Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial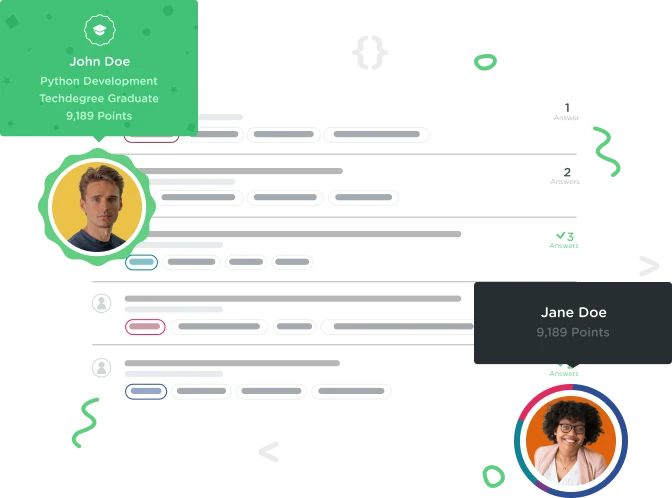

Eric Denton
4,552 PointsJust sharing my solution. It seems like there's a lot of room for creativity!
That was rather fun!
7 Answers

Eric Denton
4,552 PointsMight not be the most concise, but made me feel accomplished!
var answer1 = 60; // minutes in an hour
var answer2 = 24; // hours in a day
var answer3 = 7; // days in a week
var answer4 = 52; // weeks in a year
var answer5 = 10; // years in a decade
var score = 0; // user score
//Question set 1
var guess1 = parseInt(prompt("How many minutes in an hour?"));
if (guess1 === answer1) {
score += 1; // rather than score = score + 1
alert("That's right! Your current score = " + score);
} else {
alert("Sorry Charlie! Your current score = " + score);
}
//Question set 2
var guess2 = parseInt(prompt("How many hours in a day?"));
if (guess2 === answer2) {
score += 1; // rather than score = score + 1
alert("Nailed it! Your current score = " + score);
} else {
alert("DOH! Your current score = " + score);
}
//Question set 3
var guess3 = parseInt(prompt("How many days in an week?"));
if (guess3 === answer3) {
score += 1; // rather than score = score + 1
alert("Good job! Your current score = " + score);
} else {
alert("Ohhh they let you out in public? Your current score = " + score);
}
//Question set 4
var guess4 = parseInt(prompt("How many weeks in an year?"));
if (guess4 === answer4) {
score += 1; // rather than score = score + 1
alert("Check out Einstein over here! Your current score = " + score);
} else {
alert("HASHTAG FAIL! Your current score = " + score);
}
//Question set 5
var guess5 = parseInt(prompt("How many years in an decade?"));
if (guess5 === answer5) {
score += 1; // rather than score = score + 1
alert("MENSA MATERIAL! Your current score = " + score);
} else {
alert("Bless your heart... Your current score = " + score);
}
//Final Score is...
document.write("Your score is a whopping " + score + "! Way to go?! ");
//Medal Ceremony
if (score === 5) {
document.write ("You get a GOLD MEDAL!");
} else if (score === 3 || score === 4) {
document.write ("You get a SILVER MEDAL!");
} else if (score === 1 || score === 2) {
document.write ("You get a Bronze MEDAL!");
} else {
document.write ("You get a participation sticker!");
}
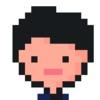
Owa Aquino
19,277 PointsLove the alert messages.. haha and the "You get a participation sticker!" message.
haha. Great job mate!

wouterb
1,307 PointsHere is mine:
/*
Quiz:
ask 5 questions
2. keep track of correctly answered questions
3. final message after quiz with number of correct answers
4. rank player
a. all 5 correct = gold crown
b. 3/4 correct = silver crown
c. 1/2 correct = bronze crown
d. 0 correct = no crown
*/
//Question 1:
var correctAnswer = 1+1;
var score = 0;
var answer = parseInt(prompt('What is 1+1?'));
if (answer === correctAnswer) {
alert('Answer is correct!');
score += 1;
} else {
alert('Answer is wrong!');
}
console.log('Score: ' + score);
//Question 2:
correctAnswer = 8-2;
answer = parseInt(prompt('What is 8 - 2?'));
if (answer === correctAnswer) {
alert('Answer is correct!');
score += 1;
} else {
alert('Answer is wrong!');
}
console.log('Score: ' + score);
//Question 3:
correctAnswer = 22*3;
answer = parseInt(prompt('What is 22 * 3?'));
if (answer === correctAnswer) {
alert('Answer is correct!');
score += 1;
} else {
alert('Answer is wrong!');
}
console.log('Score: ' + score);
//Question 4:
correctAnswer = 80/4;
answer = parseInt(prompt('What is 80 / 4?'));
if (answer === correctAnswer) {
alert('Answer is correct!');
score += 1;
} else {
alert('Answer is wrong!');
}
console.log('Score: ' + score);
//Question 5:
correctAnswer = 12+(3*3);
answer = parseInt(prompt('What is 12 + (3 * 3)?'));
if (answer === correctAnswer) {
alert('Answer is correct!');
score += 1;
} else {
alert('Answer is wrong!');
}
console.log('Score: ' + score);
//Rank:
var rank = 'no crown';
if (score === 1 || score === 2){
rank = 'Bronze crown';
} else if (score === 3 || score === 4) {
rank = 'Silver crown';
} else if (score === 5) {
rank = 'Gold crown';
}
if (rank === 'no crown') {
document.write('Your score = ' + score + '. You won ' + rank + '!');
} else {
document.write('Your score = ' + score + '. You won the ' + rank + '!');
}
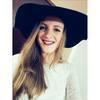
Sonja Tomcic
6,590 Points//5-question math quiz
var welcome = alert("Welcome to the quiz. Please answer 5 questions");
var questions = 5
//question 1. result=8
var question1 = prompt("Question 1: Add number 5 to number 3. What's the result?");
if(parseInt(question1) === 8 ) {
var questionsCorrect = 1;
alert("Your result is correct. You answered " + questionsCorrect + " question correctly.");
}
else {
var questionsCorrect = 0;
alert("Your result isn't correct. You answered " + questionsCorrect + " question correctly.");
}
//question 2. result=5
var question2 = prompt("Question 2: Subtract 5 from 10. What's the result?");
if(parseInt(question2) === 5) {
questionsCorrect += 1;
alert("Your result is correct. You answered " + questionsCorrect + " question correctly.");
}
else {
alert("Your result isn't correct. You answered " + questionsCorrect + " question correctly.");
}
//question 3. result=72
var question3 = prompt("Question 3: Multiply 9 and 8. What's the result?");
if(parseInt(question3) === 72) {
questionsCorrect += 1;
alert("Your result is correct. You answered " + questionsCorrect + " question correctly.");
}
else {
alert("Your result isn't correct. You answered " + questionsCorrect + " question correctly.");
}
//question 4. result=10
var question4 = prompt("Question 4: Divide 72 with 9 and add number 2. What's the result?");
if(parseInt(question4) === 10) {
questionsCorrect += 1;
alert("Your result is correct. You answered " + questionsCorrect + " question correctly.");
}
else {
alert("Your result isn't correct. You answered " + questionsCorrect + " question correctly.");
}
//question 5. result=50
var question5 = prompt("Question5: Multiply 7 and 7 and add 1. What's the result?");
if(parseInt(question5) === 50) {
questionsCorrect +=1;
alert("Your result is correct. You answered " + questionsCorrect + " question correctly.");
}
else {
alert("Your result isn't correct. You answered " + questionsCorrect + " question correctly.");
}
//final message and ranking
document.write("You answered " + questionsCorrect + " questions correctly. ");
if(questionsCorrect === 5) {
document.write("Congratulations, you are awarded with gold crown.")
}
else if(questionsCorrect===3 || questionsCorrect===4) {
document.write("Congratulations, you are awarded with silver crown.");
}
else if(questionsCorrect===1 || questionsCorrect===2) {
document.write("Congratulatons, you are awarded with bronze crown. ");
}
else {
document.write("You are not awarded with crown, unfortunately.");
} ```
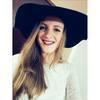
Sonja Tomcic
6,590 PointsHow can I send my code like this? I only know how to share link to my workspace?
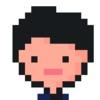
Owa Aquino
19,277 PointsHi Sonja,
You can display it to the comment by adding this ``` before and after your codes.
Cheers!
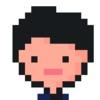
Owa Aquino
19,277 PointsHere's mine!
/*
To do:
1. Ask at least five questions - DONE
2. Keep track of the number of questions the user answered correctly - DONE
3. Provide a final message after the quiz letting the user know
the number of questions he or she got right. - DONE
4. Rank the player. If the player answered all five correctly,
give that player the gold crown: 3-4 is a silver crown; 1-2 correct answers
is a bronze crown and 0 correct is no crown at all. - DONE
*/
var answer1 = 'Mark';
var answer2 = 'Steve Jobs';
var answer3 = 'Van Schienider';
var answer4 = 'Austin Kleon';
var answer5 = 'Tesla';
var correctAnswer = 0;
// QUestion no. 1
var question1 = prompt('First name of founder of Facebook?');
if(answer1.toUpperCase() === question1.toUpperCase()){
correctAnswer += 1;
}
// Question no. 2
var question2 = prompt('Who invented the iPhone?');
if(answer2.toUpperCase() === question2.toUpperCase()){
correctAnswer += 1
}
// Question no. 3
var question3 = prompt('Who was the lead designer of Spotify?');
if(answer3.toUpperCase() === question3.toUpperCase()){
correctAnswer += 1
}
console.log('Correct answer : ' + correctAnswer);
// Question no. 4
var question4 = prompt('Author of Steal Like an Artist?');
if(answer4.toUpperCase() === question4.toUpperCase()){
correctAnswer += 1
}
// Question no. 5
var question5 = prompt('Created the electricity?');
if(answer5.toUpperCase() === question5.toUpperCase()){
correctAnswer += 1
}
//Final Message
var congratsMessage = document.write('Your total score is : ' + correctAnswer + '</br>');
//Ranking
if (correctAnswer === 5 ){
document.write('You earned GOLD Crown for answering 5 consecutive correct answers!');
console.log('Gold Crowns!');
}else if(correctAnswer > 2 && correctAnswer < 5){
document.write('You earned SILVER Crown for answering 3-4 consecutive correct answers!');
console.log('Silver Crowns!');
}else if(correctAnswer > 0 && correctAnswer < 3){
document.write('You earned BRONZE Crown for answering 1-2 consecutive correct answers!');
console.log('Bronze Crowns!');
}else{
document.write("Sorry but you didn't got any correct answer.");
console.log('No Crowns!');
}
console.log('End of quiz.');
Cheers!
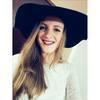
Sonja Tomcic
6,590 PointsOwa Aquino Thanks!

Keziyah Lewis
3,134 PointsIt's so great to see the different ways of doing this. Here's mine.
var score = 0;
alert("Let's see if you know your country capitals. The first one is easy, but it gets harder. Remember, spelling counts! Ready?");
/* Prompt: What's the capital of ______?
If user enters correct answer, "Yay. Your score is ___."
->If not, a prompt appears with the first letter of the city
as a clue.
->If second try is correct, alert("Your score is ___")
->If second try is wrong, "Sorry. The answer is ____." */
/*Question 1*/
var paris = prompt("What's the capital of France?");
if(paris.toUpperCase() === "PARIS") {
score +=1
alert("Yay! Your score is " + score);
} else if (paris.toUpperCase() !== "PARIS") {
var paris2 = prompt("Try again. It starts with a P.");
if (paris2.toUpperCase() === "PARIS") {
score +=1
alert("Good. Your score is " + score);
} else {
alert("Sorry. The answer is Paris.");
}
}
/*Question 2*/
var canberra = prompt("What's the capital of Australia?");
if(canberra.toUpperCase() === "CANBERRA") {
score +=1
alert("Yay! Your score is " + score);
} else if (canberra.toUpperCase() !== "CANBERRA") {
var canberra2 = prompt("Try again. It starts with a C.");
if (canberra2.toUpperCase() === "CANBERRA") {
score +=1
alert("Good. Your score is " + score);
} else {
alert("Sorry. The answer is Canberra.");
}
}
/*Question 3*/
var riyadh = prompt("What's the capital of Saudi Arabia?");
if(riyadh.toUpperCase() === "RIYADH") {
score +=1
alert("Yay! Your score is " + score);
} else if (riyadh.toUpperCase() !== "RIYADH") {
var riyadh2 = prompt("Try again. It starts with an R.");
if (riyadh2.toUpperCase() === "RIYADH") {
score +=1
alert("Good. Your score is " + score);
} else {
alert("Sorry. The answer is Riyadh.");
}
}
/*Question 4 */
var santiago = prompt("What's the capital of Chile?");
if(santiago.toUpperCase() === "SANTIAGO") {
score +=1
alert("Yay! Your score is " + score);
} else if (santiago.toUpperCase() !== "SANTIAGO") {
var santiago2 = prompt("Try again. It starts with an S.");
if (santiago2.toUpperCase() === "SANTIAGO") {
score +=1
alert("Good. Your score is " + score);
} else {
alert("Sorry. The answer is Santiago.");
}
}
/*Question 5 */
var nairobi = prompt("What's the capital of Kenya?");
if(nairobi.toUpperCase() === "NAIROBI") {
score +=1
alert("Yay! Your score is " + score);
} else if (nairobi.toUpperCase() !== "NAIROBI") {
var nairobi2 = prompt("Try again. It starts with an N.");
if (nairobi2.toUpperCase() === "NAIROBI") {
score +=1
alert("Good. Your score is " + score);
} else {
alert("Sorry. The answer is Nairobi.");
}
}
/*Telling the player what their score is and what crown they get.*/
document.write("<h1>You answered " + score + " questions right.</h1>");
if (score === 5) {
document.write("<h1>Congrats! You got a gold crown!</h1>");
} else if (score === 3 || 4) {
document.write("<h1>Good job. Silver crown for you.</h1>");
}
else if (score === 1 || 2) {
document.write("<h1>Ehh. Could have been better. Have a bronze crown.</h1>");
}
else {
document.write("<h1>Wow, really? You need to study your capitals more.</h1>");
}
```
Dante Alston
988 PointsDante Alston
988 PointsSo many interesting approaches... here's mine.