Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial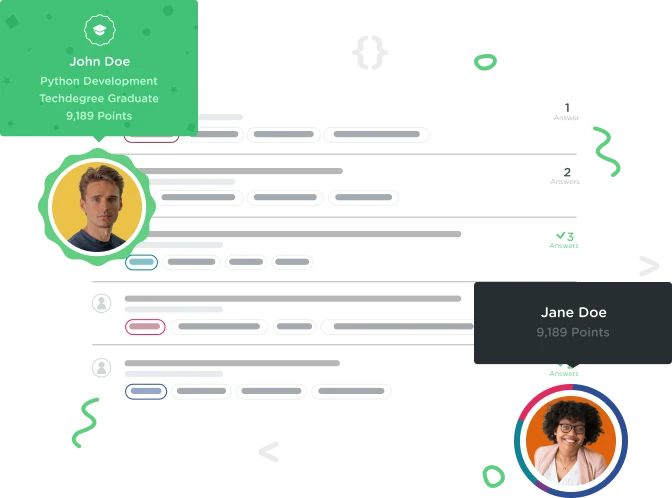

Matt Bloomer
10,608 Pointslogging property values
What am I doing wrong?
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for (var prop in shanghai)
{
console.log(prop, ':', shanghai[
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'];
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Objects</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
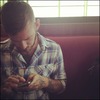
Erik McClintock
45,783 PointsMatt,
Iterating through objects with for...in
loops can be tricky at first. Let's walk through it.
We have our object, "shanghai". That object has a handful of properties and values, i.e. "key/value" pairs ("population" being a key, and 14.35e6 being its value).
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
To cycle through this object (which is just a collection of key/value pairs, i.e. the equivalent of an "associative array"), we use the handy for...in
loop, in which we declare a variable to temporarily store each property as we iterate through them, as well as the object (array) that we wish to run through:
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for( var prop in shanghai ) {
// do something!
}
That loop is going to retrieve each property name in the shanghai object (array) and return it to us to do something with. Firstly, we are instructed to log each property to the console. To do that, we simply log to the console whatever is held in the 'prop' variable at the time, as follows:
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for( var prop in shanghai ) {
// log each property name to the console
console.log( prop );
}
// copy and paste this into your console and run it! It will log: "population, longitude, latitude, country"
Next, we are told to take it a step further, and retrieve the values associated with those properties (or "keys") and log those to the console, as well. This we'll do just like we would do in a regular for
loop, which you may recall is done by taking our variable and referring to individual indexes in our object (array) with it. For example, if you had the following array:
var temperatures = [ 100, 85, 73, 64, 55 ];
And wanted to log each of those values to the console, you would use a regular ol' for
loop as follows:
var temperatures = [ 100, 85, 73, 64, 55 ];
for ( var i = 0; i < temperatures.length; i++ ) {
console.log( temperatures[ i ] );
}
// copy and paste this into your console and run it! it will log: "100, 85, 73, 64, 55"
As you may know!
So, we can do the same type of thing inside our for...in
loop! Rather than naming our variable "i" as we did in the regular for loop (and frequently do, though it can be named anything!), we have our variable named "prop", but we're going to use it the same way (which is to reference the index that we are iterating over in our object at the time, and thus retrieve the value at said index):
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for( var prop in shanghai ) {
// log each key and value pair to the console, using the key name (stored in the "prop" variable) to refer to the proper index
// within the shanghai array
console.log( prop + ": " + shanghai[ prop ] );
}
// on the first iteration, the value of "prop" is "population", so the computer sees this as though it said:
// console.log( 'population' + ": " + shanghai[ 'population' ] );
By doing this, we are logging each property name and its associated value to the console as we iterate over the shanghai object.
It's just like accessing the value of an object (array) using bracket (or even dot) notation. If you wanted to just retrieve the value stored in the "country" property of the shanghai object, you could do so as follows:
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
console.log( shanghai[ 'country' ] );
// copy and paste this into your console and run it! it will log: "CHN"
As you may be familiar with, doing the above (passing the name of a given property) into the brackets like that will retrieve the value of that property. And if you deconstruct what is happening in a for...in
loop, you'll realize that our "prop" variable is equal to an individual property name each time it iterates through the shanghai object. So, on the first pass, "prop" is really equal to "population", so if we said console.log( shanghai[ prop ] );
, it would really be like saying console.log( shanghai[ 'population' ] );
, which would get us whatever value was stored in that "population" variable!
Hopefully this breakdown has served to help clarify some things, but let me know if there is still confusion!
Erik