Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial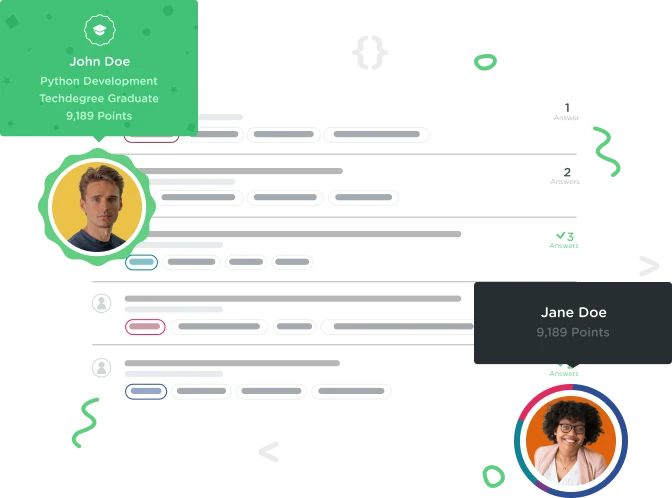

Travis Roby
7,793 Points"It's Friday, but I don't have enough money to go out"
var money = 9; var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 9 || today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
*** Need help getting past this one. ***
else if ( money < 9 || today === 'Friday' ) { alert("It's Friday, but I don't have enough money to go out");
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 9 || today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

Tabatha Trahan
21,422 PointsChange the logical OR operators to logical AND operators in the condition statements for the first three conditions. Because the variable today is set to Friday, and the first condition statement is joined by a logical or, it meets that condition and will execute the code within that block.

Travis Roby
7,793 PointsThank you so much
Dylan Glover
2,537 PointsDylan Glover
2,537 PointsIt seems like you have a few operator issues. if your money = 9 and you want to trigger the event, the code should say "money <= 9" for if money is less than or equal to 9. You could also say < 10. Also you are using the || operator for OR when I believe it should be the && for AND, as in you don't have the money for a movie and it is also Friday (this should probably also go for your other answers as well) .
So, I think it should look like this:
else if ( money <= 9 && today === 'Friday' ) { alert("It's Friday, but I don't have enough money to go out");