Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial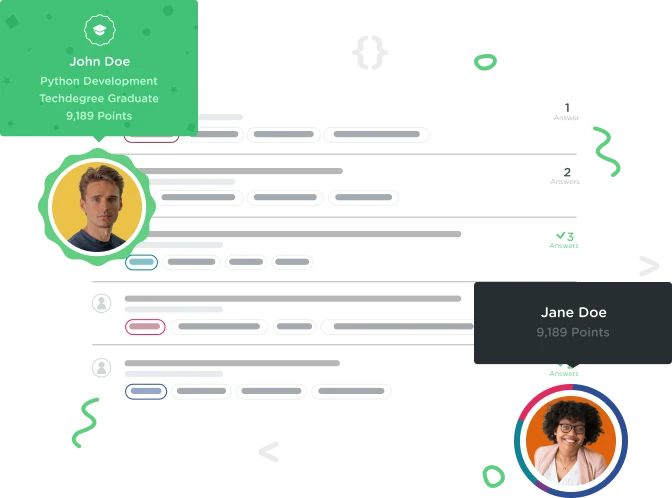

Sky Davis
665 PointsIs this the correct approach?
In my code, I created the questionGame within a if-statement that checks a boolean value. I wondering if this is the correct approach. If not, how can it be improved?
var questions = ["How do you spell cat?", "What is cat?", "CatDog?", "Cat Map?", "CatDoggieCat?"];
var answers = ["Cat","Cat","Cat","Cat","Cat"];
var equalQuestionsToAnswers = false;
var score = 0;
if(questions.length === answers.length) {
equalQuestionsToAnswers = true;
} else {
alert("THERE IS AN ERROR. QUESTIONS ARE NOT EQUAL TO ANSWERS");
}
if (equalQuestionsToAnswers) {
var questionGame = function() {
var userAnswerArr = [];
for( var i = 0; i <= questions.length - 1; i++ ) {
var userAnswers = prompt(questions[i]);
userAnswerArr.push(userAnswers);
}
for ( var j = 0; j <= userAnswerArr.length - 1; j++){
if(userAnswerArr[j] === answers[j]){
score += 1;
}else{
score;
}
}
alert(userAnswerArr);
alert("Your score is " + score);
if( score >= 4 ){
alert("You scored " + score + "Enjoy your gold crown.");
} else if( score >= 1){
alert("You win a silver crown. You got " + score + "correct");
} else if( score === 0 ){
alert("You win nothing.You got zero. Zeroooooooo");
}
}
questionGame();
}
2 Answers

Yuda Leh
7,618 PointsThe for loop and setting the var to false I found a little uneeded!
What you did was add a var score and a for loop, what you could have done was just have a var score = 0, in the if statments you can just added score++ (this increases the score by one)
This is what I did...
/*
5 triva Quiz Game: Programming Lanuages -
Created by Yehuda Lelah -(Fez)
UixNaN iX 1 (Develope Ver.1)
*/
// Counter Variable
var counter = 0;
// Languages used for Web Dev: Question One
var questionsOne = prompt("Name one language used to build a website?");
if ( questionsOne.toUpperCase() === "HTML" || questionsOne.toUpperCase() === "CSS" || questionsOne.toUpperCase() === "PHP" || questionsOne.toUpperCase() === "JAVASCRIPT" || questionsOne.toUpperCase() === "JS" || questionsOne.toUpperCase() === "JQUREY" ) {
alert("That's right!")
counter++
} else {
alert("Sorry, the answer is Html, Css, PHP, JavaScript or jQuery.");
}
// Languages used for iOS Dev: Question Two
var questionsTwo = prompt("Name one language used to make iOS Applications?");
if ( questionsTwo.toUpperCase() === "OBJECTIVE-C" || questionsTwo.toUpperCase() === "OBJECTIVE C" || questionsTwo.toUpperCase() === "OBJECTIVEC" || questionsTwo.toUpperCase() === "OBJ-C" || questionsTwo.toUpperCase() === "OBJC" || questionsTwo.toUpperCase() === "OBJ C" || questionsTwo.toUpperCase() === "SWIFT" ) {
alert("That's right!");
counter++
} else {
alert("Sorry, the answer is Objective-C or Swift.");
}
// Languages used for text bassed game: Ruby or Vim: Question Three
var questionsThree = prompt("What langauges is used for coding a text bassed game? Ruby or Vim?");
if ( questionsThree.toUpperCase() === "VIM" ) {
alert("That's right!");
counter++
} else {
alert("Sorry, the answer is Vim.");
}
// Language Google maps and Gmil build from: Question Four
var questionsFour = prompt("Name the language that G-main and Google Maps made from?");
if ( questionsFour.toUpperCase() === "JAVASCRIPT" || questionsFour.toUpperCase() === "JS" ) {
alert("That's right!");
counter++
} else {
alert("Sorry, the answer is JavaScript.");
}
// Languages used in Unity: Question Five
var questionsFive = prompt("Name a language that you can use to code games Unity?");
if ( questionsFive.toUpperCase() === "JAVASCRIPTT" || questionsFive.toUpperCase() === "JS" || questionsFive.toUpperCase() === "BOO" || questionsFive.toUpperCase() === "C#" || questionsFive.toUpperCase() === "CSHARP" || questionsFive.toUpperCase() === "C SHARP" ) {
alert("That's right!");
counter++
} else {
alert("Sorry, the answer is JavaScript, C#(CSharp) or Boo");
}
// How much player got correct
document.write("<p>You got " + counter + " / 5 programming questoins correct!</p>");
// Score
if ( counter === 5 ) {
document.write("<p>Since you got all 5 questions correct you are ranked <span style='color: #AEB404'>Legend Rank</span>!</p>");
} else if ( counter === 4 || counter === 3 ) {
document.write("<p>Sicne you got 4-3 questions correct you are ranked <span style='color: #04B404'>Master Rank</span>!</p>");
} else if ( counter === 2 || counter === 1 ) {
document.write("<p>Since you got 2-1 questions correct you are ranked <span style='color: #01A9DB'>Tyro Ranks</span>!</p>");
} else {
document.write("<p>You got none of the questions correct so, you don't get a rank :(!</p>");
}
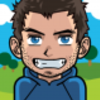
Daniel Bardaji Torres
15,266 PointsIf you don't want to "touch" a lot your code, you can add a little things like a validate user answers or try to do it with objects, I mean, question and answers can be together in a simple object. I think with that it will be easier to improve it.