Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial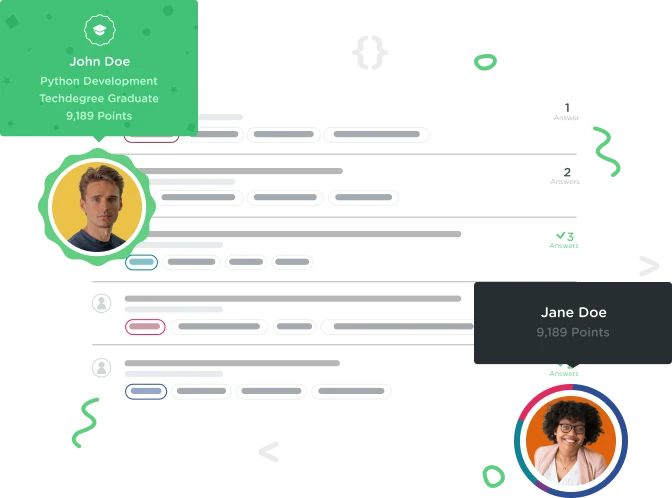
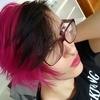
Kat Cuccia
786 PointsIs there a cleaner way to code this?
In the "Conditionals" challenge, it asks you to implement an if-then statement to display text depending on variable input for two separate sets of variables. Is it possible to condense my two if-then statements to one and still get the same result, and if so, how?
My code, for reference:
if ($studentOneGPA == 4.0) {
echo $studentOneName . " made the Honor Roll";
} else {
echo $studentOneName . " has a GPA of " . $studentOneGPA;
}
if ($studentTwoGPA == 4.0) {
echo $studentTwoName . " made the Honor Roll";
} else {
echo $studentTwoName . " has a GPA of " . $studentOneGPA;
}
2 Answers
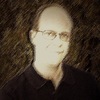
Jason Anders
Treehouse Moderator 145,860 PointsHey Kat,
That's a very good question for the Community!
There are probably a couple ways you could check the logic, but it would probably end up with the same number of (or more) lines of code and become pretty complex.
Because you are checking two separate variables, in my opinion, what you have is the cleanest way to do it. It's readable, and you know exactly what (or, in this case, who) is being checked and against what. I think combining them would become complicated and definitely not as readable.
Good Job & Keep Coding! :)
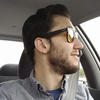
Benjamin Larson
34,055 PointsI agree with Jason that with that as far as the conditional logic goes, to try to check the state of multiple students inside the IF statements would just get messy. But eventually you'll learn about functions and arrays in PHP, and that will give you the tools to condense this kind of code into something that might look like this:
<?php
// function accepts a $student array containing the student's name and GPA
function displayStudentDetails($student) {
if ($student['GPA'] == 4.0) {
echo $student['name'];
} else {
echo $student['name'] . 'has a GPA of ' . $student['GPA'];
}
}
displayStudentDetails($studentOne);
displayStudentDetails($studentTwo);
Don't worry if that seems confusing now or if it seems like more work. Just know that as you learn more, you'll discover more tools and patterns for making your code more efficient. Sometimes when learning something new, it seems like it's more complicated as first, but then you realize the benefit later on. In this instance, you could keep re-using a single function to check any number of students with a single call to the function.
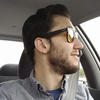
Benjamin Larson
34,055 PointsThis method also won't work for this particular code challenge, since it's expecting you to solve it in the manner which you posted above. This was just to affirm the fact that you were correct to notice that you were repeating a lot of the same code. That's a good thing to recognize because it often is a sign that you could refactor your code to make it more efficient.
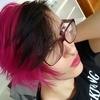
Kat Cuccia
786 PointsYeah, I did wonder if an array could be used, but since I don't really know how to use them, I was thinking more along the lines of a "simplified" solution for this question. Thank you though :) I can't wait to find out what all that means lol
Kat Cuccia
786 PointsKat Cuccia
786 PointsThank you! c: