Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial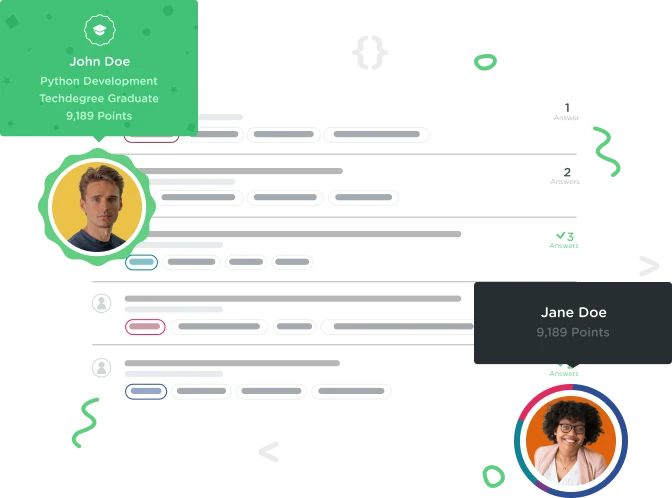

Stewart Yu
1,226 PointsIt was saying that my function returned the wrong teacher, even though that teacher was not in the example.
After hitting "Submit" a few times, it eventually accepted my solution.
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(teacher_dict):
max_count = 0
cur_max_teacher = ''
for teacher in teacher_dict:
if len(teacher_dict[teacher]) > max_count:
cur_max_teacher = teacher
return cur_max_teacher
most_classes({'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
'Kenneth Love': ['Python Basics', 'Python Collections']})
2 Answers
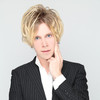
Mikael Enarsson
7,056 PointsInside your if statement, if the count for the current teacher is greater than that of the current max teacher, you also need to set the max_count
:
for teacher in teacher_dict:
if len(teacher_dict[teacher]) > max_count:
cur_max_teacher = teacher
max_count = len(teacher_dict[teacher]) #You also have to do this, otherwise any time
#a teacher has ANY courses cur_max_teacher
#will be set to that teacher
I hope this helps ^^
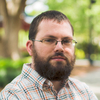
Kenneth Love
Treehouse Guest TeacherWell, example is just that, an example. If it was the actual input, it would be too easy to just return the top teacher after you figured it out yourself (not saying you would do it, but others might and have in the past).
And, trust me, you don't want to go down the path of me checking that your code matches an expected way of writing it. That's not very Pythonic and is way too much work for all of us, students and teachers.
Stewart Yu
1,226 PointsStewart Yu
1,226 PointsThanks! I ended up figuring it out later. :) It would be helpful to see the inputs that you are using to test the work. My solution was working with the example data in the comments, but it wasn't working with the data that was actually being used to test my solution.
Mikael Enarsson
7,056 PointsMikael Enarsson
7,056 PointsThe problem may be that dictionaries are unsorted, so if you make the dictionary
{'one': 1, 'two': 2}
, it may actually be represented as{'two': 2, 'one': 1}
. If you have a loop like the one you posted, the last item in the dictionary is the one that is stored incur_max_teacher
, and that can be any teacher, depending on how the dictionary is represented. Or something like that ^^Seeing the inputs and outputs in the challenges would be very helpful indeed.