Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial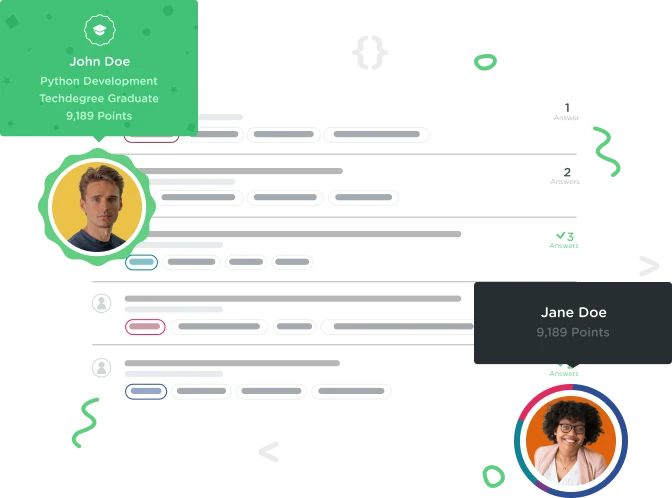
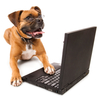
Sam S
4,447 PointsIs there any advantage to using a do while loop in this exercise?
I wrote the exercise like this:
while ( parseInt(guess) !== randomNumber ){ guess = prompt("Guess a number between 1 and 10:"); guessCount += 1; } Because the variable guess was already declared in the beginning of the script, I don't see why a do while loop is any better than a plain old while loop in this context. Can someone explain? Thanks!
2 Answers
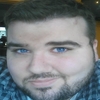
Marcus Parsons
15,719 PointsHi Sam,
The real difference between do while and while loops is when they check their conditional statement. do while loops execute the code within the code block and then check the conditional statement to see if it is true. while loops check the conditional statement to see if it is true and then runs the code within the block if it is. Both of them run until either the condition is false or they are broken with a break
command.
You could just as easily do the same code present in this course with a while loop (and it will still look clean):
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
while (!correctGuess) {
guess = prompt('I am thinking of a number between 1 and 10. What is it?');
guessCount += 1;
if (parseInt(guess) === randomNumber) {
correctGuess = true;
}
}
document.write('<h1>You guessed the number!</h1>');
document.write('It took you ' + guessCount + ' tries to guess the number ' + randomNumber);

Daniel Newman
Courses Plus Student 10,715 PointsIt's a difference between:
var getPromt = promt(....);
if(.....) {
} else if {
} else if {
}
while ( getPromt !== guess ) {
// the same bunch of logic we have before
}
vs.
var getPromt = promt(....);
do {
if(.....) {
} else if {
} else if {
}
// one set of logic
} while ( getPromt !== guess );
It's more clean and understandable code.
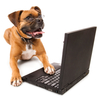
Sam S
4,447 PointsThanks, Marcus. Your response makes sense. For some reason I'm already partial to while loops (instead of do while) because it's easy to see the condition upfront. Maybe someday I'll come across a scenario where a do while loop really makes more sense to use.
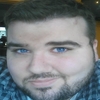
Marcus Parsons
15,719 PointsYou're welcome, Sam. There may be a scenario pop up where a do while is better than a while to use, but for most coding scenarios, you can switch in between using a while or do while loop. Cheers!