845 PointsHi Jay,
The majority of your code is redundant and any changes would require you to add the same code in multiple areas (for instance, if you wanted to add an alert to let the user know if they got it right you would have to do that in 5 places). More typing leaves more room for error. May I suggest using a for loop to go through your questions/answers and storing them in an array (http://www.w3schools.com/js/js_loop_for.asp):
var questions = [{ 'question': 'What is my name?', 'answer': 'jay' }, { 'question': 'What is my middle name?', 'answer': 'edward' }, { 'question': 'What is my last name?', 'answer': 'wilson' }, { 'question': 'What is my mom\'s name?', 'answer': 'ruby' }, { 'question': 'What is my nickname?', 'answer': 'big sexy' } ];
var answeredCorrectly = 0;
for (var i = 0, l = questions.length; i < l; i++) { var r = prompt(questions[i].question); if (r.toLowerCase() == questions[i].answer.toLowerCase()) { answeredCorrectly++; } }
This way any change only needs to be typed once.
For your prizes, we can use a switch (http://www.w3schools.com/js/js_switch.asp):
var prize; switch(answeredCorrectly){ case 5: prize = 'a Gold Crown'; break; case 3,4: prize = 'a Silver Crown'; break; case 1,2: prize = 'a Bronze Crown'; break; default: prize = 'absolutely nothing. Try again'; }
Again, this allows you to remove redundant code which will help make it cleaner, easier to read and easier to debug. With 5 questions answers this is not a big deal, but what if you had 20 questions?
Here is the full working example: https://jsfiddle.net/n4q0jf92/
-Eric
Kirby Abogaa
3,058 PointsHi Eric!
I believe the "for loop" and "switch" were not yet discussed when this challenge was given.
Only "if and else if statements".