Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial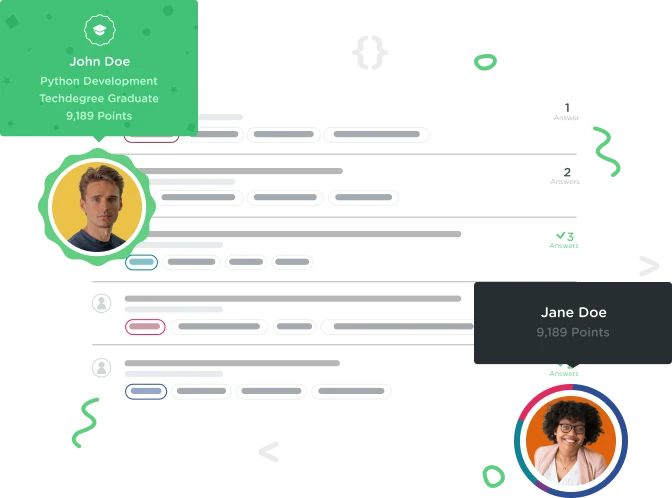

Timothy Smith
4,961 PointsIt prints the correct message, but it still tells me that the answer is wrong.
I've tried many different values for money, large and small. Maybe I just don't understand the concept of two comparison statements in the "if", and one in the "else if". Help please.
Thank you
var money = 9;
var today = 'Tuesday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
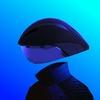
Kai Nightmode
37,045 PointsI find it helpful to read out logic statements to myself when programming. Usually in a slow, more proper english like way. For example, the following code...
if ( money >= 100 || today === 'Friday' ) {
...could be phrased as, "If I have 100 bucks or more OR it is Friday then do something." This sounds pretty understandable and if we take a look at another bit of code...
} else if ( money >= 50 || today === 'Friday' ) {
We could rephrase it as, "If I have 50 bucks or more OR it is Friday then do something." Here is where we can notice our issue. Both bits of logic only care about the money OR the fact that it may be Friday.
The fix is pretty easy, change the occurrences of || aka OR to && which means AND. For bonus points, rephrase the logic again to more of a english sentence and it should make a lot more sense. :D
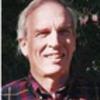
jcorum
71,830 PointsThey wanted you to fix the script, not change the values of the variables. There are several ways to fix it, but the editor is picky and seems to want only this one:
var money = 9;
var today = 'Friday'
if ( money >= 100 && today !== 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today !== 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today !== 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}