Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial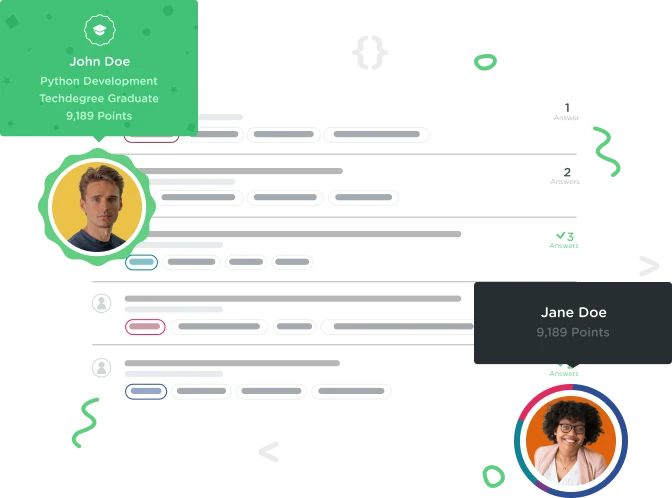

Rachael A
1,044 PointsI've tested this and it works but it doesn't pass the challenge
I've tested this in sublime with spam = "I do not like it Sam I Am" and I get this as my result: {'i': 2, 'do': 1, 'not': 1, 'like': 1, 'it': 1, 'sam': 1, 'am': 1} so why doesn't this work?
def word_count(spam):
unique = []
word_dictionary = {}
count = 1
spam = spam.lower().split()
for word in spam:
if word not in unique:
unique.append(word)
count = 1
word_dictionary[word] = count
else:
count += 1
word_dictionary[word] = count
return(word_dictionary)
2 Answers
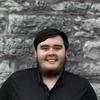
Michael Hulet
47,912 PointsWhile I personally encourage you to test in an external REPL as it can help you test and understand your code before you submit it, I do have to say that Steven's example exposes your problem fairly well. He's right that no word appears more than twice. However, when I test your function with his example, this is the output I get:
>>> word_count("What is love Baby don't hurt me Don't hurt me no more")
{'what': 1, 'is': 1, 'love': 1, 'baby': 1, "don't": 2, 'hurt': 3, 'me': 4, 'no': 1, 'more': 1}
You'll notice that 'hurt'
is 3
and 'me'
is 4
. That's not correct, because as Steven and I said before, no word appears more than twice. In your code, the only place that a number is set in word_dictionary
is from your variable count
, so this means that your variable count
is getting out of sync with reality.
In this case, it's a scoping issue. Before the loop starts, you set count
to 1
. It's important to note that this was declared before the loop began, and thus it exists and persists with the same value for each iteration of the loop without being reset by Python. In your code, when the loop encounters a word that doesn't exist as a key in the word_dictionary
yet, it resets count
to 1
, which is fine. However , because count
holds its value between iterations, this can be a problem when the loop encounters a few words in a row that are already in word_dictionary
.
Let's jump ahead to the 8th iteration of the loop. Right now, the word we're on from the original sentence is "Don't"
, but it's been lowercased earlier in the function. This is what word_dictionary
looks like:
{'what': 1, 'is': 1, 'love': 1, 'baby': 1, "don't": 1, 'hurt': 1, 'me': 1}
Up to this point, every word we've encountered has been unique, so the count
variable has been reset to 1
every time. However, now that we're on "don't
", we've seen this word before, so we go onto the else
block. 1
is added to count
, so count
is now 2
. This is a correct final value, but as you'll see in a moment, that's coincidental. word_dictionary["don't"]
is set to count
(which, again, is 2
at this point), and we continue onto the next iteration of the loop
At this iteration, the word we're on is the 2nd "hurt
" in the sentence. Since it's outside the loop, count
has hung onto its value that it's had before, which is still 2
at this point. We've seen this word before, so we go onto the else
block again. We add 1
to count
so that it's 3
now, and we assign that to word_dictionary["hurt"]
.
The same thing happens to "me
" on the next iteration. count
is 3
when the loop starts, and when the else
block runs, count
is incremented once more, so word_dictionary["me"]
is set to 4
.
I know I've been stressing throughout this answer that the fact that count
is declared outside the loop, but this was just to illustrate why it goes too high on certain words. Ultimately, placing it inside the loop isn't a very good solution, either, because then its value will always be either 1
or 2
depending on if the word was encountered before, and won't ever go higher, even if the word is found 3 or more times. Instead of holding onto an external count
variable, I recommend checking the value in the dictionary on each iteration of the loop and setting it to 1
if the key doesn't exist yet or increment it if it does
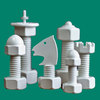
Steven Parker
231,007 Points Be careful about testing a challenge in an external REPL — it's very easy to misinterpret the results.
To illustrate the issue, try testing your code with this input string: "What is love Baby don't hurt me Don't hurt me no more
". You'll notice no word is used more than twice ... but what does your function say?
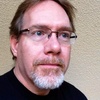
Chris Freeman
Treehouse Moderator 68,426 PointsWhy was this down voted?
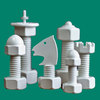
Steven Parker
231,007 PointsI may have become a target of the radical spoilerists.
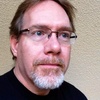
Chris Freeman
Treehouse Moderator 68,426 PointsUpvoting to get back to 0!