Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial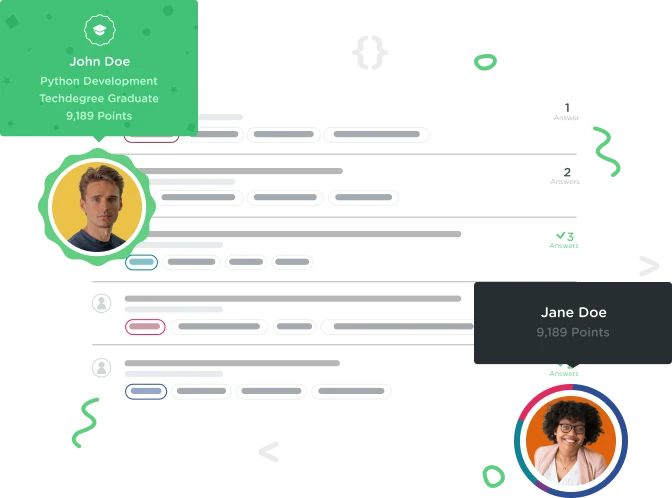

adensaid
4,461 PointsIt is telling that I have num isn't defined but I think there are couple other error in my program!
Here is my Code.
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start == random.randint(1, 99)
while True:
if even_odd(num) == 5:
print("{} is even!").format(num)
else:
print("{} is odd!").format(num)
start -= 1
3 Answers
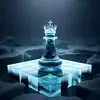
crosscheckking
25,155 PointsYou were close, but there were a few problems. Let's go through them one at a time. First you will want to store the value of random.randint in a different variable than start. This is because start is going to be used as a counter and we don't want to override it except to decrease it after each iteration of the while statement. Also, you will want to use just on equals sign because you want to assign the value to the variable (== means you are asking if they are equal). You will want to put this variable in the while statement so it changes after each iteration. Also, make sure the variable name is the same as the argument that you pass the even_odd and format functions because these functions will use this number. Also, these do not need to match the parameter that you used for even_odd (which you used as num). To clarify, in you code the num used in the while statement is a variable and it is not the same as the parameter num in the def function line. You can name the variables whatever you want. The important part is that they are passed to the appropriate function as an argument. Next, your while statement will need to be changed. It wants you to have it iterate as long as start is not 0. This means we can do while start and as long as start is any other number other than 0 it will keep iterating (we call these truthy values). Next you will want to take out the "=5" from your if statement. The even_odd function returns true if the random number it was passed was even, so we can do "if even_odd(num)" and it's block will run when the number is even. You also want to remove the ending parenthesis that is after the string literal "This is even". This is because format just goes right after the string literal. Lastly, you will need to get rid of the exclamation point in your string literal because the challenge is very specific and won't work if it is there (but it's not incorrect to have it there normally, just because it is checking for a specific string in this case). Hope this helps. The code is below.
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
num = random.randint(1, 99)
if even_odd(num):
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
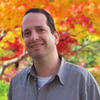
Russell Sawyer
Front End Web Development Techdegree Student 15,705 PointsYour code is pretty close.
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start: # <=== while start implies True until start is 0 in which case it becomes False and ends the loop
number = random.randint(1,99) #<==The random number needs to be inside the loop and should = a variable.
if number % 2 == 0: #<=== Capturing the remainder of the random number / 2 will tell you if the number is odd or even. If the remainder is 0 then it's even. If the remainder is anything other than 0 its odd.
print("{} is even".format(number)) #<=== The format needs to be inside the parentheses for the print()
else:
print("{} is odd".format(number))
start -= 1 #<=== The start -= 1 needs to be inside the while loop otherwise the loop will never end

David Bath
25,940 PointsYou have a few problems here (but I guess you knew that!). First, you define start at the beginning as 5, but then reuse that variable for a different value later. You should give the randomly generated number a different name, like num. However, that variable should be defined within the loop (read the instructions). The instructions also say to perform the loop until start is falsey (remember that 0 is considered falsey). So your while statement needs to reflect that:
while start:
Your IF statement also is testing the wrong thing. You want to use the even_odd function to find out if num is even. The function will return True if it's even, so you just need to test "if even_odd(num)".
Then, your decrement of start isn't inside the while loop, so the loop will run forever. Be careful, and read the instructions!