Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial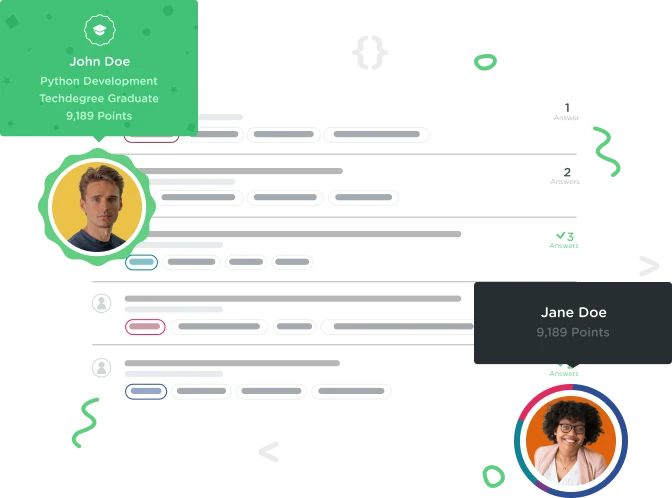
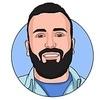
Eni Begotaraj
13,723 PointsIt doesn't show for me. Please help!!!
var students = [
{
name: 'Eni',
track : 'Front End Development',
achiviements: '158',
points: '14730'
},
{
name: 'Ardi',
track: 'iOS Development with Swift',
achiviements: '175',
points: '16375'
},
{
name: 'Irdi',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'Enea',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Zamir',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var message = '';
var student;
var search;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Achieviements: ' + student.achievements + '</p>';
report += '<p>Points: ' + student.points + '</p>';
return report;
}
while (true) {
search = prompt('Search student records: type a name [Ardi] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
}
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search ) {
message = getStudentReport(student);
print(message);
}
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Students</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>Students</h1>
<div id="output">
</div>
<script src="js/students.js"></script>
<script src="js/student_report.js"></script>
</body>
</html>
3 Answers
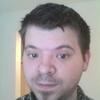
Aaron Loften
12,864 PointsYou have a typo in your object. You spell "achievements" a few different ways...
...
{
name: 'Ardi',
track: 'iOS Development with Swift',
achiviements: '175',
points: '16375'
},
{
name: 'Irdi',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
....
You then come back and ask for it spelled this way...
...
student.achievements
...
This one was tricky. The error message LOOKS like its saying "you couldnt find the element, bro!" but it actually means.... "So we found the element, but we cant write null to it." Which I thought it could, but I guess it cant. I mean...I guess LOGICALLY....you cant physically write nothing on a chalk board, because....you.....cant write nothing. ITs either nothing or something.
Get it? heh
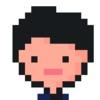
Owa Aquino
19,277 PointsI think you're missing the else statement on the while loop where you verify if the student name is in your list.
while (true) {
search = prompt('Search student records: type a name [Ardi] (or type "quit" to end)');
if (search === null || search.toLowerCase() === 'quit') {
break;
} else { //you forget about this
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name === search ) {
message = getStudentReport(student);
print(message);
}
}
}
}
Hope this help!
Cheers!
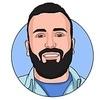
Eni Begotaraj
13,723 PointsUncaught TypeError: Cannot set property 'innerHTML' of null student_report.js:10
Thats what it shows on console.log. I rechecked and its the same code as it is on the video.
Eni Begotaraj
13,723 PointsEni Begotaraj
13,723 PointsThank you bro, It was very helpful. Sometimes its so annoying when you discover it's just a typo.
Aaron Loften
12,864 PointsAaron Loften
12,864 PointsI agree. I always say the scariest halloween costume for a developer would be the missing semi colon. lol