Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial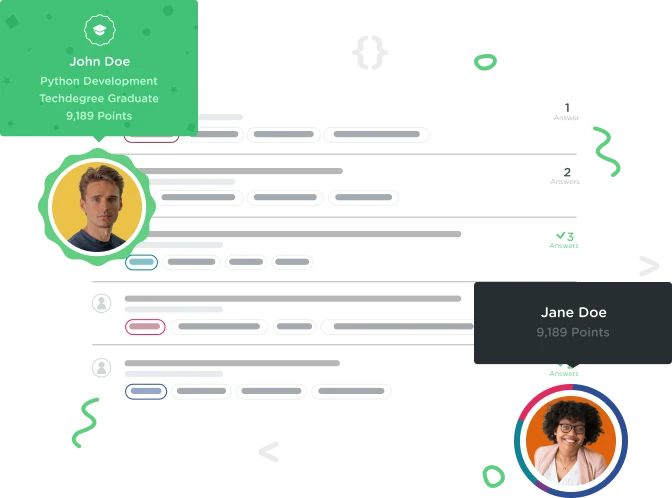
Ahmed Hassan
5,533 PointsI've completed the quiz challenge. Please review and feed back.
/*
This is my attempt to make a program to do the following:
1- Create a 5 questions quiz.
2- Keep track of player's correct answers.
3- Print a final message telling the player how many correct answer did he/she got.
4- Rank the player.
*/
//Player Name
var PlayerName=prompt("Please provide your name below to start the quiz.");
document.write("Hello "+PlayerName+"!");
//Correct Answers Counter
var CorrectAnswer=0;
//Remaining Questions Counter
var RemainQuestion=4;
//Questions
document.write("<p>Please read and answer the following questions</p>");
var question_1=prompt("Question 1: What is the name of U.S capital? | Remaining Questions: "+RemainQuestion);
if(question_1.toLowerCase()==="washington dc" || question_1.toLowerCase()==="washington") {
document.write("<p>Question 1: Correct Answer</p>");
CorrectAnswer+=1;
} else {
document.write("<p>Question 1: Wrong Answer!</p>");
}
RemainQuestion-=1;
var question_2=prompt("Question 2: Who is teaching JavaScript Basics on Tree House? | Remaining Questions: "+RemainQuestion);
if(question_2.toLowerCase()==="dave" || question_2.toLowerCase()==="dave mcfarland") {
document.write("<p>Question 2: Correct Answer</p>");
CorrectAnswer+=1;
} else {
document.write("<p>Question 2: Wrong Answer!</p>");
}
RemainQuestion-=1;
var question_3=prompt("Question 3: Who is the former CEO of Appl Inc.? | Remaining Questions: "+RemainQuestion);
if(question_3.toLowerCase()==="steve" || question_3.toLowerCase()==="steve jobs") {
document.write("<p>Question 3: Correct Answer</p>");
CorrectAnswer+=1;
} else {
document.write("<p>Question 3: Wrong Answer!</p>");
}
RemainQuestion-=1;
var question_4=prompt("Question 4: Who invented the electric lamp? | Remaining Questions: "+RemainQuestion);
if(question_4.toLowerCase()==="thomas edison" || question_4.toLowerCase()==="edison") {
document.write("<p>Question 4: Correct Answer</p>");
CorrectAnswer+=1;
} else {
document.write("<p>Question 4: Wrong Answer!</p>");
}
RemainQuestion-=1;
var question_5=prompt("Question 5: Who invented the famous equation E=MC^2 that describes relativity? | Remaining Questions: "+RemainQuestion);
if(question_5.toLowerCase()==="albert einstein" || question_5.toLowerCase()==="einstein") {
document.write("<p>Question 5: Correct Answer</p>");
CorrectAnswer+=1;
} else {
document.write("<p>Question 5: Wrong Answer!</p>");
}
alert("You have answered "+CorrectAnswer+" questions correctly");
if (CorrectAnswer===5) {
document.write("You've got "+CorrectAnswer+" correct answers out of 5, you are ranked: Golen Crown");
}
if (CorrectAnswer===4 || CorrectAnswer===3) {
document.write("You've got "+CorrectAnswer+" correct answers out of 5, you are ranked: Silver Crown");
}
if (CorrectAnswer===2 || CorrectAnswer===1) {
document.write("You've got "+CorrectAnswer+" correct answers out of 5, you are ranked: Bronze Crown");
}
if (CorrectAnswer===0) {
document.write("You've got "+CorrectAnswer+" correct answers out of 5, you are ranked: No Crown");
}

Jacob Mishkin
23,118 Pointsjust review the markdown cheatsheet for code use
Code
Wrap your code with 3 backticks () on the line before and after. If you specify the language after the first set of backticks, that'll help us with syntax highlighting.
html
<p>This is code!</p>
```
2 Answers
Ahmed Hassan
5,533 PointsYeah the code is too much, but u know.. I tried with the knowledge I have. Im sure it will be much shorter when I learn how to loop and make usable functions.
Thanks Daniel.

Daniel Newman
Courses Plus Student 10,715 PointsHere the script you've provided. It's nice and messy because you did not use the next chapter of your learning js: loops and function. When you get learned about it the whole code become much shorter and "expressive". Wish you happy learning the next chapters of your js course, Ahmed!
/* This is my attempt to make a program to do the following: 1- Create a 5 questions quiz. 2- Keep track of player's correct answers. 3- Print a final message telling the player how many correct answer did he/she got. 4- Rank the player. */
//Player Name var PlayerName=prompt("Please provide your name below to start the quiz."); document.write("Hello "+PlayerName+"!");
//Correct Answers Counter var CorrectAnswer=0;
//Remaining Questions Counter var RemainQuestion=4;
//Questions document.write("Please read and answer the following questions");
var question_1 = prompt("Question 1: What is the name of U.S capital? | Remaining Questions: " + RemainQuestion);
if (question_1.toLowerCase() === "washington dc" || question_1.toLowerCase() === "washington") {
document.write("Question 1: Correct Answer");
CorrectAnswer += 1;
} else {
document.write("Question 1: Wrong Answer!");
}
RemainQuestion -= 1;
var question_2 = prompt("Question 2: Who is teaching JavaScript Basics on Tree House? | Remaining Questions: " + RemainQuestion);
if (question_2.toLowerCase() === "dave" || question_2.toLowerCase() === "dave mcfarland") {
document.write("Question 2: Correct Answer");
CorrectAnswer += 1;
} else {
document.write("Question 2: Wrong Answer!");
}
RemainQuestion -= 1;
var question_3 = prompt("Question 3: Who is the former CEO of Appl Inc.? | Remaining Questions: " + RemainQuestion);
if (question_3.toLowerCase() === "steve" || question_3.toLowerCase() === "steve jobs") {
document.write("Question 3: Correct Answer");
CorrectAnswer += 1;
} else {
document.write("Question 3: Wrong Answer!");
}
RemainQuestion -= 1;
var question_4 = prompt("Question 4: Who invented the electric lamp? | Remaining Questions: " + RemainQuestion);
if (question_4.toLowerCase() === "thomas edison" || question_4.toLowerCase() === "edison") {
document.write("Question 4: Correct Answer");
CorrectAnswer += 1;
} else {
document.write("Question 4: Wrong Answer!");
}
RemainQuestion -= 1;
var question_5 = prompt("Question 5: Who invented the famous equation E=MC^2 that describes relativity? | Remaining Questions: " + RemainQuestion);
if (question_5.toLowerCase() === "albert einstein" || question_5.toLowerCase() === "einstein") {
document.write("Question 5: Correct Answer");
CorrectAnswer += 1;
} else {
document.write("Question 5: Wrong Answer!");
}
alert("You have answered " + CorrectAnswer + " questions correctly");
if (CorrectAnswer === 5) {
document.write("You've got " + CorrectAnswer + " correct answers out of 5, you are ranked: Golen Crown");
}
if (CorrectAnswer === 4 || CorrectAnswer === 3) {
document.write("You've got " + CorrectAnswer + " correct answers out of 5, you are ranked: Silver Crown");
}
if (CorrectAnswer === 2 || CorrectAnswer === 1) {
document.write("You've got " + CorrectAnswer + " correct answers out of 5, you are ranked: Bronze Crown");
}
if (CorrectAnswer === 0) {
document.write("You've got " + CorrectAnswer + " correct answers out of 5, you are ranked: No Crown");
}
Ahmed Hassan
5,533 PointsAhmed Hassan
5,533 PointsGuys I want to know how to attach the workspace.. because when I copy and past my written code, I get the above mess.
Thanks