Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial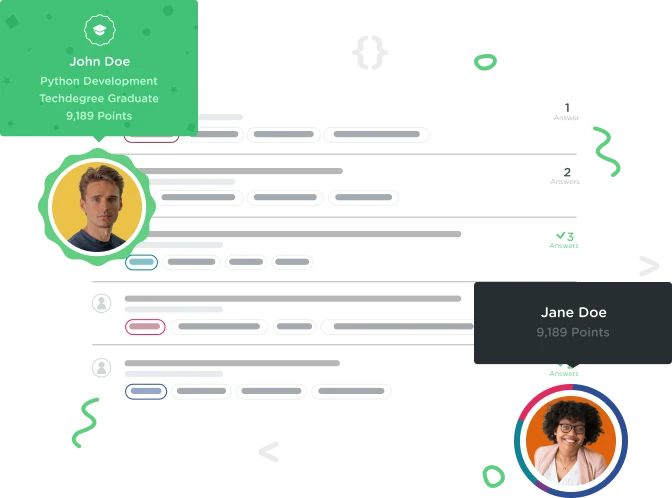
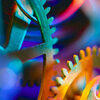
ja5on
10,338 PointsIs this a callback?
I was struggling last night to get this in my head, but woke up this morning and created a callback... I think....
function callback() {
console.log("Hi");
}
function callingTheCallback (callback) {
console.log("Oh hello");
}
callingTheCallback(callback())
Outputs:- Hi
Outputs:- Oh hello
Appreciate the help.
8 Answers

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsOk, to explain in your own example see this:
function mycallback() {
console.log("Hi");
}
function callingTheCallback(callback) {
console.log("Oh hello");
callback();
}
callingTheCallback(mycallback);
I only renamed the original callback function to mycallback for better distinction. Here you'll see that the output changes in order. Here you really call the callback from your function inner block and you have full control. Your initial code only has a proof because you simply log into the console. And of course this happens already on your argument. For later working with real callback with values this won't work.
To simplify: to call the callback inside the inner block of a function makes it a callback!
Hope this helps for clarification!

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsHi Jason, actually you're really close to it. But to simplify let's say a callback is a function given to another function which calls it later on a certain time. In your example it outputs both to the console because you call both functions. But a callback is not called when given as argument.
Example:
function callTheCallback(callback) {
callback('hello world');
}
callTheCallback(console.log);
callTheCallback(window.alert);
Here you give the predefined methods console.log
and window.alert
as callback each into your function. They get both called then in your function code. See the missing () when given as argument. This is because they are only a reference and can be called later on. This is a callback. Often you'll find callbacks when working with asynchronous code which calls your callback at an undefined time.
Hope this helps. Happy coding!
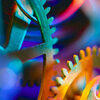
ja5on
10,338 PointsBut here your using one function, the teacher uses two functions one to call another. If fact the teacher says a callback is one function calling another, not two arguments.

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsYes right, one function calling another... but not when given as argument, only inside the function block it's called.
When you call the callback function with () in between the callTheCallback's (), you only give the value of the callback function into the other. But you don't want to give the value but the function reference itself.
console.log and window.alert are both methods (functions) itself. So when you reach them into your function you can say that you use them as callback. You could also rewrite to use your own functions and variables, for example with two different callbacks to be more clear:
function myCallbackOne(value) {
console.log(value);
}
function myCallbackTwo(value) {
window.alert(value);
}
function callTheCallback(callback, value) {
callback(value);
}
callTheCallback(myCallbackOne, 'hello from myCallbackOne');
callTheCallback(myCallbackTwo, 'hello from myCallbackTwo');
Here you have two different callbacks and you can use both with a value. See again when calling the callTheCallback() we only give the function name as argument and do NOT call it. The callTheCallback function itself calls them with the value you also give to it. Also you label them inside your function just as "callback" which can be pretty everything.
Just remember "myCallbackOne" is just the function name/reference but "myCallbackOne()" (with parentheses) is when the function is called and the value is returned. This is a huge difference.
Maybe you find more deeper explanation here: https://en.wikipedia.org/wiki/Callback_(computer_programming)
Hope this helps. Good luck!
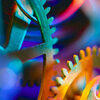
ja5on
10,338 PointsDon't get how yours is callback and mine isn't, i've requested help from support.

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsLook again the difference between following two, look for parentheses with the argument:
callingTheCallback(callback()); // wrong
and
callingTheCallback(callback); // right
Let's imagine your callback returns a value instead of doing side-effects (logging to console). See following example where the first is correct and the second throws an error because you only receive the value from the call to your function:
function callback() {
return 5;
}
function callingTheCallback(myCustomCallback) {
return myCustomCallback();
}
var right = callingTheCallback(callback); // the variable right has now the value of 5
console.log(right); // logs 5 to the console
var wrong = callingTheCallback(callback()); // this actually calls callingTheCallback(5) and throws error because 5 cannot be called in line above "return myCustomCallback();" becaue "return 5();" is not valid
It took me many hours in the beginning of learning function concepts. And still after many years I have to rethink when reading/writing code. But just make yourself clear that you can reach around function references that can be called later from another function.
Keep it up and good luck!
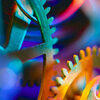
ja5on
10,338 PointsBut i get the proof from running the program, so callingTheCallback(callback()) works its accessing another function and return that function and itself, that's why i get two outputs... From what youve said callingTheCallback(callback) only returns oh hello and so doesnt reach into the other function - a callback accesses another function which is what has happened with my original code...
Your code I can't follow btw thats why i refer to the original

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsThe second function expects a function as parameter and we label it "callback" in our definition. Below we call our second function with the argument of the first function (but do not call the first function itself, see without parentheses). Now when second function is called it has a reference to the first one. Then it first logs "Oh hello" and second it calls the callback. The call to the callback results in logging "Hi".
Maybe it becomes more clear to you if we add a different third function (or another callback so to say):
function callback() {
console.log("Hi");
}
function anotherCallback() {
console.log("bye");
}
function callingTheCallback(callback) {
console.log("Oh hello");
callback();
}
callingTheCallback(callback);
callingTheCallback(anotherCallback);
Run this and see what happens.
Here in Treehouse is a course about functions in JavaScript: https://teamtreehouse.com/library/javascript-functions
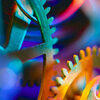
ja5on
10,338 PointsSorry it's my own annoyance at myself at what seems incredibly hard - I've come so far and even doing function course four times but callbacks seem inpossible.

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsNo problem, Jason! Don't worry, try more and play around with your code and the code from others. Make it, break it. This will help you better understand what's happening and becoming a senior level developer at the end.
There is a good book about JavaScript free available to read online: https://eloquentjavascript.net/. I can highly recommend it and the author has an amusing writing style.
Keep it up and happy coding! Thanks for choosing best answer
ja5on
10,338 Pointsja5on
10,338 Pointswhat is the callback(); inside the second function? A method?