Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial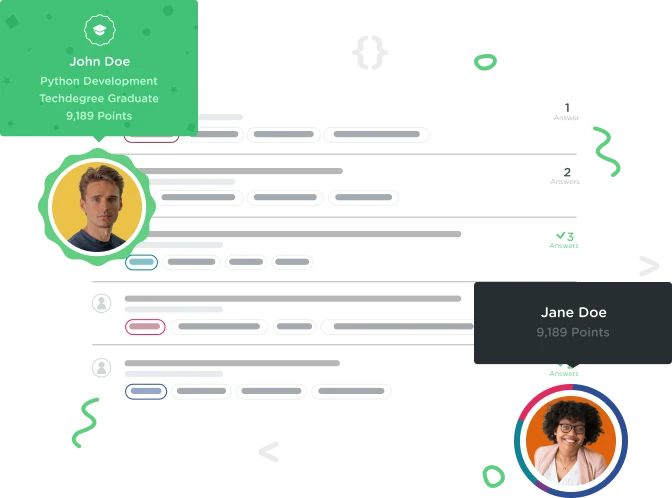

ibbhfsmeaf
4,568 PointsIs this done "efficiently"?
I have yet to watch Dave's solution, and I will probably have watched it by the time I get a response. Can someone tell me if my code is done properly? What I mean by that is I am always getting told my code is not "efficient", although it has the intended outcomes. I have been trying to work on that. My code works just as Dave wants it to work and I did it all from my own knowledge and it took about 20 minutes.
Any advice/comments/tips would be helpful.
var quiz = [
['What is the color of grass?', 'green'],
['What is 1 + 3?', 4],
['Who was the first president of the USA?', 'george washington'],
['Who is the current president?', 'barrack obama'],
['How many fingers do you have?', 10]
];
var answersCorrect = 0;
var answersCorrectCount = '';
var answersWrong = 0;
var answersWrongCount = '';
var listHTML = '<ol>';
var listHTML2 = '<ol>';
function print(message) {
document.write('<p>' + message + '</p>');
}
while (true) {
for (var i = 0; i < quiz.length; i++) {
var answer = prompt(quiz[i][0]);
if (answer === quiz[i][1] || parseInt(answer) === quiz[i][1]) {
answersCorrect++;
answersCorrectCount = quiz[i][0];
for (var listHTML_ol_count = 0; listHTML_ol_count < answersCorrect; listHTML_ol_count++) {
listHTML += '<li>' + answersCorrectCount + '</li>';
break;
}
} else {
answersWrong++;
answersWrongCount = quiz[i][0];
for (var listHTML2_ol_count = 0; listHTML2_ol_count < answersWrong; listHTML2_ol_count++) {
listHTML2 += '<li>' + answersWrongCount + '</li>';
break;
}
}
}
break;
}
listHTML += '</ol>';
listHTML2 += '</ol';
print('You got ' + answersCorrect + ' question(s) right');
print('You got these questions correct:');
print(listHTML);
print('You got these questions wrong:');
print(listHTML2);
8 Answers
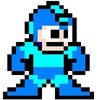
Robert Richey
Courses Plus Student 16,352 PointsHi Lansana,
Nice job working that out on your own! After reading through your code, I was able to delete some of it - only one loop is needed. The only addition was a closing bracket for listHTML2
.
Thanks for sharing :)
Cheers
var quiz = [
['What is the color of grass?', 'green'],
['What is 1 + 3?', 4],
['Who was the first president of the USA?', 'george washington'],
['Who is the current president?', 'barrack obama'],
['How many fingers do you have?', 10]
];
var answersCorrect = 0;
var answersCorrectCount = '';
var answersWrong = 0;
var answersWrongCount = '';
var listHTML = '<ol>';
var listHTML2 = '<ol>';
function print(message) {
document.write('<p>' + message + '</p>');
}
for (var i = 0; i < quiz.length; i++) {
var answer = prompt(quiz[i][0]);
if (answer === quiz[i][1] || parseInt(answer) === quiz[i][1]) {
answersCorrect++;
answersCorrectCount = quiz[i][0];
listHTML += '<li>' + answersCorrectCount + '</li>';
} else {
answersWrong++;
answersWrongCount = quiz[i][0];
listHTML2 += '<li>' + answersWrongCount + '</li>';
}
}
listHTML += '</ol>';
listHTML2 += '</ol>'; // added closing bracket
print('You got ' + answersCorrect + ' question(s) right');
print('You got these questions correct:');
print(listHTML);
print('You got these questions wrong:');
print(listHTML2);

ibbhfsmeaf
4,568 PointsThank you for your response. I added the next part Dave taught to my print function, and the outcome isn't exactly desirable - the program only prints out how many questions I got wrong in an ordered list but completely ignores everything else. But when I change the print function back to the normal document.write() it works fine. Can you help me fix this issue?
Here is the updated code that isn't working properly:
var quiz = [
['What is the color of grass?', 'green'],
['What is 1 + 3?', 4],
['Who was the first president of the USA?', 'george washington'],
['Who is the current president?', 'barrack obama'],
['How many fingers do you have?', 10]
];
var answersCorrect = 0;
var answersCorrectCount = '';
var answersWrong = 0;
var answersWrongCount = '';
var listHTML = '<ol>';
var listHTML2 = '<ol>';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for (var i = 0; i < quiz.length; i++) {
var answer = prompt(quiz[i][0]);
if (answer === quiz[i][1] || parseInt(answer) === quiz[i][1]) {
answersCorrect++;
answersCorrectCount = quiz[i][0];
listHTML += '<li>' + answersCorrectCount + '</li>';
} else {
answersWrong++;
answersWrongCount = quiz[i][0];
listHTML2 += '<li>' + answersWrongCount + '</li>';
}
}
listHTML += '</ol>';
listHTML2 += '</ol>'; // added closing bracket
print('You got ' + answersCorrect + ' question(s) right');
print('You got these questions correct:');
print(listHTML);
print('You got these questions wrong:');
print(listHTML2);
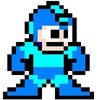
Robert Richey
Courses Plus Student 16,352 PointsCan you post the HTML file also please?

ibbhfsmeaf
4,568 PointsSorry, here it is:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
</head>
<body>
<h1>JavaScript Quiz</h1>
<div id="output">
</div>
<script src="playlist.js"></script>
</body>
</html>
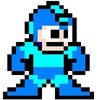
Robert Richey
Courses Plus Student 16,352 PointsThe text being stored in the div keeps changing with each call to print, and is over-written by the value in message. That's why the only thing you see is the last thing sent to print.

ibbhfsmeaf
4,568 PointsHow can I counter this? I'm thinking something along the lines of either more divs and more print functions so that each print function has its own unique div, thus allowing them all to print... but that idea raises alarms because Dave said to never repeat myself.
edit: wait.. can I use a class instead of an id and put however many div's I need but still keep the same .js code? (going to try it now)
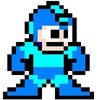
Robert Richey
Courses Plus Student 16,352 PointsYou can either build the entire output into one call to print, or concatenate the contents of message to the div.
outputDiv.innerHTML += message;

ibbhfsmeaf
4,568 PointsThank you very much.
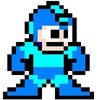
Robert Richey
Courses Plus Student 16,352 PointsSure, my pleasure. I couldn't help but take another look at the code, and sticking with the theme of this thread on 'efficiency', I'd recommend building your entire output into one call to print.
var quiz = [
['What is the color of grass?', 'green'],
['What is 1 + 3?', 4],
['Who was the first president of the USA?', 'george washington'],
['Who is the current president?', 'barrack obama'],
['How many fingers do you have?', 10]
];
var answersCorrect = 0;
var answersCorrectCount = '';
var answersWrong = 0;
var answersWrongCount = '';
var listHTML = '<ol>';
var listHTML2 = '<ol>';
var output = '';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
for (var i = 0; i < quiz.length; i++) {
var answer = prompt(quiz[i][0]);
if (answer === quiz[i][1] || parseInt(answer) === quiz[i][1]) {
answersCorrect++;
answersCorrectCount = quiz[i][0];
listHTML += '<li>' + answersCorrectCount + '</li>';
} else {
answersWrong++;
answersWrongCount = quiz[i][0];
listHTML2 += '<li>' + answersWrongCount + '</li>';
}
}
listHTML += '</ol>';
listHTML2 += '</ol>'; // added closing bracket
output += 'You got ' + answersCorrect + ' question(s) right';
output += 'You got these questions correct:';
output += listHTML;
output += 'You got these questions wrong:';
output += listHTML2;
print(output);

ibbhfsmeaf
4,568 PointsI see what you did there. Thanks a lot!
One more question.. I am very ambitious and I like to take on way more than I can in a small amount of time. I'm already at 2804 score and I made my Treehouse account 11 days ago. I feel as though I'll hit a point where I can no longer retain information because of information-overload. Do you recommend keep going as I am as long as its working for me, or learn one day, practice the next, and so forth? My current routine is basically learn every day, with practice in-between learning sessions.
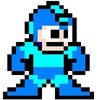
Robert Richey
Courses Plus Student 16,352 PointsI don't have any good advice for you in this regard, because I struggle with this myself. Our score is just a pat-on-the-back for effort, but what really matters is retaining that information - and the best way to do that is to apply it. Apply it to a project, or to helping others on the forum.
For myself, by trying to help others with their code, I actually learn a lot in the process and it helps me to retain the information as I go through some challenge for the n'th time.
I still forget a lot about the fundamentals, but what makes me better than my younger self, is that I'm getting faster at finding the right question to ask, doing a Google search, and understanding the answer I find - or get nudged in the right direction to eventually find the answer. Sometimes the problem I'm trying to solve is just way too over my head and I abandon the problem in favor of spending my time doing something else. For example, I can't yet wrap my head around d3.js. Intermediate and advanced topics of Java give me brain freeze. And I still can't remember how to write a http-server in node.js without reading a tutorial - after a dozen times.
We're all different, but from what I keep reading and hearing is that this is a never ending journey and no matter how many walls we break down, there will be more in our way further ahead - unless, we stop learning and just continue solving the same problem day after day.
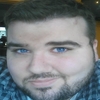
Marcus Parsons
15,719 PointsJust to add my own two cents here from my own personal experience, I've found that when I watch a video that goes over new things I'm not familiar with, I've found the best way for me to retain that information is to try to make some random, simple programs that use the information I was just taught. I'm a hands on learner, myself, so once I get my hands on something and start using it, I can figure out through trial and error exactly how they work. Then, you can go on to make more complicated programs using the practice you've gained earlier.
I did that method to come up with my paint program: http://marcusparsons.com/projects/sketchmeh. It started off with Andrew Chalkley's awesome course "Simple jQuery Drawing App" and then blossomed into a work I'm proud to show off.
Again, this is just my own personal opinion, and your learning style may be completely different. You just gotta find your groove and be groovy, my friend!

ibbhfsmeaf
4,568 PointsThank you for that enlightening comment. I've had many ideas in my head over the years and I always put them away because whenever I attempted them (starting with C++ idiotically) it started to become way over my head and I just gave up. Then I took a C++ class in college and became the teachers pet. Got a 98% on my final (the question I got wrong was on purpose as an inside joke). Although it wasn't extremely high level C++, when I first started even conditional statements were beyond my comprehension. It just goes to show how giving up is literally the only reason people fail, because if you try hard enough and long enough you will succeed at anything in life - and I have now applied this logic to programming. I wont stop until I realize all of my visions - or die in the process; and learning this is the first step.

ibbhfsmeaf
4,568 PointsThe paint tool looks sublime. Keep up the good work, and thanks for the advice. =)