Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial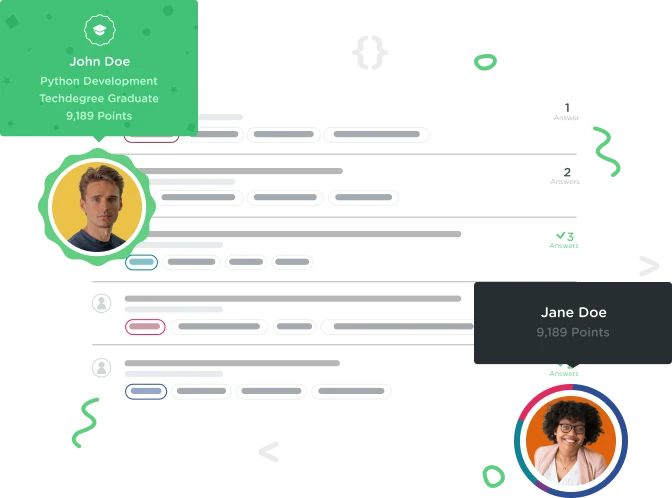

Fernando Paredes
15,425 PointsIsn't it simplier to put the condition inside the while() than using that flag?
I tried to do the exercise in advance to the teacher, and I made this:
var upper = 10
var randomNumber = getRandomNumber(upper);
var guess;
var guessCount = 0;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do{
guessCount += 1;
guess = prompt ('I am thinking of a number between 1 and ' + upper + '. What is it?');
}while( parseInt(guess) !== randomNumber)
document.write('It took you ' + guessCount + ' attempts to guess the number ' + randomNumber +'.');
As you can see, instead of using the flag variable, I just put the condition inside the while(). So I guess that using the flag var may be a kind of best practice or something like that. Is that right?
2 Answers

ibbhfsmeaf
4,568 PointsI did basically the same thing. There is no difference, really. I guess its just easier for other people to read the program with a flag and know what the do-while loop is doing, rather than having to look at misc. variables inside the condition and trying to find out what they are. But in either case, it's the same outcome.
That's my take on it.
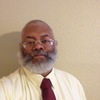
Adiv Abramson
6,919 PointsI was wondering the same thing, too. I think it's better programming style to simply compare the guessed number against the randomly generated number in the while () clause of the loop structure. That way you have one less variable to deal with, viz. the flag correctGuess.
To my mind, code inside the loop should cause the test condition to become false at some point, thus ending the loop, but should not actually include the test condition itself, which should be consigned to the while () clause.
Tibor Ruzinyi
17,968 PointsTibor Ruzinyi
17,968 PointsDoes it work for you ? Because ive tried the same as you for make it more easy, but i can not gues the number and when i close the prompt dialog the chrome freezes.