Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial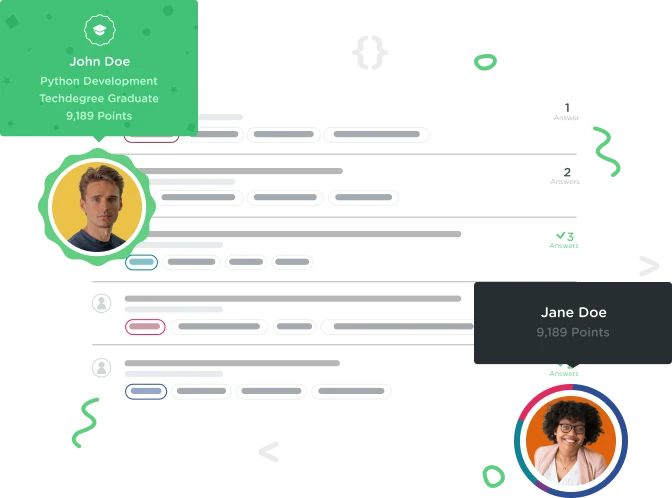

Gianpaul Rachiele
4,844 PointsIt keeps telling me there was a problem and won't submit my code. Is it because there is no break in the loop?
I can't tell if there is a problem with my code or a technical problem on the website's end. It keeps telling me there is a communication error.
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while True:
integer = random.randint(1, 99)
if even_odd(integer) == True:
print("{} is even".format(integer))
else:
print("{} is odd".format(integer))
start -= 1
3 Answers

Josh Keenan
20,315 PointsYou need to change your loop! It's infinite!
while start != 0:
False can mean 0 and it does in this instance!
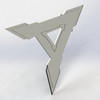
Afrid Mondal
6,255 PointsHey Gianpaul, Actually the challenge is asking you to continue the loop until the start is false. So, you have to check your condition against start.
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
start = 5
while start:
integer = random.randint(1, 99)
if even_odd(integer) == True:
print("{} is even".format(integer))
else:
print("{} is odd".format(integer))
start -= 1
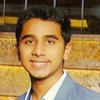
Anish Walawalkar
8,534 PointsHey you're doing great, you just made a couple of small mistakes:
- your while loop never ends become while True will never be false. you should change that to while start
- You don't need to check even_odd(integer) == True, you can just check even_odd(integer)
in code:
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
while start:
integer = random.randint(1, 99)
if even_odd(integer):
print("{} is even".format(integer))
else:
print("{} is odd".format(integer))
start = start - 1