Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial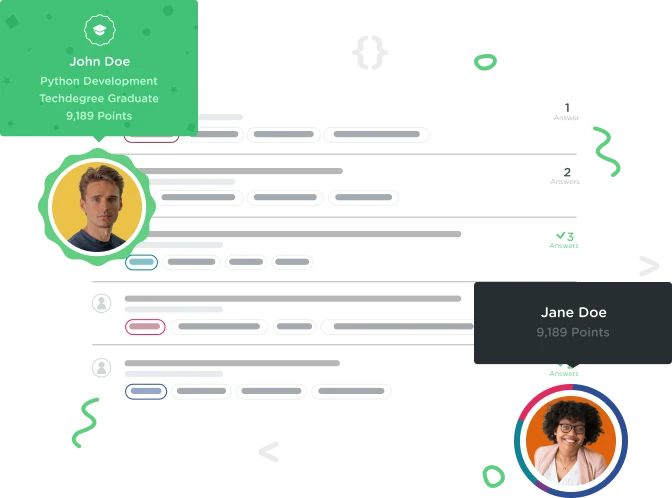

Michael Gardiner
4,282 PointsLocation of problem?
Not seeing the issue here
var money = 0;
var today = 'Thursday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
3 Answers
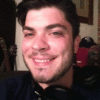
Sean Ford
9,581 PointsAlso, you should be using &&, which is basically AND, instead of ||, which means OR. Also, you used !== towards the end, but you should have used ===, since you are checking to see if today is Friday, according to the alert that will be thrown if the condition is met. Your code should look like this:
var money = 0; var today = 'Thursday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
// if you have 100 money or more, and today is Friday, then throw the alert.
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
// if you have 50 money or more (but not 100 or more), and today is Friday, throw this alert
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
//if you have more than 10 money (but not 50 or more), and today is Friday, throw this alert
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
// if today is Friday, but you don't have enough money to meet any of the above conditions, then throw this alert
} else {
alert("This isn't Friday. I need to stay home.");
// if today is not Friday, then throw this last alert
hope this helps!

Ben Myhre
28,726 PointsI am not sure what the issue actually is, but there seems to be a logic problem
else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
}
This conditional is evaluating to true because it is var today = 'Thursday' AND ( today !== 'Friday' ) , but then in the alert... it seems to indicate that this part of the conditional is supposed to be if it IS friday.

Michael Gardiner
4,282 Pointsso... it's impossible? lol
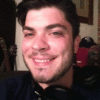
Sean Ford
9,581 PointsTry putting a semicolon after declaring your today variable, which would look like this:
var money = 0; var today = 'Thursday';
Michael Gardiner
4,282 PointsMichael Gardiner
4,282 Pointsgot it, thanks!
Ben Myhre
28,726 PointsBen Myhre
28,726 Pointsthis is the full on best answer imo.