Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial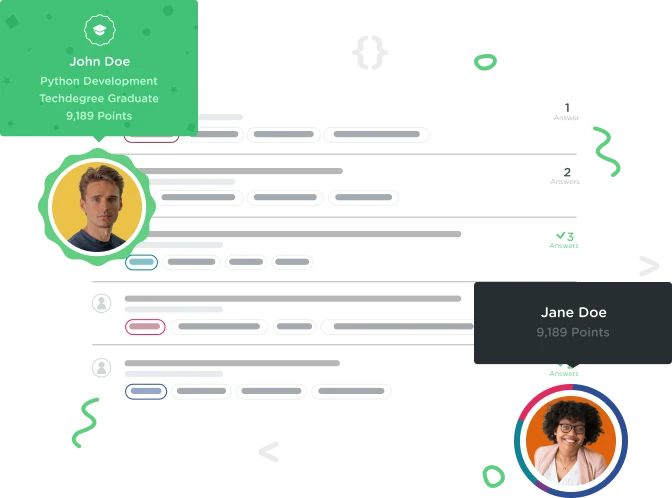
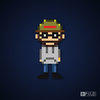
Benjamin Huffcut
7,294 PointsLooking for feedback...
I believe I got everything to run correctly, it just feels like I have a lot of extra steps. Any feedback would be helpful!
/*********************
This is the Quiz Game
**********************/
document.write('<h2>Are you ready to play the Ultimate Quiz Challenge?</h2>');
var totalRight = 0;
//Questions
var guess1 = prompt('How many actors have played James Bond?');
if (parseInt(guess1) === 8 ) {
document.write('<p>Good Job!</p>');
totalRight += 1;
} else {
document.write('<p>Nope, but nice try!</p>');
}
var guess2 = prompt('What is Captain America\'s real name?');
if (guess2.toUpperCase() == "STEVEN ROGERS" || guess2.toUpperCase() == "STEVE ROGERS") {
document.write('<p>Good Job!</p>');
totalRight += 1;
} else {
document.write('<p>Nope, but nice try!</p>');
}
var guess3 = prompt('What is Michaelangelo\'s catchprhase?');
if (guess3.toUpperCase() === "COWABUNGA" ) {
document.write('<p>Good Job!</p>');
totalRight += 1;
} else {
document.write('<p>Nope, but nice try!</p>');
}
var guess4 = prompt('Who directed the first Batman movie?');
if (guess4.toUpperCase() === "TIM BURTON" ) {
document.write('<p>Good Job!</p>');
totalRight += 1;
} else {
document.write('<p>Nope, but nice try!</p>');
}
var guess5 = prompt('Who is Slade Wilson?');
if (guess5.toUpperCase() === "DEATHSTROKE" ) {
document.write('<p>Good Job!</p>');
totalRight += 1;
} else {
document.write('<p>Nope, but nice try!</p>');
}
//Game Score
document.write('<h2>Congratulations, you answered ' + totalRight + ' questions correctly!</h2>');
//Reward
if (totalRight === 5) {
document.write('<h2>Yay! You get a gold crown!</h2>');
} else if (totalRight == 4 || totalRight == 3) {
document.write('<h2>Woo! You get a silver crown!</h2>');
} else if (totalRight == 2 || totalRight == 1) {
document.write('<h2>Keep Trying! You get a bronze crown!</h2>');
} else if (totalRight === 0) {
document.write('<h2>Better luck next time!</h2>');
}
3 Answers
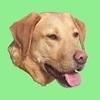
alastair cooper
30,617 PointsHi,
Your logic is impeccable, but it uses a lot of repeated code.
Consider making 1 function for testing the answer that takes two arguments (which question you want to answer and the answer given). the function could contain an array of answers which you could test against.
something like this
function testAnswer( whichQuestion, guess ){
var correctAnswers = [ 'ans1' , 'ans2' , 'ans3' , 'ans4', 'ans5' ]
if ( guess === correctAnswers[whichQuestion] ) {
// do whatever you do if correct
} else {
// do whatever you do if incorrect
}
}
If ever you find yourself repeating code (usually subconsciously indicated by going for the copy and paste buttons), it is worth stopping and considering if the code would be better inside a reuseable function.
Keep up the learning. Isn't JavaScript cool.
Cheers
Alastair
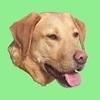
alastair cooper
30,617 PointsBetter yet, the function could return true or false inside the conditional statement so that the value can be used by the code calling the function.
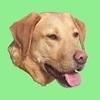
alastair cooper
30,617 Points1 more thing. If you want to store the answers given then you could put them in an array. But you don't use the answers after you have tested them so you don't need to create 5 separate guess variables. Just overwrite the first one. i.e. just declare var guess; at the beginning and you can overwrite it every time they answer a new question by just calling guess = prompt(...).
By doing this, you enable the placing of the whole game inside a for loop that runs 5 times, asks a question(from an array you declare beforehand), sets the value of 'guess'. Tests it with the function above. If true, increment points, etc. Then go through the whole loop again 4 more times.
This way, you could shorten your code to 2 variable declarations, 1 for loop and 2 functions (testAnswer and getScoreMessage).
Cheers
I'll stop interrupting you now.
Alastair
Benjamin Huffcut
7,294 PointsBenjamin Huffcut
7,294 PointsThanks Alastair!
I didn't learn how to use functions yet, but I definitely see how they are useful from your example!