Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial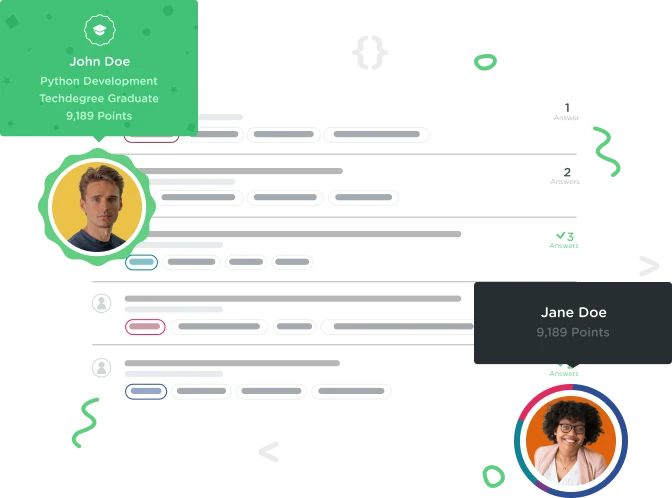

Justus Thompson
3,201 PointsIt's impossible for me to guess the correct answer and the loop won't stop. Can anyone help?
var randomNumber = getRandomNumber(10); var guess; var guessCount = 0; var correctGuess = false;
function getRandomNumber( upper ) { var num = Math.floor(Math.random() * upper) + 1; return num; }
do { guess = prompt("I am thinking of a number between 1 and 10. Can you guess the number?"); guessCount += 1; if (parseInt(guess) === randomNumber){ correctGuess === true; } } while ( ! correctGuess ) document.write ('<h1>You guessed the number!</h1>'); document.write ('It took you ' + guessCount + ' tries to guess the number ' + randomNumber);
2 Answers
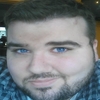
Marcus Parsons
15,719 PointsHey Justus Thompson,
The only problem with your code is that in your if statement in the do-while loop, you were using the === comparison operator to try to assign a value to correctGuess
. Any time you are assigning a value to a variable you must always use the single = sign. == or === are reserved for comparisons only!
Here is the fixed code! :)
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt("I am thinking of a number between 1 and 10. Can you guess the number?");
guessCount += 1;
if (parseInt(guess) === randomNumber){
//Changed to assignment operator, single = sign
correctGuess = true;
}
} while ( ! correctGuess )
document.write ('You guessed the number!');
document.write ('It took you ' + guessCount + ' tries to guess the number ' + randomNumber);
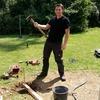
Nejc Vukovic
Full Stack JavaScript Techdegree Graduate 51,574 PointsOne way is this:
JSFIddle Link <- This is just an uprgrade to your code check the answer below.
The problem your code doesn't stop is this line:" correctGuess === true; "
This means you are comparing the correctGuess to boolean value. Thats why your loop doesn't end.
You have to set the variable correctGuess to true, this looks like this:" correctGuess = true; "
Try this and I hope it helps.
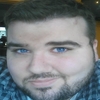
Marcus Parsons
15,719 PointsNejc, I literally said that above so what is the purpose in answering a question with the same answer that's already been answered? lol Btw, changing the "document.write" to "alert" is not an upgrade of any kind. lol
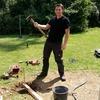
Nejc Vukovic
Full Stack JavaScript Techdegree Graduate 51,574 PointsWell the jsfiddle complains about the document.write method if you don't know. And I'm on a SPS course and typing as much as I can. Took me longer to write and didn't refresh the page every 2secs.
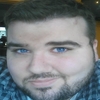
Marcus Parsons
15,719 PointsIt's an example, so using document.write is fine. This code isn't going to be used by thousands of people on a day to day basis so I think we can let Justus get away with using document.write in his example. What do you say? lol
Justus Thompson
3,201 PointsJustus Thompson
3,201 PointsThis code should match the teachers code in the video.