Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial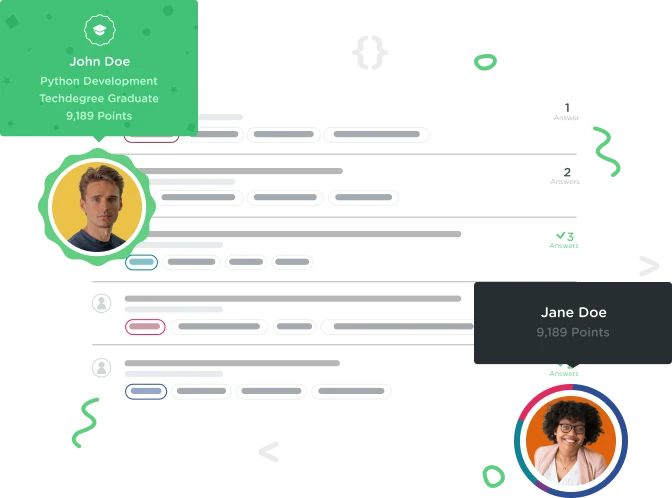

Sven Huff
Courses Plus Student 4,355 PointsJS: Issues with else if and logical operator
Hi,
I've used the || operator, but it seems, that there is another mistake too. But I can't figure out which one.
Thanks for your help.
Cheers,
Sven
var money = 9;
var today = 'Friday';
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 10 || today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers

Ryan Duchene
Courses Plus Student 46,022 PointsThis challenge is a bit poorly worded IMO, so I'll give you the answer and we'll walk through it together.
So, this is the code you start out with:
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
The challenge asks us to make sure that the alert we get says "It's Friday, but I don't have enough money to go out." So, let's take a look at the code to see which if/else clause will output that code. After some examination, we see that it's the fourth clause.
In order to activate the fourth clause, we need to pass its test, which requires that the today
variable be unequal to 'Friday'
. Well, the today
variable obviously doesn't pass, so we need to change the conditional so that it accepts today
when it's equal to 'Friday'
.
So here's what that change looks like:
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Okay, this should pass now, right? Nope, the first clause is still firing off its alert. Why is that? Well, let's take a took at its conditional and write it out in plain English: "If my money is at least 100, OR today is Friday, then I can go to the theater."
Hmm...that doesn't sound right. It should be "If my money is at least 100, AND today is Friday, then I can go to the theater."
And, if we look down at the following clauses, we can see that they all have the exact same problem as well. So we need to change ||
to &&
everywhere.
Now it should pass. And it does!
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
If any part of my explanation was fuzzy, just let me know and I'll try to smooth it out.
Hope that helps! Happy coding!
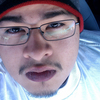
Chyno Deluxe
16,936 PointsYou're conditional statement is asking if money is greater than or equal to 100 ||(OR) if today is friday. since today is Friday that is why your alert is firing.
You should be asking if money >= 100 &&(AND) today is Friday instead. View Below
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today == 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}

Sven Huff
Courses Plus Student 4,355 PointsThank you so much Ryan and Chyno! Your answers were very insightfull for me.

Ryan Duchene
Courses Plus Student 46,022 PointsGlad I could help!